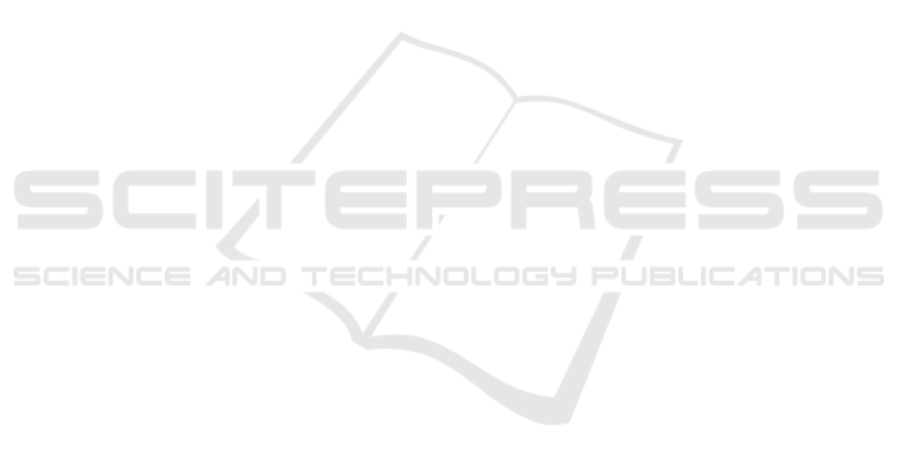
7 CONCLUSIONS
In this paper, we showed how Umple provides major
features required for concurrent programming. The
focus was on showing how active object development
in Umple will be simplified such that a user will not
need to worry about all of its challenges and
implementation details.
Concurrent programming in Umple is supported
at the model level meaning that concurrency will be
consequently enforced at the generated code level.
We showed how concurrency definition and
implementation in Umple could easily help a
developer to optimize performance of their
applications
Using simple Umple constructs, a user is able to
define their time constraints. We evaluated our work
on two bases, qualitative and quantitative. In the
qualitative evaluation, we showed a comparison
between standards (UML and MARTE) used for time
management, and Umple. The essence behind our
comparison was to show how Umple can meet time
requirements specified in these common standards.
For quantitative evaluation, we showed a comparison,
based on LOC and cyclomatic complexity, between
Umple models and their generated code in C++,
based on which we showed significant statistical
difference.
For future work, we will highlight the
communication among active objects in a distributed
environment. This requires the implementation of
concepts such as ports and composite structure.
REFERENCES
Alghamdi, A. (2010). Queued and Pooled Semantics for
State Machines in the Umple Model-Oriented
Programming Language (Master’s thesis). University
of Ottawa.
Badreddin, O., Forward, A., and Lethbridge, T. C. (2014).
Improving Code Generation for Associations:
Enforcing Multiplicity Constraints and Ensuring
Referential Integrity. SERA (selected papers) (Vol.
430). https://doi.org/10.1007/978-3-642-30460-6
Badreddin, O., Lethbridge, T. C., and Forward, A. (2014).
A Test-Driven Approach for Developing Software
Languages. In MODELSWARD 2014, International
Conference on Model-Driven Engineering and
Software Development (pp. 225–234). SCITEPRESS -
Science and and Technology Publications.
https://doi.org/10.5220/0004699502250234
Cplusplus.com. (2016). Future. Retrieved June 20, 2001,
from http://www.cplusplus.com/reference/future/
Espinoza, H., Gérard, S., Lönn, H., and Kolagari, R. T.
(2009). Harmonizing MARTE, EAST-ADL2, and
AUTOSAR to Improve the Modelling of Automotive
Systems. In The workshop standard, AUTOSAR.
Fuks, O., Ostroff, J. S., and Paige, R. F. (2004). SECG: The
SCOOP-to-Eiffel code generator. Journal of Object
Technology, 3, 143–160. https://doi.org/10.5381/
jot.2004.3.10.a3
Gherbi, A., and Khendek, F. (2006). UML Profiles for
Real-Time Systems and their Applications. Journal of
Object Technology, 5(4), 149–169.
Kaneiwa, K., and Satoh, K. (2010). On the complexities of
consistency checking for restricted UML class
diagrams. Theoretical Computer Science, 411(2), 301–
323. https://doi.org/10.1016/j.tcs.2009.04.030
Klein, P. N., Lu, H. I., and Netzer, R. H. B. (2003).
Detecting race conditions in parallel programs that use
semaphores. Algorithmica (New York), 35(4), 321–345.
https://doi.org/10.1007/s00453-002-1004-3
Lavender, R. G., and Schmidt, D. C. (1996). Active object:
an object behavioral pattern for concurrent program-
ming. In Pattern languages of program design 2 (pp.
483–499). Addison-Wesley Longman Publishing Co.,
Inc. Boston, MA, USA.
Lethbridge, T. C., Abdelzad, V., Husseini Orabi, M.,
Husseini Orabi, A., and Adesina, O. (2016). Merging
Modeling and Programming Using Umple. In
International Symposium on Leveraging Applications
of Formal Methods, ISoLA 2016 (pp. 187–197).
https://doi.org/10.1007/978-3-319-47169-3_14
Mallet, F. (2008). Clock constraint specification language:
Specifying clock constraints with UML/MARTE.
In Innovations in Systems and Software Engineering
(Vol. 4, pp. 309–314). https://doi.org/10.1007/s11334-
008-0055-2
Meyer, B. (1993). Systematic concurrent object-oriented
programming. Communications of the ACM.
https://doi.org/10.1145/162685.162705
Microsoft.com. (2015). Delegates (C# Programming
Guide). Retrieved January 1, 2017, from https://msdn.
microsoft.com/en-CA/library/ms173171.aspx
Ober, I., and Stan, I. (1999). On the Concurrent Object
Model of UML*. In 5th International Euro-Par
Conference Toulouse, France, August 31 – September
3, 1999 Proceedings (pp. 1377–1384). https://doi.org/
10.1007/3-540-48311-X_193
OMG. (2011a). UML 2.4.1. Retrieved January 1, 2015,
from http://www.omg.org/spec/UML/2.4.1/
OMG. (2011b). UML Profile for MARTE: Modeling and
Analysis of Real-Time Embedded Systems. Retrieved
from http://www.omg.org/spec/MARTE/1.1/PDF
Orabi, M. H. (2017). Facilitating the Representation of
Composite Structure, Active objects, Code Generation,
and Software Component Descriptions for AUTOSAR
in the Umple Model-Oriented Programming Language
(PhD Thesis). University of Ottawa. https://doi.org/
10.20381/ruor-20732
Orabi, M. H., Orabi, A. H., and Lethbridge, T. (2016).
Umple as a Component-based Language for the
Development of Real-time and Embedded
Applications. In Proceedings of the 4th International
Conference on Model-Driven Engineering and
MODELSWARD 2018 - 6th International Conference on Model-Driven Engineering and Software Development
584