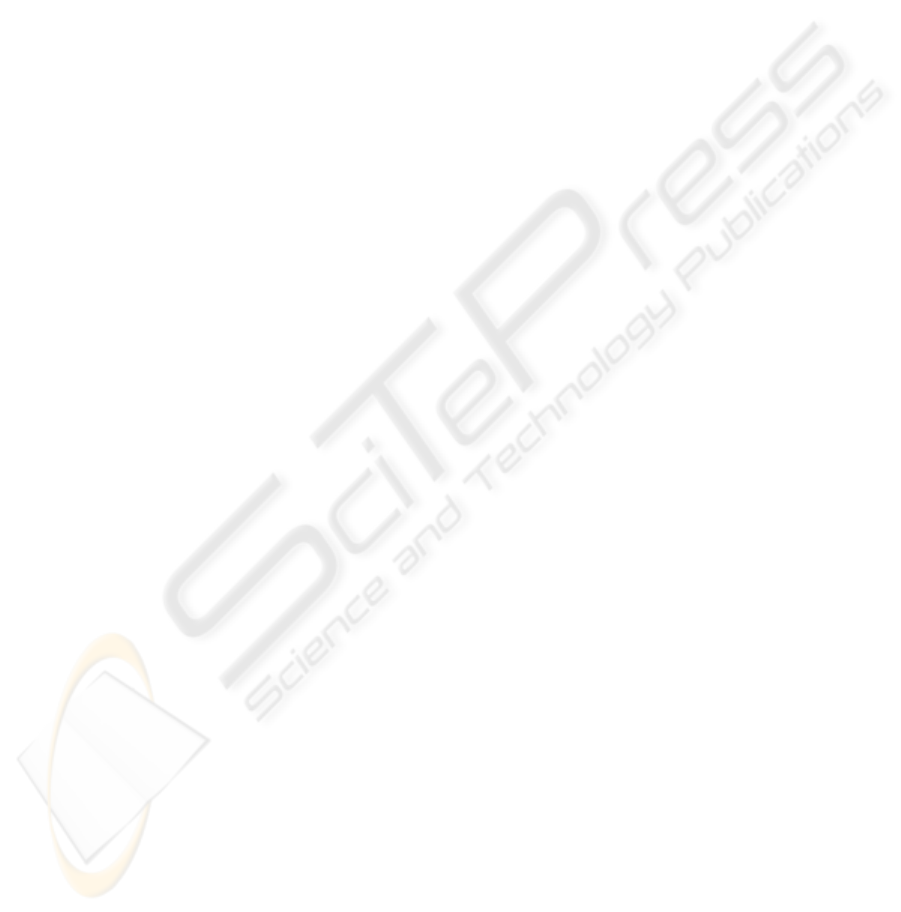
are promising, end-user experience from a variety of
applications still needs to be obtained and studied, to
find out whether the presented approach is practical
for analyzing memory consumption. Analyzing the
memory and run-time overhead is feasible, as we have
demonstrated above. Analyzing the value of our tools
to developers is more difficult; based on past publica-
tions in this field, we expect that any report on utility
and viability of this approach will remain anecdotal.
We envision two application areas for this ap-
proach: development and operations. Our applica-
tions of the tool had primarily been in the field of de-
velopment – helping the developer to find out mem-
ory leaks in the application, so that the code can be
improved.
In operations, the application of the approach
would be different. For example, the operator might
apply memory accounting to different services run-
ning in the same service container, and then take ser-
vice management decisions based on the amount of
memory used by each service (e.g. to migrate a ser-
vice with high memory consumption to a different
machine). As another example, the approach could
be used for the self-policing of application containers:
the container could enforce an upper limit on memory
consumption, and let allocations from a principal fail
if the principal’s memory account is overdrawn.
Further implementation details can be found
in (Bouch´e, 2007). The profiler is available at
sourceforge.net
.
REFERENCES
Ammons, G., Ball, T., and Larus, J. R. (1997). Exploiting
hardware performance counters with flow and con-
text sensitive profiling. In PLDI ’97: Proceedings
of the ACM SIGPLAN 1997 conference on Program-
ming language design and implementation, pages 85–
96, New York, NY, USA. ACM Press.
