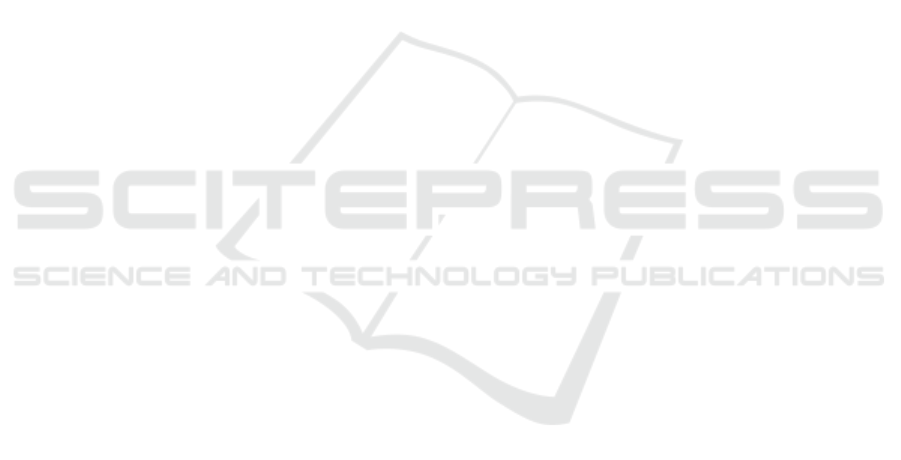
REFERENCES
Arcelli, D., Berardinelli, L., and Trubiani, C. (2015). Per-
formance antipattern detection through fuml model li-
brary. In Proceedings of the 2015 Workshop on Chal-
lenges in Performance Methods for Software Develop-
ment, pages 23–28. ACM.
Arcelli, D., Cortellessa, V., and Trubiani, C. (2012). Anti-
pattern-based model refactoring for software perfor-
mance improvement. In Quality of Software Architec-
tures, pages 33–42. ACM.
Bernardi, M. L., Cimitile, M., and Di Lucca, G. A. (2013).
A model-driven graph-matching approach for design
pattern detection. In Working Conf. on Reverse Engi-
neering, pages 172–181. IEEE.
Burn, O. (2017). Checkstyle. http://checkstyle.
sourceforge.net/index.html.
Copeland, T. and Le Vourch, X. (2017). PMD. https://pmd.
github.io/.
Cortellessa, V., Martens, A., Reussner, R., and Trubiani, C.
(2010). A process to effectively identify guilty per-
formance antipatterns. In International Conference
on Fundamental Approaches to Software Engineer-
ing, pages 368–382. Springer.
Djoudi, L., Barthou, D., Carribault, P., Lemuet, C., Acqua-
viva, J.-T., and Jalby, W. (2005). Exploring applica-
tion performance: a new tool for a static/dynamic ap-
proach. In Proceedings of the 6th LACSI Symposium.
Los Alamos Computer Science Institute.
Dynatrace (2017). Dynatrace appmon. https://
www.dynatrace.com/.
Fontana, F. A. and Zanoni, M. (2017). Code smell seve-
rity classification using machine learning techniques.
Knowledge-Based Systems, 128:43–58.
Foundation, A. S. (2017). Apache tomcat. http://tomcat.
apache.org/.
Fowler, M. and Beck, K. (1999). Refactoring: improving
the design of existing code. Addison-Wesley Professi-
onal.
Gamma, E., Helm, R., Johnson, R., and Vlissides, J.
(1995). Design Patterns: Elements of Reusable
Object-oriented Software. Addison-Wesley Longman
Publishing Co., Inc., Boston, MA, USA.
Goetz, B. (2005). Anatomy of a flawed microbenchmark.
https://www.ibm.com/developerworks/java/library/
j-jtp02225/.
Grabner, A. (2009). Performance analysis: How to
identify synchronization issues under load? https://
www.dynatrace.com/blog/performance-analysis-
how-to-identify-synchronization-issues-under-load/.
Herber, P., Fellmuth, J., and Glesner, S. (2008). Mo-
del Checking SystemC Designs Using Timed Auto-
mata. In International Conference on Hardware/Soft-
ware Codesign and Integrated System Synthesis (CO-
DES+ISSS), pages 131–136. ACM press.
Herber, P., Pockrandt, M., and Glesner, S. (2015). STATE
– A SystemC to Timed Automata Transformation En-
gine. In International Conferen on Embedded Soft-
ware and Systems (ICESS), pages 1074–1077. IEEE.
Heuzeroth, D., Holl, T., Hogstrom, G., and Lowe, W.
(2003). Automatic design pattern detection. In Pro-
gram Comprehension, 2003. 11th IEEE International
Workshop on, pages 94–103. IEEE.
Long, J. (2001). Software reuse antipatterns. ACM SIGS-
OFT Software Engineering Notes, 26(4):68–76.
Louridas, P. (2006). Static code analysis. IEEE Software,
23(4):58–61.
Luo, Q., Nair, A., Grechanik, M., and Poshyvanyk, D.
(2017). Forepost: Finding performance problems au-
tomatically with feedback-directed learning software
testing. Empirical Software Engineering, 22(1):6–56.
Owen, K. (2016). Improve the smell of your code with mi-
crorefactorings. https://www.sitepoint.com/improve-
the-smell-of-your-code-with-microrefactorings/.
Pugh, B. and Hovemeyer, D. (2017). Findbugs. http://
findbugs.sourceforge.net/.
Rasool, G. and Streitfdert, D. (2011). A survey on design
pattern recovery techniques. IJCSI International Jour-
nal of Computer Science Issues, 8(2):251–260.
Smith, C. U. and Williams, L. G. (2002). New software per-
formance antipatterns: More ways to shoot yourself in
the foot. In Int. CMG Conf., pages 667–674.
Tsantalis, N., Chatzigeorgiou, A., Stephanides, G., and Hal-
kidis, S. T. (2006). Design pattern detection using si-
milarity scoring. IEEE transactions on software engi-
neering, 32(11).
Washizaki, H., Fukaya, K., Kubo, A., and Fukazawa, Y.
(2009). Detecting design patterns using source code
of before applying design patterns. In Intl. Conf. on
Computer and Information Science, pages 933–938.
IEEE.
Wendehals, L. (2003). Improving design pattern instance
recognition by dynamic analysis. In Proc. of the ICSE
2003 Workshop on Dynamic Analysis (WODA), Port-
land, USA, pages 29–32.
Wierda, A., Dortmans, E., and Somers, L. J. (2007). De-
tecting patterns in object-oriented source code - a case
study. In ICSOFT (SE), pages 13–24. INSTICC Press.
Woodside, M., Franks, G., and Petriu, D. C. (2007). The
future of software performance engineering. In Future
of Software Engineering, 2007. FOSE’07, pages 171–
187. IEEE.
ICSOFT 2018 - 13th International Conference on Software Technologies
44