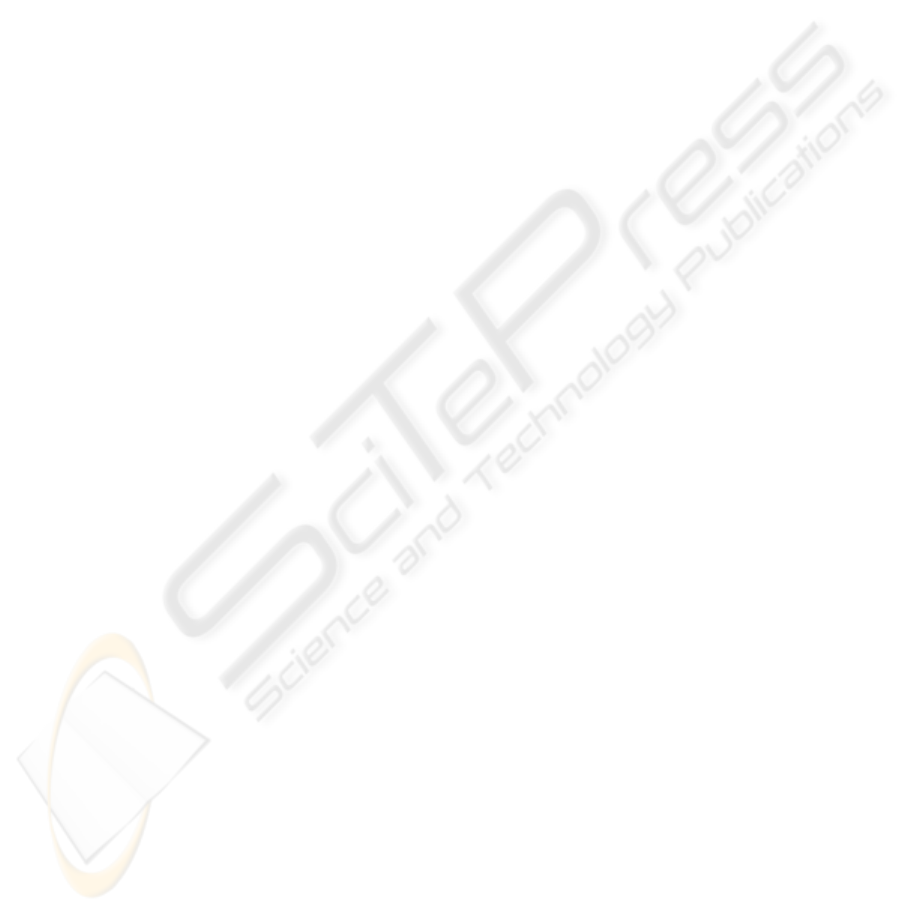
5 CONCLUSIONS AND FUTURE
WORK
In this paper we presented a new, modular system for
automated detection of buffer overflows in programs
written in C. Our system performs flow-sensitive and
inter-procedural static analysis. The system gener-
ates correctness and incorrectness conditions for in-
dividual commands, which are then tested for validity
by an automated theorem prover for linear arithmetic.
Some of the main noveltiesand advantagesof our sys-
tem are: its modular and flexible architecture (so its
building blocks can be easily changed and updated),
an external and open database of conditions (so the
underlying reasoning rules are not hard-coded into
the system so the user can vary them), buffer over-
flow correctness conditions given explicitly in logical
terms, using external theorem provers. We presented
the current prototype implementation of the proposed
system, the Fado tool, that gives promising results.
The presented system is a subject of further, more
detailed evaluation, improvements, and development.
For instance, although heuristics for dealing with
loops are efficient and can have a wide range, for
the next stage of development, we are planning to ex-
tend our system to preform full analysis of loops (in
a similar manner as proposed in some modern sys-
tems (Dor et al., 2003)) and of user-defined functions,
so the system will be sound and its inter-procedural
analysis will be fully automatic. In addition, we are
planning to use theorem provers with more expres-
sive background theories. Our goal is to make Fado
efficiently applicable to long, real-world critical pro-
grams. Thanks to the tool’s flexible architecture, we
are also planning to extend it for other sorts of pro-
gram analysis (e.g., testing for memory leaks).
