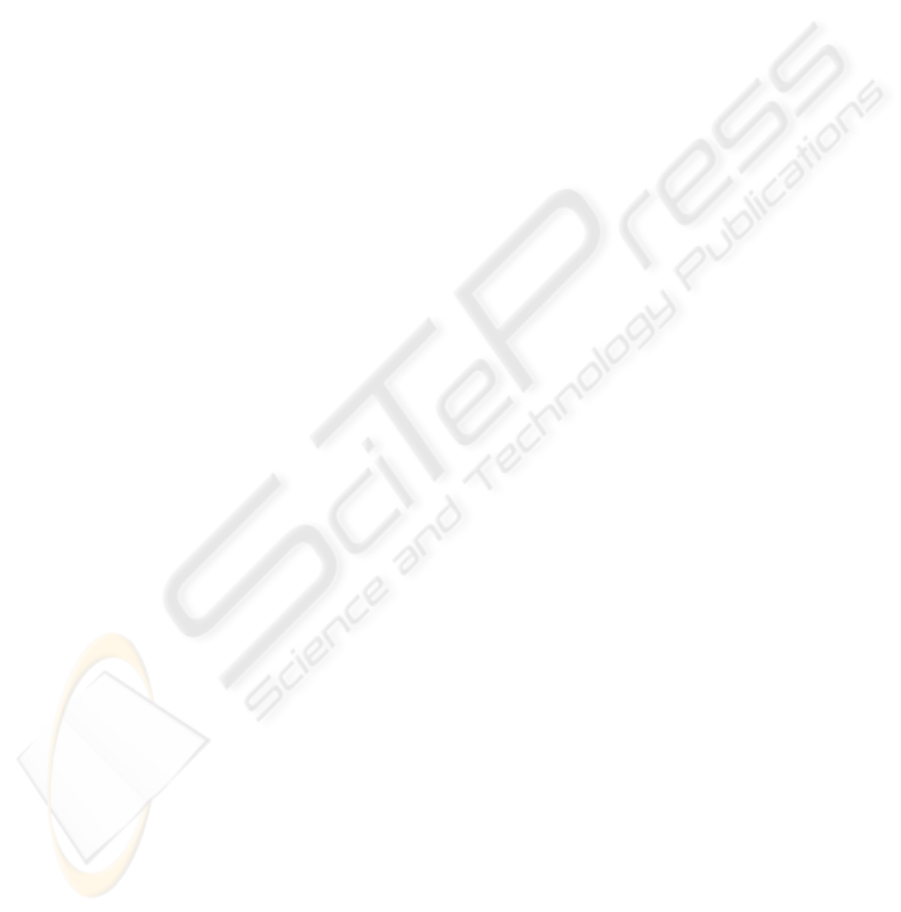
raise an exception when this happens but others,
like PostgreSQL (Douglas and Douglas, 2003) do
not detect this situation, and allow the change of
the isolation level without setting up to false the
auto-commit flag. With Aspy we can deal with
this situation and a modification of the code to
avoid it is minimal, just by adding one line to the
Driver when an Isolation Level change occurs.
2. Suspicious accesses to restricted tables can be de-
tected when the code of the application is not pro-
vided, see (Hu and Panda, 2003) for more infor-
mation. This is a very important utility for users
and enterprises that have bought a third-party ap-
plication/library and want to be sure about the
confidentiality of their data. As in the first case, a
simple modification can be made to directly avoid
these operations or allowing only a subset of per-
mitted ones.
3. Aspy generated logs can be the fed to an expert
system that makes suggestions on query optimiza-
tion to the programmer or on syntactic rules to the
DBMS administrator. Our efforts are going in this
way, by analyzing performance techniques shown
in SQL Performance Tuning to build optimizers
which can detect and suggest changes to improve
productivity. Note, however, that modern DBMSs
have very powerful query optimizers and that our
intent is simply to discourage the use of some sen-
tences that can not be adequately optimized by the
underlying DBMS.
4. Another kind of expert system, that might use
Aspy, can help the administrator to improve the
scheme, by suggesting indexes on the most ac-
cessed fields and tables. Due to the fact that all
the information can be logged, this system can be
made by identifying each field and table that was
referenced in the operation and generating statis-
tics with that information.
5. Cold replication can be made using the log file and
a program that reproduces the operations, to an-
other database. This is specially useful when het-
erogeneous systems, like different DBMS, want
to be compared. It is also needed for raising
DBMS at debug phase in order to detect imple-
mentation errors. It can be a fast alternative to
(Krishnamoorthy, 1999).
6. In order to detect bad practices, such as ignor-
ing exceptions by a deployed program, a logger
with exception reporting can be used. So final
users will detect forbidden accesses, failed con-
nections, rollback operations and other behaviors
produced when a communication with a database
takes place. As it was mentioned before, this in-
troduces an overload, so the decision should be
taken by the administrator in order to maintain a
good performance.
7 RELATED AND FURTHER
WORK
As mentioned earlier in this paper, p6spy is a good ap-
proach to the main goal, but its installation requires a
more intrusive procedure and updating the whole tool
represents an expensive cost of development. Some
similar tools, like LOG4PLSQL (The LOG4PLSQL
project team, 2002), are also interesting but, when us-
ing LOG4PLSQL, the deployment is a hard process
due to modifications that should take place at DBMS
side. When comparing with Oracle Log Buffers, see
(Oracle Backup and Recovery, 2008), we can con-
clude that obtaining this information at DBMS level is
a hard and expert process, and a specific knowledge of
the DBMS and the version is required (to access to the
committed and uncommitted operations). However,
one main lack appears when using LOG4PLSQL and
Oracle buffers, due to obtaining operation informa-
tion at this level is less expressive than at top level,
therefore, some relevant data will be lost (such as
JDBC methods, parameters names, and their values)
and it can be insufficient to feed the developer at the
debug phase of the application.
Our efforts are focused on developing solutions
that use the log generated by Aspy to reach the ap-
plications presented in Section 6. Some need a bigger
effort than others, but most of them have a common
approach, which can be implemented as a log line an-
alyzer. Depending on every case, analysis can be done
off-line or on-line but its goal is to provide advices to
improve software quality and correctness.
We are planning to employ Aspy as the central
componentof a larger system, which provides support
for database query analyzer modules. Analyzer mod-
ules can be either static or dynamic. Static modules
process the Aspy log output offline, whereas dynamic
modules are employed at run-time.
In order to support simple programming of ana-
lyzer modules, we intend to define a query language
providing basic primitives for log processing. Fur-
thermore, we will investigate the possibility of pro-
viding a graphic user interface that allows the con-
struction of analyzers.
Finally, we plan to define an API that allows the
interception and filtering of database accesses at run
time. Such an interface could be used, for example,
for security purposes.
ICSOFT 2008 - International Conference on Software and Data Technologies
110