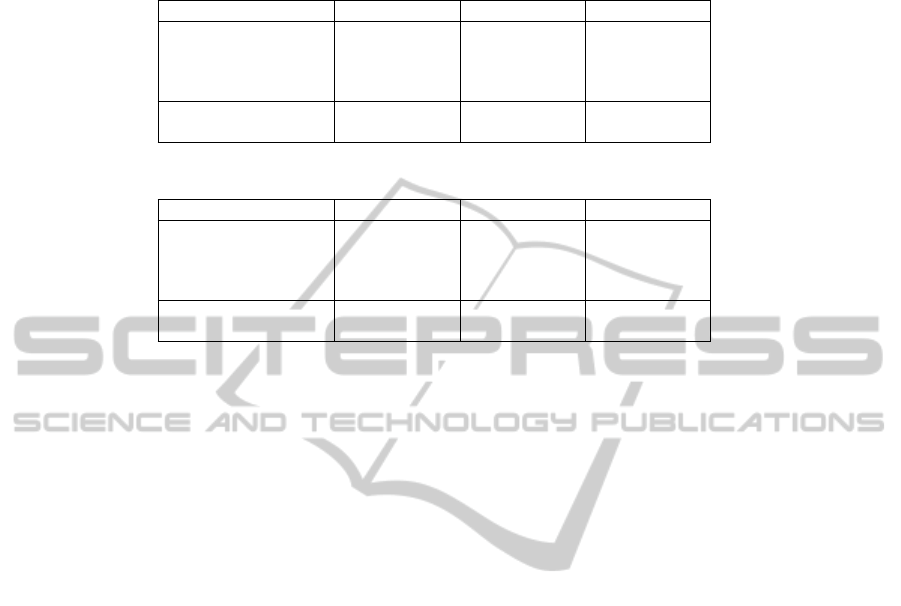
Table 1: Performance comparison for several chains, in asynchronous and synchronous modes, in microseconds per transmis-
sion.
Synchronouse mode
Small data Medium data Big data
FOR 0, 024 ± 0, 001 0, 017 ± 0 0, 017 ± 0
Radiant Chains 0, 031 ± 0, 001 0, 024 ± 0 0, 023 ± 0, 001
Liberty Chains 0, 335 ± 0, 006 0, 325 ± 0, 005 0, 323 ± 0, 006
CCR (in sync mode) 5, 687 ± 0, 518 5, 194 ± 0, 648 5, 169 ± 0, 579
Prime Sources 0, 642 ± 0, 022 0, 498 ± 0, 009 0, 491 ± 0, 012
CCR (in async mode) 1, 254 ± 0, 025 0, 949 ± 0, 02 0, 913 ± 0, 019
Asynchronouse mode
Small data Medium data Big data
FOR 0, 018 ± 0 0, 006 ± 0 0, 005 ± 0
Radiant Chains 0, 018 ± 0 0, 007 ± 0 0, 007 ± 0
Liberty Chains 0, 184 ± 0, 008 0, 131 ± 0, 006 0, 125 ± 0, 004
CCR (in sync mode) 8, 654 ± 0, 493 8, 379 ± 0, 47 8, 402 ± 0, 405
Prime Sources 0, 954 ± 0, 046 0, 743 ± 0, 026 0, 726 ± 0, 032
CCR (in async mode) 0, 918 ± 0, 018 0, 812 ± 0, 025 0, 783 ± 0, 018
4 PERFORMANCE OF PRIME
In this section, we describe the computational exper-
iment that certifies the effectiveness of Prime. Our
main objective was to test the approach rather than
implementation, and so we choose to compare Liberty
and Radiant Prime to Concurrency and Coordination
Runtime (CCR), a Microsoft .NET solution to mes-
saging in service-oriented robotics system. We did
not considered Decentralized Software Services tech-
nologies, which is built upon CCR and is a true core of
Microsoft Robotics Studio, because DSS adds over-
heads of interprocess interaction that Liberty Prime
does not have. We have not considered Optimus
Prime, because the majority of overheads are intro-
duced by Redis in this case, and testing Redis per-
formance is not a theme of our article; in addition,
we do not declare that Redis is the best solution for
interprocess communication, and Prime architecture
allows us replacing it with another technology easily.
We have also not tested ROS, because it runs on dif-
ferent framework and on different operating system,
so it would be unclear, whether overheads are brought
by communication approach or by framework; how-
ever, based on (Hongslo, 2012), we assume ROS per-
formance is inferior to the CCR.
For experiment, we used a topology that consists
of several chains of services. Each service performs
an addition function, and therefore consumes a neg-
ligible time. The data that flow through the services,
and can be small (100 bytes), medium (1 kilobyte) or
big (10 kilobytes). There are two modes of how each
chain processes signals:
• Asynchronous mode, when data flow through the
chain constantly. The common example is pro-
cessing data from sensors. It is the native behav-
ior of CCR. In Prime, it is represented as ISource
with the subsequently connected IChain (let us
call this topology Prime Source for shortness).
• Synchronous mode, when the new input signal ap-
pears only when the output signal is produced for
the previous input, most commonly, as a response
to it. Synchronous chains are the most common
element of our control systems. The systems are
mostly synchronous; however, they sometimes re-
quire the collection of processed asynchronous
data. Prime Sources and CCR may operate in this
mode, if they are augmented with a dispatcher that
releases an input signal when the output is com-
puted. The presumed implementation of this pat-
tern in Prime is linked chains, which have differ-
ent implementation in Radiant and Liberty Prime.
For comparison, we also consider the time, re-
quried to achieve the same task without any services,
by calling the methods directly inside for cycle. This
“system” is represented in results with the name FOR.
At the first series of our experiment, we directly
measured overheads in Prime Sources, Prime Chains
and CCR. Two later systems were forced to work in
synchronous mode by the dispatcher. We measured
the total time of processing several signals, and ob-
tained the overheads time per one transmission, which
is shown in Table 1 for various package sizes.
The time depends on packages sizes only slightly,
because neither Prime not CCR does not serialize or
deserialize them for local systems. We can see that
ICINCO2014-11thInternationalConferenceonInformaticsinControl,AutomationandRobotics
174