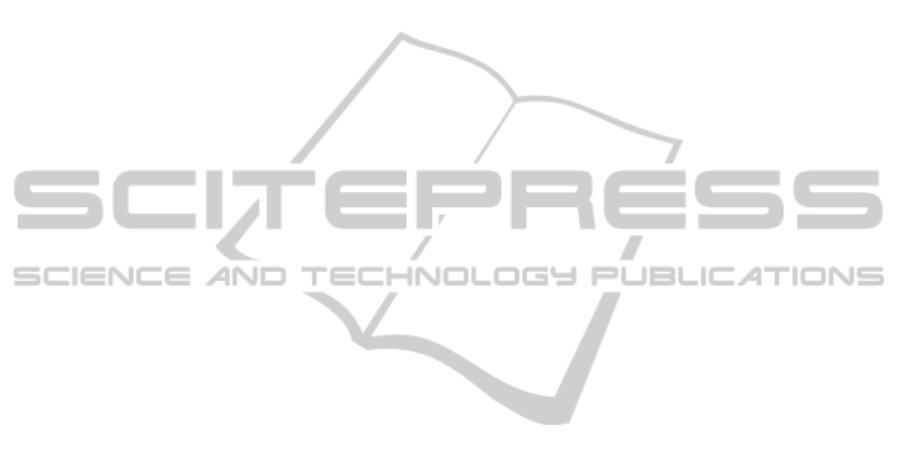
work will also address trying to find the globally vol-
ume maximizing path among all paths from the start
to the goal configuration. In addition, we took only
strictly better improvements to the volume of the ob-
ject in Step 10 in Algorithm 2 and Step 6 in Algorithm
1. A further avenue for investigation could be to re-
place this with a simulated annealing style optimiza-
tion to allow non-optimal adjustments so that one has
the possibility of escaping local maxima.
ACKNOWLEDGEMENTS
This work was carried out at the Wingquist Labora-
tory VINN Excellence Centre, and is part of the Sus-
tainable Production Initiative and the Production Area
of Advance at Chalmers University of Technology. It
was supported by the Swedish Governmental Agency
for Innovation Systems.
REFERENCES
Bj
¨
orkenstam, S., Segeborn, J., Carlson, J. S., and Bohlin, R.
(2012). Assembly verification and geometry design
by distance field based shrinking. In 4th CIRP Con-
ference on Assembly Technology and Systems-CATS
2012, University of Michigan, Ann Arbor, USA on
May 21-23, 2012.
Bohlin, R. and Kavraki, L. (2000). Path planning using lazy
prm. In IEEE International Conference on Robotics
and Automation, volume 1, pages 521–528. IEEE.
Carlson, J. S., Spensieri, D., S
¨
oderberg, R., Bohlin, R., and
Lindkvist, L. (2013). Non-nominal path planning for
robust robotic assembly. Journal of manufacturing
systems, 32(3):429–435.
Cohen-Or, D. and Kaufman, A. (1995). Fundamentals of
surface voxelization. Graphical models and image
processing, 57(6):453–461.
COIN-OR (2014). COmputational INfrastructure for Oper-
ations Research. http://www.coin-or.org/.
Folkestad, J. E. and Johnson, R. L. (2002). Integrated rapid
prototyping and rapid tooling (irprt). Integrated Man-
ufacturing Systems, 13(2):97–103.
Geraerts, R. and Overmars, M. H. (2005). On improv-
ing the clearance for robots in high-dimensional con-
figuration spaces. In Intelligent Robots and Sys-
tems, 2005.(IROS 2005). 2005 IEEE/RSJ Interna-
tional Conference on, pages 679–684. IEEE.
Geraerts, R. and Overmars, M. H. (2007). The corridor
map method: Real-time high-quality path planning.
In Robotics and Automation, 2007 IEEE International
Conference on, pages 1023–1028. IEEE.
Hermansson, T., Bohlin, R., Carlson, J. S., and S
¨
oderberg,
R. (2012). Automatic path planning for wiring harness
installations (wt). In 4th CIRP Conference on Assem-
bly Technology and Systems-CATS 2012, University of
Michigan, Ann Arbor, USA on May 21-23, 2012.
Ilies, H. T. and Shapiro, V. (1999). The dual of sweep.
Computer-Aided Design, 31(3):185–201.
Kim, J., Pearce, R. A., and Amato, N. M. (2003). Ex-
tracting optimal paths from roadmaps for motion plan-
ning. In Robotics and Automation, 2003. Proceed-
ings. ICRA’03. IEEE International Conference on,
volume 2, pages 2424–2429. IEEE.
Larsen, E., Gottschalk, S., Lin, M. C., and Manocha, D.
(1999). Fast proximity queries with swept sphere vol-
umes. Technical report, Technical Report TR99-018,
Department of Computer Science, University of North
Carolina.
LaValle, S. and Kuffner Jr, J. (2001). Randomized kin-
odynamic planning. The International Journal of
Robotics Research, 20(5):378–400.
N
¨
uchter, A., Elseberg, J., Schneider, P., and Paulus, D.
(2010). Linearization of rotations for globally con-
sistent n-scan matching. In IEEE International Con-
ference on Robotics and Automation (ICRA), page 7.
Shellshear, E., Tafuri, S., and Carlson, J. (2014). A multi-
threaded algorithm for computing the largest non-
colliding moving geometry. Computer-Aided Design,
49(0):1 – 7.
Spensieri, D., Bohlin, R., and Carlson, J. S. (2013). Coordi-
nation of robot paths for cycle time minimization. In
CASE, pages 522–527.
Spensieri, D., Carlson, J. S., Bohlin, R., and S
¨
oderberg, R.
(2008). Integrating assembly design, sequence opti-
mization, and advanced path planning. ASME Confer-
ence Proceedings, (43253):73–81.
Vanderhyde, J. and Szymczak, A. (2008). Topological sim-
plification of isosurfaces in volumetric data using oc-
trees. Graphical Models, 70(1):16–31.
Zachmann, G. et al. (2000). Virtual Reality in Assem-
bly Simulation: Collision Detection, Simulation Algo-
rithms, and Interaction Techniques. Fraunhofer-IRB-
Verlag.
Zheng, L., Cho, Y.-K., Liu, X., and Wang, W. (2011). Cvt-
based 2d motion planning with maximal clearance. In
Robotics and Automation (ICRA), 2011 IEEE Interna-
tional Conference on, pages 2281–2287. IEEE.
AHeuristicFrameworkforPathPlanningtheLargestVolumeObjectfromaStarttoGoalConfiguration
271