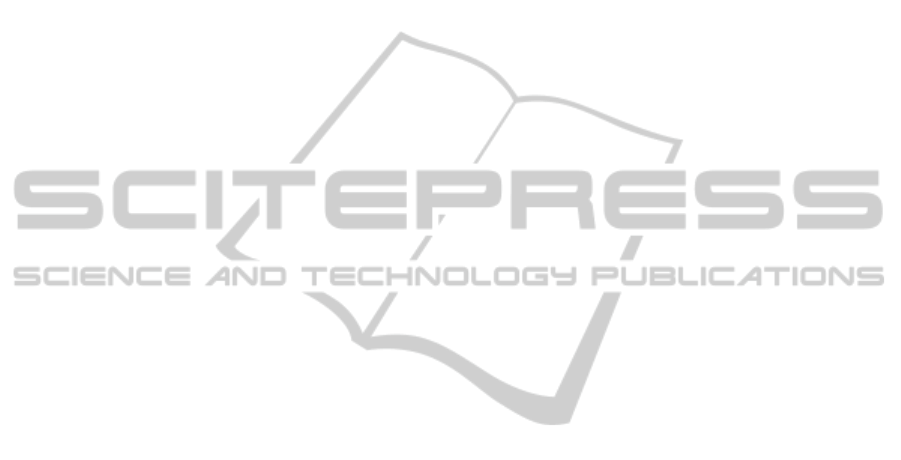
abled by default, which can be provided by the type-
assotiation, but later overriden for the particular ob-
ject by assotiation of the corresponded AD with this.
Therefore, the priority of the AD should be defined:
for example, object assotiation is almost always more
important that the type assotiation.
Our implementation of AD which guarantees cor-
rectness of usage in compilation time. Consider the
following example of AD settings:
employee.Set(e=>e.Position,
AD.Caption,"text")}
Here the address is specified by LINQ Expression,
and it is impossible to assotiate data with a non-
existing field. The name of AD is provided via single-
ton object
AD.Caption
, which also defines the type of
the stored value. The type of the third
"text"
argu-
ment is defined by
AD.Caption
, and therefore guar-
antees the type correctess of the value that is being as-
sociated. This effect is reached by the extensiveuse of
.NET generic-methods and LINQ expressions. Type-
associated data can also be defined in attributes, and
such definitions are placed into hashtable with other
AD at the first time the type is used.
AD.Caption
also defines the logic of AD aggregation.
Summarizing, the introduced metadata system is:
1. Flexible. It allows storing actions in metadata,
which is vital to the procedure of metadata-driven
validation. It also allows changing metadata in
runtime, and defining context-dependent meta-
data. All these features are new in comparison
with traditional .NET metadata.
2. Type-safe, and discloses more errors in the com-
pilation time in comparison with storing metadata
value in hash-tables.
3. Expandable, because the addition of new AD re-
quires only the definition of a singleton object,
similar to
AD.Caption
.
3 DATA SERVER
This section describes the development of a data-
driven application in the Thornado framework. The
most important part of the application is a model,
which represents data. We demand a firm correspon-
dence between a hierarchy of objects in model, and
hierarchy of controls in a page. That means we must
provide a data structures for
MainWindow
and other
controls. Such structures play the role of a view
model in MVVM pattern. For example,
MainWindow
is a small class with one property
Content
, which
represents a top-level control in a window. To display
data in
MainWindow
, the
Content
property should
be assigned to some object, and so various methods
like
ShowEmployee
or
ShowDepartments
are defined
in
MainWindow
inheritant and are bound with it by
AD. Other auxiliary classes, like
StackPanel
,
Grid
and
MasterDetailTemplate
, are also provided by
the Thornado framework. It is important that these
classes are data structures, are not widgets, and do not
haveany references to windows, HTML code builders
or other platform-specific code.
The data structures can be displayed in the
MainWindow
simply by setting
Content
property to,
for example,
Employee
or
List<Employee>
object.
The way how data structures are displayed are defined
in various ADs, including
Caption
and
Enabled
.
The data server (DS) provides the interaction between
data structures and the user, by acting like a controller
and creating views with the aid of the associated data.
Data server follows the algorithm, shown in the
Figure 2. At the first call DS goes through the tree
of objects in the model, gathers all the additional
data and prepares a tree-like
NEW
reply with a de-
tailed description of each node in the GUI. The re-
ply is passed to the Client, which actually builds and
processes graphic user interface. Depending on the
client’s type, it may create WPF widgets and sub-
scribe to their events, or send an HTML page and then
reroute Ajax requests from the page to the data server
in a proper format.
The most important requests are
SET
and and
PUSH
. The
SET
request contains an address of a field
and a new value. The data server processes the re-
quest by settings the field to the received value, and
calling the notifications delegates associated with the
field, as well as validation prodecures that may be de-
fined in the model. The
PUSH
request contains the ad-
dress of an element and a name of the button, which
was associated with the element. After receiving a
PUSH
request, the DS invokes the corresponding dele-
gate from an object at the specified address. Methods,
called by the data server, alter the model and AD ac-
cording to the business logic. After the methods are
completed, DS creates a
UPD
reply, which contains the
updated values for changed nodes.
If the invoked method requires a dialog window or
a message box to be shown, it calls the corresponding
method from the data server. In this case, the server
forms a
NEW
reply that corresponds to the new window
and processes events of the window. Execution of the
old window’s methods are suppressed, and the control
will return to them when the dialog box is closed.
AnApproachtotheMetadataDrivenProgrammingin.NetFramework
241