Arnold, M. and Ryder, B. G. (2001). A framework for re-
ducing the cost of instrumented code. In SIGPLAN
Conference on Programming Language Design and
Implementation, pages 168–179.
Binder, W. and Hulaas, J. (2006). Exact and portable profil-
ing for the JVM using bytecode instruction counting.
volume 164, pages 45–64.
Binder, W., Hulaas, J. G., and Villazon, A. (2001). Portable
resource control in java. In Proceedings of the
16th ACM SIGPLAN conference on Object oriented
programming, systems, languages, and applications,
pages 139–155. ACM Press.
Blackburn, S. M., Garner, R., and Hoffmann, C. (2006).
The DaCapo benchmarks: java benchmarking devel-
opment and analysis. In OOPSLA ’06, pages 169–190,
New York, NY, USA. ACM.
Bouch´e, P. (2007). A comparative study of J2EE profil-
ing approaches for usage within asg. Master’s the-
sis, Hasso-Plattner-Institute for IT-Systems Engineer-
ing of the University of Potsdam.
Brear, D. J., Weise, T., Wiffen, T., Yeung, K. C., Bennett, S.
A. M., and Kelly, P. H. J. (2003). Search strategies for
java bottleneck location by dynamic instrumentation.
In Software, IEE Proceedings, volume 150, Issue: 4,
pages 235– 241.
Carrera, D., Guitart, J., Torres, J., Ayguade, E., and Labarta,
J. (2003). Complete instrumentation requirements
for performance analysis of web based technologies.
In Performance Analysis of Systems and Software,
2003. ISPASS. 2003 IEEE International Symposium
on, pages 166– 175.
Cooper, B., Lee, H., and Zorn, B. (1998). Profbuilder: A
package for rapidly building java execution profilers.
Dmitriev, M. (2003). Design of JFluid: A profiling technol-
ogy and tool based on dynamic bytecode instrumenta-
tion. Technical report, Sun Microsystems Inc.
Factor, M., Schuster, A., and Shagin, K. (2004). Instru-
mentation of standard libraries in object-oriented lan-
guages: the twin class hierarchy approach. In OOP-
SLA ’04: Proceedings of the 19th annual ACM SIG-
PLAN conference on Object-oriented programming,
systems, languages, and applications, pages 288–300,
New York, NY, USA. ACM Press.
Goldberg, A. and Havelund, K. (2003). Instrumentation of
java bytecode for runtime analysis.
Harkema, M., Quartel, D., van der Mei, R., and Gijsen,
B. (2003). JPMT: A java performance monitoring
tool. Technical Report TR-CTIT-03-25 Centre for
Telematics and Information Technology, University of
Twente, Enschede.
Kazi, I. H., Jose, D. P., Ben-Hamida, B., Hescott, C. J.,
Kwok, C., Konstan, J., Lilja, D. J., and Yew, P.-C.
(2000). JaViz: A client/server java profiling tool.
Kiczales, G., Hilsdale, E., Hugunin, J., Kersten, M., Palm,
J., and Griswold, W. G. (2001). An overview of As-
pectJ. In ECOOP ’01: Proceedings of the 15th Eu-
ropean Conference on Object-Oriented Programming,
pages 327–353, London, UK. Springer-Verlag.
Pauw, W. D., Jensen, E., and Konuru, R. (1999). Jinsight, a
visual tool for optimizing and understanding java pro-
grams. ibm corporation, research division.
http://
www.alphaWorks.ibm.com/tech/jinsight/
. Url
last visited: 23. May 2009.
Pearce, D. J., Webster, M., Berry, R., and Kelly, P. H. J.
(2006). Profiling with aspectj. In Software: Practice
and Experience. John Wiley & Sons, Ltd.
Seragiotto, C. and Fahringer, T. (2005). Analysis of dis-
tributed java applications using dynamic instrumen-
tation. In IEEE International Conference on Cluster
Computing (Cluster 2005).
Sevitsky, G., de Pauw, W., and Konuru, R. (2001). An
information exploration tool for performance analy-
sis of java programs. In TOOLS ’01: Proceedings
of the Technology of Object-Oriented Languages and
Systems, page 85, Washington, DC, USA. IEEE Com-
puter Society.
AGGREGATED ACCOUNTING OF MEMORY USAGE IN JAVA
185