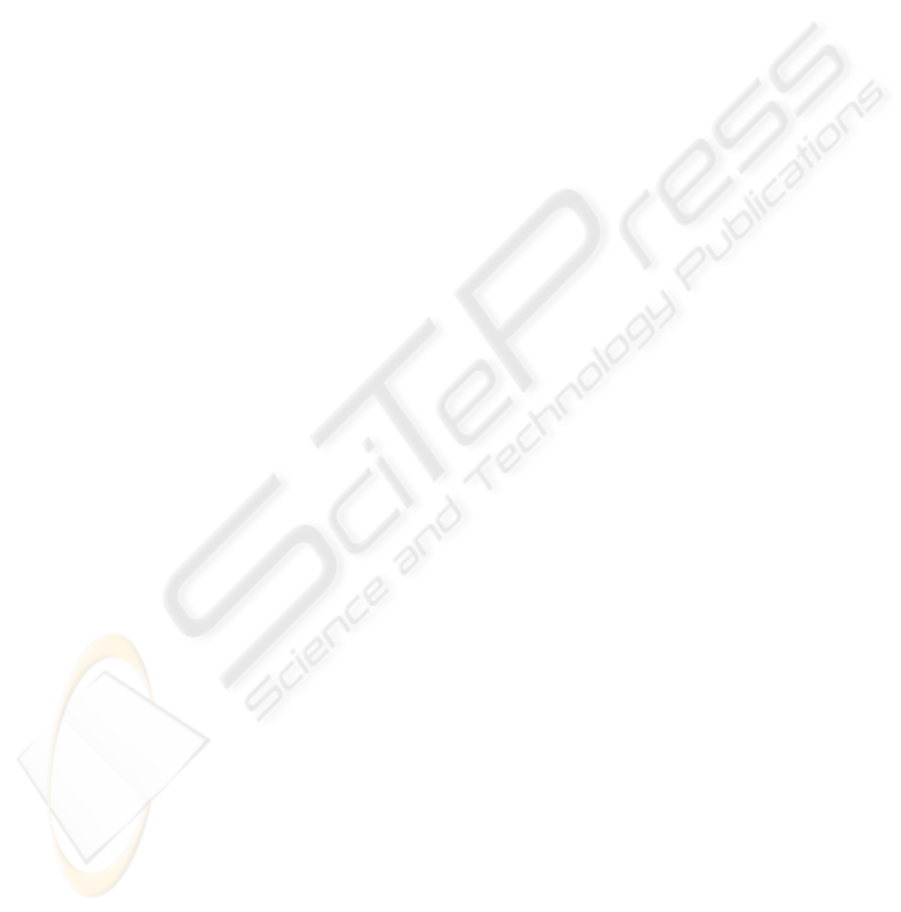
In contrast to previous research the presented ap-
proach offers: (1) An almost standardized way of rep-
resenting programs as constraints; (2) Debugging is
put down to constraint solving where a lot of research
is devoted to constraint solving algorithms; (3) The
integration of assertions and unit tests can be easily
done in our case. There is no need for a special treat-
ment. Assertions can be added to the compiled pro-
gram on the fly during a debugging session; And (4)
The debugging results depends on the syntax and the
semantics of a programming language.
Of course the complexityof debugging is still high
and improvements of both the solving algorithms and
the conversion process are necessary. The handling
of object-oriented features, multi-threaded programs,
and exception are still open issues. However, in spe-
cialized areas like the embedded systems domain, the
application of the presented approach is in reach.
REFERENCES
Aycock, J. and Horspool, N. (2000). Simple generation of
static-single assignment form. In Proceedings of the
9th International Conference on Compiler Construc-
tion (CC), pages 110–124.
Ceballos, R., Casca, R. M., Valle, C. D., and Borrego, D.
(2006). Diagnosing errors in dbc programs using con-
straint programming. In Selected Papers from the 11th
Conference of the Spanish Association for Artificial
Intelligence (CAEPIA 2005), volume 4177 of Lecture
Notes in Computer Science.
Ceballos, R., Gasca, R., Valle, C. D., and Rosa, F. D. L.
(2003). A constraint programming approach for soft-
ware diagnosis. In Ronsse, M. and Bosschere, K. D.,
editors, Proceedings of the Fifth International Work-
shop on Automated Debugging, Ghent, Belgium.
