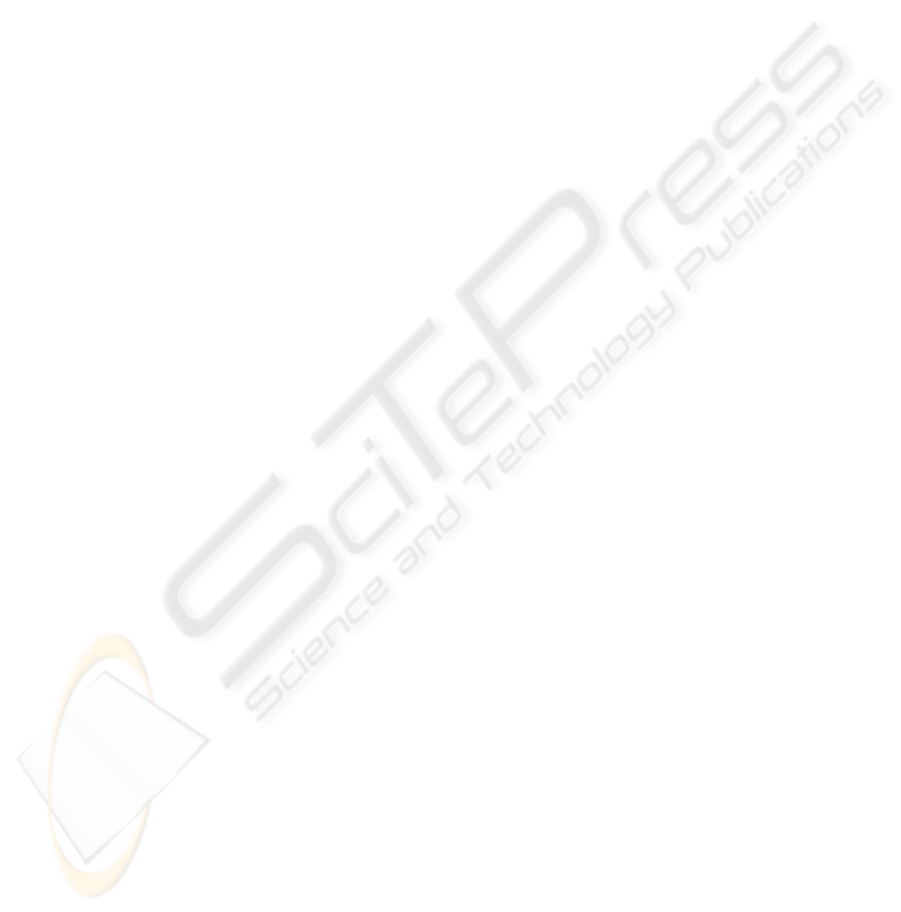
4 CONCLUSIONS AND FUTURE
WORK
This paper has presented an innovative framework
based on the evolution of our first experiences de-
veloping a real application on a distributed functional
language. The proposed architecture could be reused
in other traditional management software develop-
ment and it is a step forward in features like main-
tainability, scalability and availability with regard to
the early architecture.
The implementation of management software us-
ing a functional language may seem an exotic ap-
proach. But the adaptation of O.O. concepts to the
functional environment is a key factor for success.
Another advantage of using Erlang/OTP as the un-
derlying platform for the system development (in ad-
dition to those common in declarative languages), is
that techniques coming from the formal methods area
can be applied in order to improve the system design
and performance (Arts and Benac Earle, 2002), and to
ensure (at least partially) the system correctness (Arts
and S´anchez Penas, 2002). These techniques can be
used more naturally with high level declarative lan-
guages, and their results are specially appreciated in
complex management software.
Also, the design of a full application container
(similar to the available in J2EE (J2EE, 2003)), its de-
sign and development could be a future work line, fo-
cusing on simpler applications where persistence mat-
ters can be hidden. Particularly interesting would be
the development of a persistence layer following the
same philosophy of the Ruby on Rails (RoR) Active
Record, for the Erlang platform. The two main design
principles of RoR, “Don’t repeat yourself” and “Con-
vention over configuration” have proved themselves
as highly productive in this architecture, taking ad-
vantageof the useof simple conventionsand metapro-
gramming, which could be applied in the functional
world.
Finally, as far as lightweight-style user interface
development is concerned, we strongly believe that
the integration in the framework of any kind of inter-
face definition language like XUL (XUL, 2003) will
alleviate this task, and will improve interface design
and maintainability.
ACKNOWLEDGEMENTS
This work has been partially supported by Span-
ish MEyC TIN2005-08986 (FARMHANDS: Recur-
sos Funcionales para la Construccin de Sistemas Dis-
tribuidos Complejos de Alta Disponibilidad).
REFERENCES
Armistice (2002). Armistice, Advanced Risk Management
Information System: Tracking Insurances, Claims and
Exposures. http://www.madsgroup.org/armistice.
Armstrong, J. (2002). Getting Erlang to talk to the outside
world.
Armstrong, J., Virding, R., Wikstr¨om, C., and Williams, M.
(1996). Concurrent Programming in Erlang, Second
Edition. Prentice-Hall.
Arts, T. and Benac Earle, C. (2002). Verifying Erlang
code: a resource locker case-study. In Int. Symposium
on Formal Methods Europe, volume 2391 of LNCS,
pages 183–202. Springer-Verlag.
Arts, T. and S´anchez Penas, J. J. (2002). Global scheduler
properties derived from local restrictions. In Proceed-
ings of ACM Sigplan Erlang Workshop. ACM.
ASN.1 (2003). Introduction to ASN.1.
http://asn1.elibel.tm.fr/en/introduction/index.htm.
Cabrero, D., Abalde, C., Varela, C., and Castro, L. (2003).
Armistice: An experience developing management
software with Erlang. Proceedings of the 2003 ACM
SIGPLAN workshop on Erlang, pages 23–28.
Corba (2003). Object Management Group.
http://www.omg.org.
Gamma, E. et al. (1999). Design Patterns. Elements
of Reusable Object-Oriented Software. Professional
Computing Series. Addison-Wesley.
Gul´ıas, V. M., Abalde, C., Castro, L., and Varela, C. (2005).
A new risk management approach deployed over a
client/server distributed functional architecture. In
Proceedings of 18th International Conference on Sys-
tems Engineering (ICSEng’05). August 16 - 18, 2005,
pages 370–375. IEEE Computer Society.
Gul´ıas, V. M., Abalde, C., Castro, L., and Varela, C. (2006).
Formalisation of a functional risk management sys-
tem. In Proceedings of 8th International Conference
on Enterprise Information Systems (ICEIS’06). May
23 - 27, 2006, pages 516–519. INSTICC Press.
Hibernate (2006). Hibernate homepage.
http://www.hibernate.org/.
J2EE (2003). Enterprise Javabeans 2.0 Specification.
http://java.sun.com/products/ejb/2.0.html.
Marinescu, F. (2002). EJB Design Patterns. Advanced Pat-
terns, Processes, and Idioms. John Wiley & Sons, Inc.
Ruby (2006). Ruby on rails project homepage.
http://www.rubyonrails.org.
XDR (2003). RFC 1832 - XDR: External Data Representa-
tion Standard. http://www.faqs.org/rfcs/rfc1832.html.
XUL (2003). XML User Interface Language.
http://xul.sourceforge.net.
ERLANG/OTP FRAMEWORK FOR COMPLEX MANAGEMENT APPLICATIONS DEVELOPMENT
425