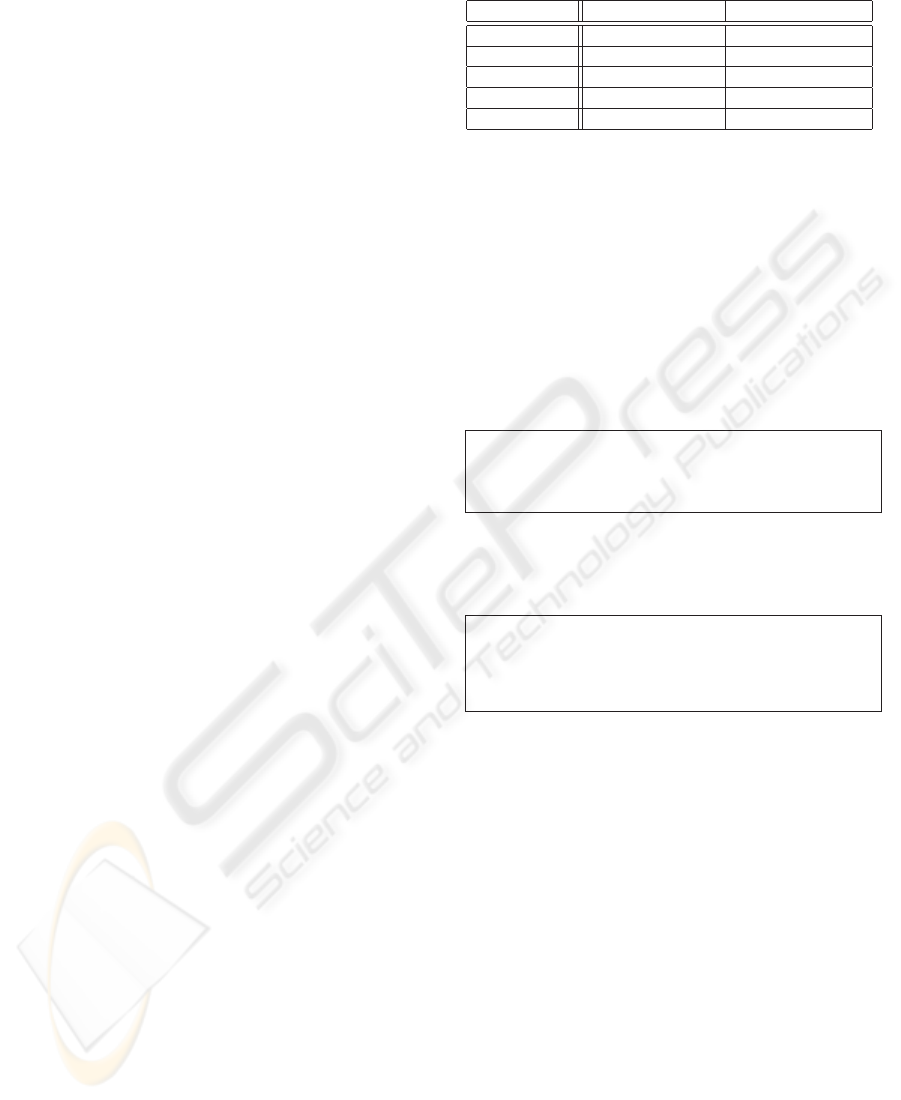
FOP. Feature modules usually contain a set of
classes. These classes are introductions or refine-
ments to existing classes. Thus, feature modules im-
plement mainly increments to a program’s function-
ality. A refinement may extend existing methods by
executing code around a method execution. Although
refinements rely on a reasonable structure of the base
program, they do not expect explicitly represented
variation points (e.g. hooks) for being applicable. A
feature module binds to the natural structure of the
base program and may apply unanticipated changes.
A consequence of its encapsulation property is that a
feature module can implement a variant that concerns
multiple variation points, e.g., a synchronization fea-
ture extends simultaneously a list and its iterator.
However, the fact that feature modules rely on
given structural abstractions defines the minimal
granularity of customization of software built of fea-
ture modules. It is not possible to refine a base fea-
ture at statement level to change existing types, etc.
However, achieving even so customizability at type
level (1) imposes performance penalties due to dy-
namic binding, i.e., implementing a feature against an
abstract class, and (2) it results in redundant code, i.e.,
for each type a distinct feature module. That is, FOP
imposes a complexity overhead at small scales.
GPP. GPP supports program customizability at a
smaller scale than FOP. For example, templates en-
able the programmer to customize program structures
down at type level by parameterizing types of used
variables and arguments. This methodology implies
that programmers have to anticipate changes to and
variants of a program. Variation points are explicit
and fixed; they are an inherent part of the referring
modules. n variation points demand for n parame-
ters. This in-language approach to customization fa-
cilitates static type-safety.
Although templates can be used to implement en-
tire feature modules (Smaragdakis and Batory, 2002),
they are mainly suited for fine-grained customization.
This is because the overhead of complexity to main-
tain the template expressions grows considerably for
large-scale features.
Table 1 summarizes our observation of the prop-
erties of FOP and GPP achieving customizability and
reusability. It is intended to serve as guideline for pro-
grammers to decide when to use which technique.
4 GENERIC FEATURE MODULES
As our analysis revealed, both, GPP and FOP, have
strengths at different scales of customization. Con-
sequently, we propose the notion of generic feature
modules that integrates mechanisms of GPP into FOP.
Table 1: Comparison of FOP and GPP.
FOP GPP
scale large small
granularity methods, classes statements, types
var. points implicit explicit
extensions unanticipated anticipated
locality multiple points single point
Templates. Mixins within feature modules can de-
clare a list of template parameters (Fig. 7). In con-
trast to traditional classes, subsequently applied re-
finements to a class may extend its (possibly empty)
template parameter list. This is useful because in this
way the set of parameterizable types has not to be an-
ticipated up front. Figure 8 depicts a refinement to the
basic list that implements the size feature. The type of
the counter is passed via template parameter; for that,
the template list is extended (Line 1).
1 template <typename _ItemT> class List {
2 typedef _ItemT ItemT;
3 ItemT
*
head;
4 void put(ItemT
*
i) { i->next = head; head = i; }
5 };
Figure 7: A list template.
1 template <typename _ItemT, typename _SizeT>
2 refines class List {
3 typedef _ItemT ItemT; typedef _SizeT SizeT;
4 SizeT size;
5 void put(ItemT
*
i) { super::put(i); size++; }
6 };
Figure 8: Extending the parameter list in a refinement.
However, extending the parameter list implies that
clients have two provide a set of expected parameters,
which may vary depending on the current feature se-
lection. We address this issue later.
Parameterizing feature modules. Templates can
be used to parameterize feature modules statically by
a set of types. Figure 9 depicts a stack of generic
feature modules. Each feature module extends ex-
isting structures, but also the template parameter list.
This enables the individual features to declare new
parameters that are intended for customizing them-
selves. Composing a concrete program out of this set
of features allows the final program to be parameter-
ized with concrete types.
This example illustrates the two-staged nature of
the configuration process imposed by generic feature
modules: (1) a subset of features is selected; (2) the
selected features are parameterized.
ICSOFT 2006 - INTERNATIONAL CONFERENCE ON SOFTWARE AND DATA TECHNOLOGIES
130