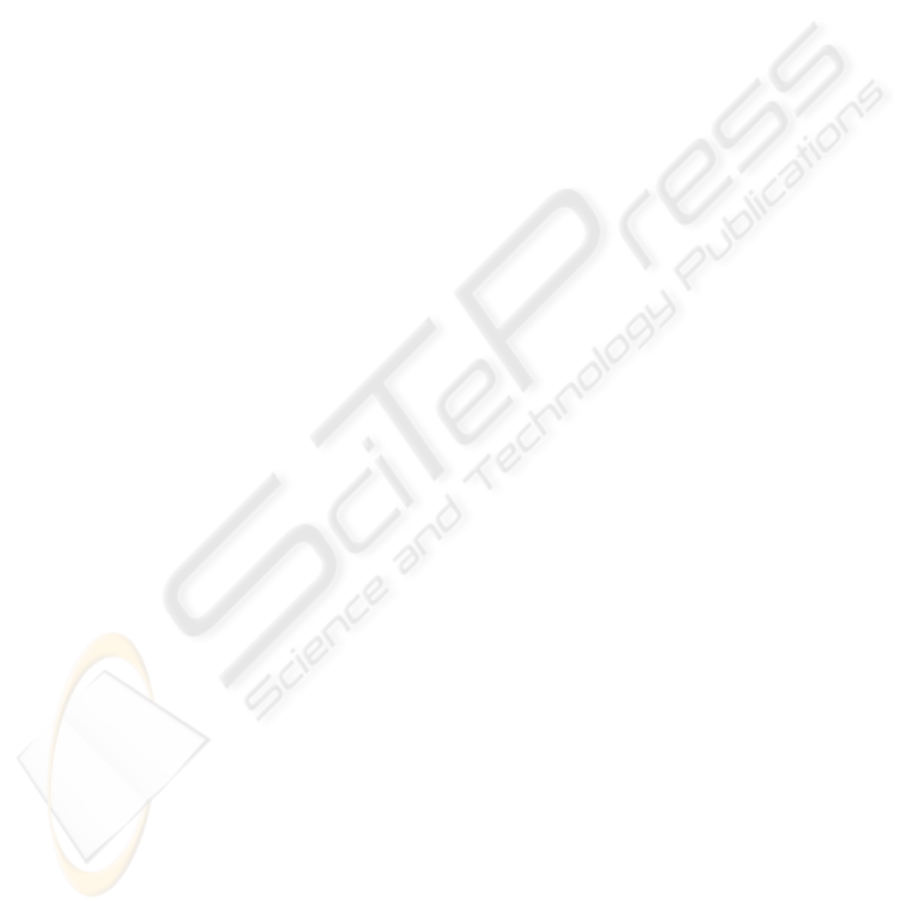
3.1 Mapping the Reactor Design
Pattern
If the reactor design pattern presented above had
been used for the NIO Framework without mod-
ification, every application-specific ConcreteEvent-
Handler would still have to take care of many NIO
specific details. These include buffers, queues, in-
complete write operations, encryption of data streams
and much more. To provide a simple API to Java net-
work application programmers, the NIO Framework
was complemented with several additional helper
classes and interfaces that will be introduced in the
following sections.
The concepts and techniques used to design and
implement a safe and scalable framework that effec-
tively exploits multiple processors are presented in
(Peierls et al., 2005).
A simplified model of the NIO Framework core is
shown in Figure 2.
The blue UML elements (Runnable, Thread, Se-
lector, SelectionKey and Executor) are part of the Java
Development Kit (JDK). The interface Runnable and
the class Thread were part of JDK from the very be-
ginning, Selector and SelectionKey have been added
to the JDK with the NIO package in JDK v1.4 and
the interface Executor was added with the concur-
rency package in JDK v1.5. The yellow UML el-
ements (ChannelHandler, HandlerAdapter and Dis-
patcher) are the essential core classes of the NIO
Framework.
The Dispatcher is a Thread that runs in an endless
loop, processes registrations of ChannelHandlers with
a channel (a nexus for I/O operations that represents
an open connection to an entity such as a network
socket) and uses an Executor to offload the execution
of selected HandlerAdapters. The Executor interface
hides the mechanics of how each task will be exe-
cuted, including details of thread use, scheduling, etc.
This abstraction is necessary because the NIO Frame-
work may be used on a wide range of systems, from
low-cost embedded devices up to high-performance
multi-core servers.
The class Selector determines which registered
channels are ready.
The class SelectionKey associates a channel with
a Selector, tells the Selector which events to monitor
for the channel and holds a reference to an arbitrary
object, called “attachment”. In the current architec-
ture the attachment is a HandlerAdapter.
The EventHandler from the reactor design pattern
is split up into several components. The first compo-
nent is the class HandlerAdapter. It manages all the
operations on a channel (connect, read, write, close)
and its queues, interacts with the Selector and Selec-
tionKey classes and, most importantly, hides and en-
capsulates most NIO details from higher level classes
and interfaces.
The second EventHandler component in the NIO
Framework is the interface ChannelHandler. It de-
fines the methods that any application-specific chan-
nel handler class has to implement so that it can be
used in the NIO framework. These include:
public void channelRegistered(
HandlerAdapter handlerAdapter)
This method gets called when a channel was regis-
tered at the Dispatcher. It is mostly used on server
type applications to send a welcome message to
clients that just connected.
public InputQueue getInputQueue()
This method returns the InputQueue that will be used
by the HandlerAdapter, if there is data to be read from
the channel.
public OutputQueue getOutputQueue()
This method returns the OutputQueue that will be
used by the HandlerAdapter, if there is data to be writ-
ten to the channel.
public void handleInput()
The HandlerAdapter calls this method, if the In-
putQueue has new data to be read from the channel.
public void inputClosed()
This method gets called by the HandlerAdapter, if no
more data can be read from the InputQueue.
public void channelException(
Exception exception)
The HandlerAdapter calls this method, if an exception
occurred while reading from or writing to the channel.
Not shown in Figure 2 are all the application-
specific channel handlers that implement the interface
ChannelHandler. They represent the ConcreteEvent-
Handler of the reactor design pattern. Because the
details of the method handleInput() may vary with
every specific handler they are outside the scope of
the NIO Framework.
Table 1 shows the mappings from the reactor de-
sign pattern to the NIO Framework.
3.2 Parallelization
Some parts of the Java NIO Framework are paral-
lelized by default, other parts can be customized to
be parallelized.
ICSOFT 2008 - International Conference on Software and Data Technologies
208