Collavizza, H. and Rueher, M. (2006). Exploration of
the capabilities of constraint programming for soft-
ware verification. In Proceedings of Tools and Algo-
rithms for the Construction and Analysis of Systems
(TACAS), pages 182–196. Springer, Vienna, Austria.
Cytron, R., Ferrante, J., Rosen, B. K., Wegman, M. N., and
Zadeck, F. K. (1991). Efficiently computing static
single assignment form and the control dependence
graph. ACM TOPLAS, 13(4):451–490.
Dechter, R. (1992). Constraint networks. In Encyclopedia
of Artificial Intelligence, pages 276–285. Wiley and
Sons.
Dechter, R. (2003). Constraint Processing. Morgan Kauf-
mann.
Dechter, R. and Pearl, J. (1988). Network-based heuristics
for constraint-satisfaction problems. Artificial Intelli-
gence, 34:1–38.
Dechter, R. and Pearl, J. (1989). Tree clustering for con-
straint networks. Artificial Intelligence, 38:353–366.
DeMillo, R. A., Pan, H., and Spafford, E. H. (1996). Critical
slicing for software fault localization. In International
Symposium on Software Testing and Analysis (ISSTA),
pages 121–134.
Ducass´e, M. (1993). A pragmatic survey of automatic
debugging. In Proceedings of the 1st International
Workshop on Automated and Algorithmic Debugging,
AADEBUG ’93, Springer LNCS 749, pages 1–15.
Freuder, E. C. (1982). A sufficient condition for backtrack-
free search. Journal of the ACM, 29(1):24–32.
Gotlieb, A., Botella, B., and Rueher, M. (1998). Au-
tomatic test data generation using constraint solving
techniques. In Proc. ACM ISSTA, pages 53–62.
Gupta, N., He, H., Zhang, X., and Gupta, R. (2005). Locat-
ing faulty code using failure-inducing chops. In Auto-
mated Software Engineering (ASE), pages 263–272.
Jackson, D. (2006). Software abstractions: logic, language,
and analysis. MIT Press.
Kamkar, M. (1998). Application of program slicing in al-
gorithmic debugging. Information and Software Tech-
nology, 40:637–645.
K¨ob, D. and Wotawa, F. (2006). Fundamentals of debug-
ging using a resolution calculus. In Baresi, L. and
Heckel, R., editors, Fundamental Approaches to Soft-
ware Engineering (FASE’06), volume 3922 of Lecture
Notes in Computer Science, pages 278–292, Vienna,
Austria. Springer.
Mackworth, A. (1987). Constraint satisfaction. In Shapiro,
S. C., editor, Encyclopedia of Artificial Intelligence,
pages 205–211. John Wiley & Sons.
Mayer, S. (2003). Static single-assignment form
and two algorithms for its generation. Semi-
nar Work, Winter Term 2002/03, University of
Konstanz, http://www.inf.uni-konstanz.de/dbis/
teaching/ws0203/pathfinder/download/ mayers-
ausarbeitung.pdf.
Reiter, R. (1987). A theory of diagnosis from first princi-
ples. Artificial Intelligence, 32(1):57–95.
Shahmehri, N., Kamkar, M., and Fritzson, P. (1995). Us-
ability criteria for automated debugging systems. J.
Systems Software, 31:55–70.
Stumptner, M. and Wotawa, F. (1998). A Survey of Intelli-
gent Debugging. AI Communications, 11(1).
Zeller, A. (1999). Yesterday, my program worked. today,
it doesn’t. why? In Proceedings of the Seventh Euro-
pean Software Engineering Conference/Seventh ACM
SIGSOFT Symposium on Foundations of Software En-
gineering (ESEC/FSE), pages 253–267.
Zeller, A. and Hildebrandt, R. (2002). Simplifying and iso-
lating failure-inducing input. IEEE Transactions on
Software Engineering, 28(2).
Zhang, X., He, H., Gupta, N., and Gupta, R. (2005). Exper-
imental evaluation of using dynamic slices for fault
localization. In Sixth International Symposium on Au-
tomated & Analysis-Driven Debugging (AADEBUG),
pages 33–42.
LOCALIZING BUGS IN PROGRAMS - Or How to Use a Program’s Constraint Representation for Software Debugging?
95