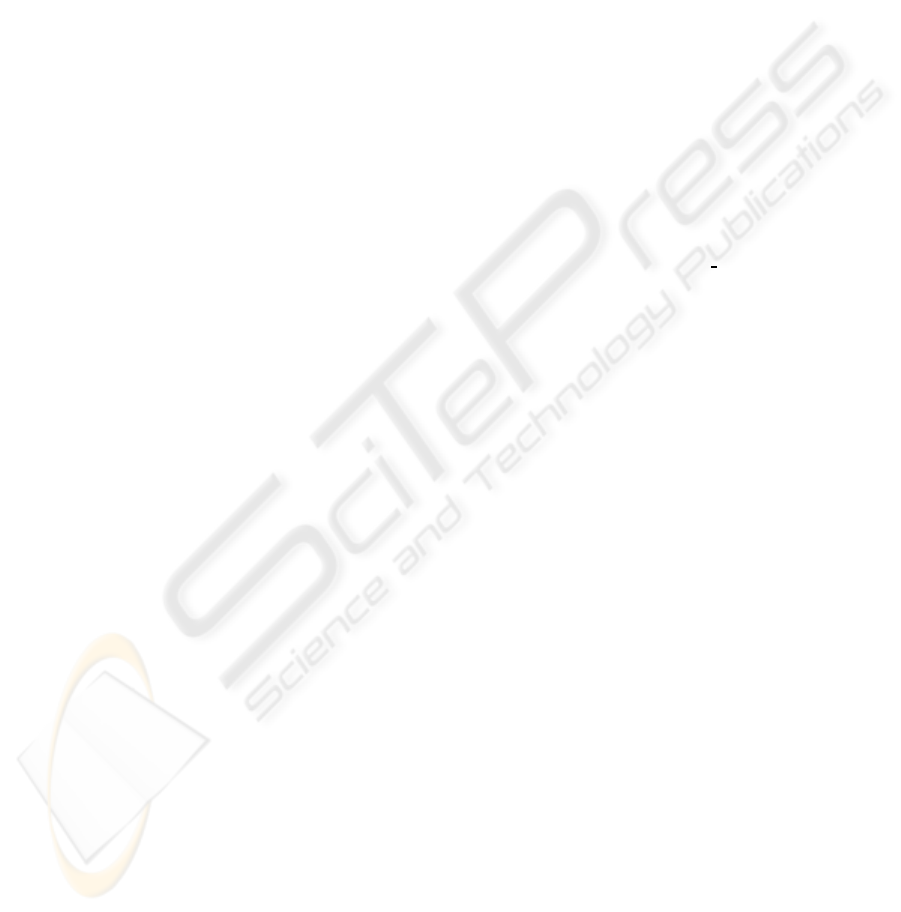
6 CONLUSIONS & FUTURE
WORK
The Open Services Gateway Initiative is a success-
ful attempt to bridge the gap between object-oriented
programming and service-oriented computing, but a
number of challenges remain unsolved. In this pa-
per, we have focused on problems stemming from the
limited expressiveness, comprehensibility and static
guarantees that the OSGi’s LDAP-based query lan-
guage provides. We have also shown that the lifecy-
cle management system requires too much program-
mer intervention and that it too often signals spurious
events.
To solve these problems, we propose an integra-
tion of ServiceJ language concepts into the OSGi
programming model. First, type qualifiers allow the
ServiceJ-to-Java transformer to inject additional in-
structions for transparently handling service failures
and lifecycle changes. Second, declarative opera-
tions allowprogrammersto fine-tune service selection
in a type-safe way. Third, programmers can demar-
cate client-service transactions using session blocks,
leaving the complex management of such transactions
entirely to the ServiceJ middleware.
Future Work. A proper event-notification system
should also support the notification of functional
events. These are events that directly relate to the
business logic of an application (e.g. an event sig-
naling that a file is successfully printed). We plan to
extend ServiceJ’s programming model so as to inte-
grate language support for working with this second
class of events.
REFERENCES
Cervantes, H. and Hall, R. (2003). Automating Service De-
pendency Management in a Service-Oriented Compo-
nent Model. In Proceedings of the 6th Workshop on
Foundations of Software Engineering and Component
Based Software Engineering, pages 379–382.
De Labey, S. and Steegmans, E. (2007). ServiceJ. A Type
System Extension for Programming Web Service In-
teractions. In Proceedings of the Fifth International
Conference on Web Services (ICWS07).
De Labey, S., van Dooren, M., and Steegmans, E. (2006).
ServiceJ: Service-Oriented Programming in Java.
Technical Report KULeuven, CW451, June 2006.
Florescu, D., Gruenhagen, A., and Kossmann, D. (2003).
XL: A Platform for Web Services. In Proceedings of
the First Conference on Innovative Data Systems Re-
search.
Hadim, M. and et al. (2000). Service Combinators for Web
Computing in Distributed Oz. In Conference on Par-
allel and DistributedProcessing Techniques and Appl.
Hall, R. and Cervantes, H. (2003). Gravity: support-
ing dynamically available services in client-side ap-
plications. SIGSOFT Software Engineering Notes,
28(5):379–382.
Hall, R. and Cervantes, H. (2004). Challenges in building
service-oriented applications for OSGi. Communica-
tions Magazine, IEEE, 42(5):144–149.
Huang, Y. and Walker, D. (2003). Extensions to Web Ser-
vice Techniques for Integrating Jini into a Service-
Oriented Architecture for the Grid. In Proceedings of
the International Conference on Computational Sci-
ence.
Kistler, T. and Marais, H. (1998). WebL - A Programming
Language for the Web. In 7th Intl. Conference on the
World Wide Web.
Marples, D. and Kriens, P. (2001). The open service gate-
way initiative: An introductory overview. IEEE Com-
munications Magazine, 39(12).
OSGi (2004). Listeners considered harm-
ful: The whiteboard pattern. In
www.osgi.org/documents/osgi technology/.
OSGi (2006). Open Services Gateway Initiative Specifica-
tion v4.0.1 – http://www.osgi.org.
Papazoglou, M. (2003). Service Oriented Computing: Con-
cepts, Characteristics and Directions. In Proceedings
of the 4th International Conference on Web Informa-
tion Systems Engineering.
Pratikakis, P., Spacco, J., and Hicks, M. (2004). Transpar-
ent Proxies for Java Futures. In OOPSLA ’04: Pro-
ceedings of the 19th annual ACM SIGPLAN confer-
ence on Object-oriented programming, systems, lan-
guages, and applications, pages 206–223, New York,
NY, USA. ACM.
Sun (2005). The Jini Architecture Specification and API
Archive – http://www.jini.org.
Tschantz, M. S. and Ernst, M. D. (2005). Javari: Adding
reference immutability to Java. In Object-Oriented
Programming Systems, Languages, and Applications
(OOPSLA 2005), pages 211–230, San Diego, CA,
USA.
van Dooren, M., Vanderkimpen, K., and De Labey, S.
(2007). The Jnome and Chameleon Metamodels for
OOP.
ENASE 2008 - International Conference on Evaluation of Novel Approaches to Software Engineering
166