REFERENCES
P. Cousot and R. Cousot. (2004) Basic Concepts of Abstract
Interpretation. In Building the Information Society.
Kluwer, 2004.
Cowan, C., Wagle, P., Pu, C., Beattie, S., and Walpole, J.
(2000). Buffer overflows: Attacks and defenses for
the vulnerability of the decade. In Proceedings of the
DARPA Information Survivability Conf. and Expo.
Dor, N., Rodeh, M., and Sagiv, M. (2003). Cssv: Towards
a realistic tool for statically detecting all buffer over-
flows in c. In Proceedings of the ACM SIGPLAN 2003
conference on Programming language design and im-
plementation. ACM Press.
Dutertre, B. and De Moura, L. (2006). A fast linear-
arithmetic solver for dpll(t). In CAV 2006, vol. 4144
of LNCS. Springer.
Ellenbogen, R. (2004). Fully automatic verification of ab-
sence of errors via interprocedural integer analysis.
Master’s thesis, University of Tel-Aviv, Israel.
Fillitre, J.-C. and March, C. (2007). The why/-
krakatoa/caduceus platform for deductive program
verification. In CAV, vol. 4590 of LNCS. Springer.
Holzmann, G. (2002). Static source code checking for user-
defined properties. In Proceedings of 6th World Con-
ference on Integrated Design and Process Technology.
Kratkiewicz, K. and Lippmann, R. (2005). Using a diagnos-
tic corpus of c programs to evaluate buffer overflow
detection by static analysis tools. In Workshop on the
Evaluation of Software Defect Detection Tools.
Larochelle, D. and Evans, D. (2001). Statically detecting
likely buffer overflow vulnerabilities. In USENIX Se-
curity Symposium.
PolySpace Technologies (2003). Polyspace c verifier. Paris,
France. http://www.polyspace.com.
Ranise, S. and Tinelli, C. (2003). The SMT-
LIB Format: An Initial Proposal. on-line at:
http://goedel.cs.uiowa.edu/smt-lib/.
Simon, A. and King, A. (2002). Analyzing String Buffers
in C. In International Conference on Algebraic
Methodology and Software Technology, volume 2422
of LNCS. Springer.
Viega, J., Bloch, J., Kohno, Y., and McGraw, G. (2000).
Its4: A static vulnerability scanner for c and c++ code.
In 16th Annual Computer Security Applications Con-
ference (ACSAC’00).
Viega, J. and McGraw, G. (2002). Building Secure Soft-
ware. Addison-Wesley.
Wagner, D., Foster, J., Brewer, E., and Aiken, A. (2000). A
first step towards automated detection of buffer over-
run vulnerabilities. In Symposium on Network and
Distributed System Security.
Wilander, J. and Kamkar, M. (2002). A comparison of pub-
licly available tools for static intrusion prevention. In
Proceedings of the 7th Nordic Workshop on Secure IT
Systems (Nordsec 2002).
Wilander, J. and Kamkar, M. (2003). A comparison of pub-
licly available tools for dynamic buffer overflow pre-
vention. In Proceedings of the 10th Network and Dis-
tributed System Security Symposium.
Xie, Y., Chou, A., and Engler, D. (2003). Archer: us-
ing symbolic, path-sensitive analysis to detect mem-
ory access errors. In Proceedings of the 9th European
software engineering conference. ACM Press.
Yorsh, G. and Dor, N. (2003). The Design of CoreC. on-line
at: http://www.cs.tau.ac.il/ gretay/GFC.htm.
Zhivich, M., Leek, T., and Lippmann, R. (2005). Dynamic
buffer overflow detection. In Workshop on the Evalu-
ation of Software Defect Detection Tools.
Zitser, M., Lippmann, R., and Leek, T. (2004). Testing static
analysis tools using exploitable buffer overflows from
open source code. In Proceedings of the 12th ACM
SIGSOFT international symposium on Foundations of
software engineering. ACM.
ICSOFT 2008 - International Conference on Software and Data Technologies
36