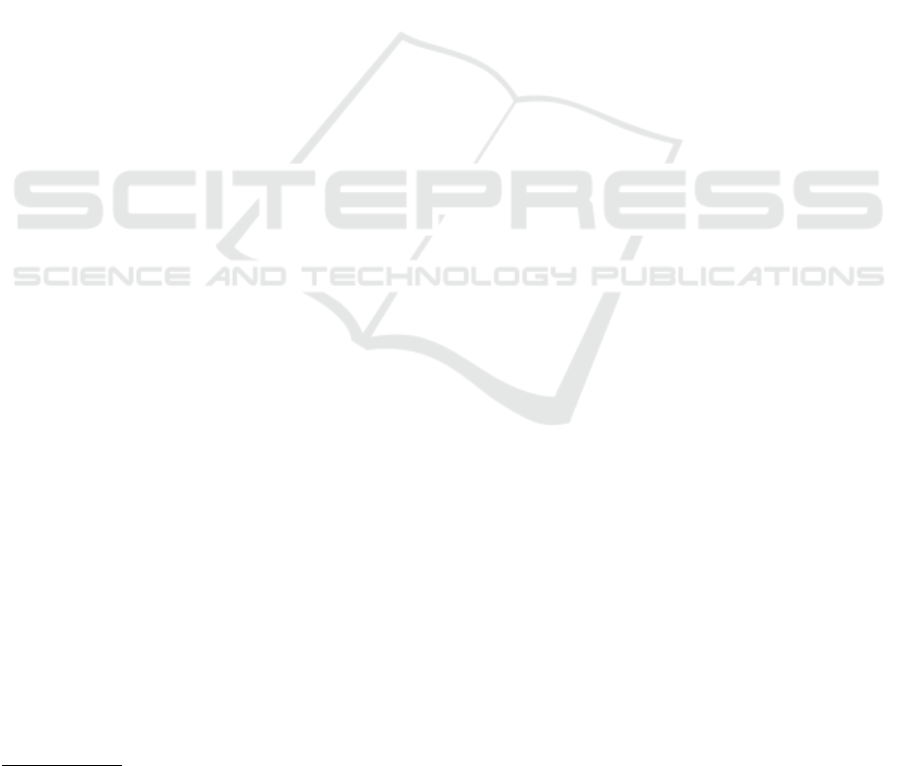
The shape of our test specifications, i.e., the sig-
nature of test, might remind us of test specifications
in the Javascript testing framework Jest.
15
Indeed,
we took some initial inspirations from Jest for spec-
ifying tests in JNRTEST. Of course, the rest of the
context (programming language, static typing vs. dy-
namic typing) does not allow for additional compar-
isons between JNRTEST and Jest.
As a testing framework, JNRTEST shares with
other testing frameworks in Java (JUnit and TestNG)
and other statically typed and dynamically typed lan-
guages (e.g., CUnit for C, Pytest for Python, SUnit
for Smalltalk, etc.), the same concepts of “test case”,
“test runner” and the ability to create a test fixture
16
for tests. Thus, JNRTEST should be easily usable for
developers who are already familiar with existing test-
ing frameworks, though its main context is the Java
language.
8 CONCLUSIONS
In this paper, we presented the prototype implemen-
tation of a novel Java testing framework without us-
ing reflection. Besides showing proof of concept that
we can avoid reflection with its drawbacks, our main
goals were to implement something simple to main-
tain, easy to use, and efficient. Although JNRTEST
is in its early development stage, it provides enough
features to be usable in practice, as shown in the pa-
per. Moreover, it can be extended and used with all
the existing Java testing libraries.
Currently, JNRTEST does not support nested tests
(a feature introduced in JUnit 5). We plan to investi-
gate how to implement them in our framework. An-
other future extension is to handle skipping of tests,
for example, based on predicates passed when calling
the method test.
We plan to implement some form of IDE support.
This will allow the developer to have a dedicated view
in the IDE, similar to the JUnit view in Eclipse. Run-
ning JNRTEST tests from an IDE or Maven is already
possible since, as shown in Section 3, it is enough to
create a Java file with the main method.
We will investigate the implementation of a simple
code transpiler that, given a JUnit test case, generates
the corresponding JnrTestCase. This will allow us
to perform further experiments and benchmarks with
existing Java open-source projects. Although a more
involved investigation of the execution speed of JN-
RTEST is required, our initial benchmarks are promis-
15
https://jestjs.io/.
16
A predefined, reproducible and well-known state/envi-
ronment for each test.
ing and match the known fact that reflection intro-
duces run-time overhead.
ACKNOWLEDGEMENTS
This work was partially supported by the PRIN
project “T-LADIES” n. 2020TL3X8X.
REFERENCES
Beck, K. (2003). Test Driven Development: By Example.
Addison-Wesley.
Bodden, E., Sewe, A., Sinschek, J., Oueslati, H., and
Mezini, M. (2011). Taming reflection: Aiding static
analysis in the presence of reflection and custom class
loaders. In ICSE, pages 241–250. ACM.
Braux, M. and Noy
´
e, J. (2000). Towards Partially Evaluat-
ing Reflection in Java. In PEPM, pages 2–11. ACM.
Igarashi, A., Pierce, B., and Wadler, P. (2001). Feather-
weight Java: a minimal core calculus for Java and GJ.
ACM TOPLAS, 23(3):396–450.
Landman, D., Serebrenik, A., and Vinju, J. J. (2017). Chal-
lenges for static analysis of Java reflection: literature
review and empirical study. In ICSE, pages 507–518.
IEEE / ACM.
Leotta, M., Cerioli, M., Olianas, D., and Ricca, F. (2020).
Two experiments for evaluating the impact of Ham-
crest and AssertJ on assertion development. Software
Quality Journal, 28(3):1113–1145.
Li, Y., Tan, T., and Xue, J. (2019). Understanding and Ana-
lyzing Java Reflection. ACM TOSEM, 28(2):7:1–7:50.
Livshits, V. B., Whaley, J., and Lam, M. S. (2005). Re-
flection Analysis for Java. In APLAS, volume 3780 of
LNCS, pages 139–160. Springer.
Park, J. G. and Lee, A. H. (2001). Removing Reflec-
tion from Java Programs Using Partial Evaluation. In
Reflection, volume 2192 of LNCS, pages 274–275.
Springer.
Smaragdakis, Y., Balatsouras, G., Kastrinis, G., and
Bravenboer, M. (2015). More Sound Static Handling
of Java Reflection. In APLAS, volume 9458 of LNCS,
pages 485–503. Springer.
Sobernig, S. and Zdun, U. (2010). Evaluating Java runtime
reflection for implementing cross-language method
invocations. In PPPJ, pages 139–147. ACM.
ICSOFT 2023 - 18th International Conference on Software Technologies
376