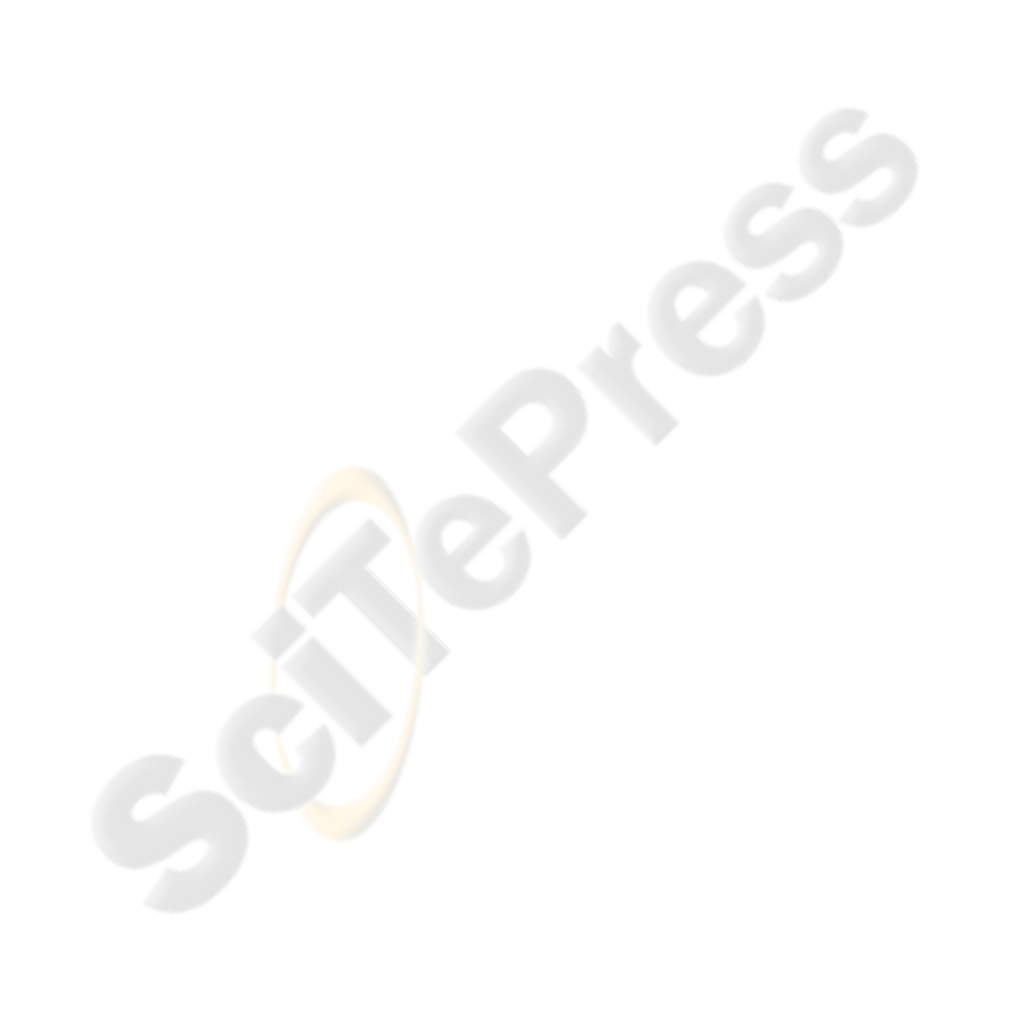
Using Kernel Meta-metamodel (KM3, part of the
AMMA tool suite) (Jouault and Bézivin, 2006), a
snippet of the domain-specific metamodel for our
case study application is shown in Figure 5. In this
figure, the RaceCar class is the main class that
defines the abstract syntax of the language. It
consists of sensors, controllers, processors,
communication channels and other hardware
devices. The concrete syntax for the RaceCar model
is written in TCS, however, for brevity is not shown
here.
Using model transformation techniques, the low-
level code required to run the system can be
generated for the RaceCar model. Thus, by focusing
on graphical or textual based DSMLs, domain
engineers can build domain specific embedded tools
that can raise the abstraction level of hardware
centric embedded programs.
3 SUMMARY
AND CONCLUSIONS
The proliferation of embedded software in everyday
life has augmented the conformity and invisibility of
software. As demands for such software increase,
future requirements will necessitate new strategies
for improved modularization, construction and
restructuring in order to support the requisite
adaptations (Masuhara and Kiczales, 2003). Proven
software engineering techniques like AOP and MDE
that occupy a bigger space in the non-embedded
domain must be investigated and disseminated into
the embedded space.
In this paper, we demonstrated how each of these
techniques can improve the construction effort of
embedded software. A greater emphasis must be
given to specialized tools and domain specific
languages that can raise the abstraction level from
hardware centric applications to software centric
models and analysis engines.
A growing challenge in the embedded world has
been to reduce the energy consumption of battery
powered devices. As part of future work, we will
look into software centric static and dynamic
optimizations embedded mobile systems that
combine the power of each of these techniques.
REFERENCES
Day, R. (2005) 'The Challenges of an Embedded Software
Enginee', Embedded Technology Journal.
Jouault, F. and Bézivin, J. (2006) 'KM3: A DSL for
Metamodel Specification', Formal Methods for Open
Object-Based Distributed Systems, Bologna, Italy,
171-185.
Laddad, R. (2003) AspectJ in Action: Practical Aspect-
Oriented Programming, Manning.
Lédeczi, Á., Bakay, A., Maroti, M., Volgyesi, P.,
Nordstrom, G., Sprinkle, J. and Karsai, G. (2001)
'Composing Domain-Specific Design Environments',
IEEE Computer, vol. 34, no. 11, November, pp. 44-51.
Masuhara, H. and Kiczales, G. (2003) 'Modeling
Crosscutting in Aspect-Oriented Mechanisms',
European Conference on Object-Oriented
Programming, Springer-Verlag LNCS 2743,
Darmstadt, Germany, 2-28.
Schmidt, D. (2006) 'Model-Driven Engineering',
IEEE Computer, February.
Shi, F. (2004) Embedded Systems Modeling
Language,http://www.omg.org/news/meetings/worksh
ops/MIC_2004_Manual/06-4_Shi_etal.pdf.
Spinczyk, O., Gal, A. and Schröder-Preikschat, W. (2002)
'AspectC++: An Aspect-Oriented Extension to C++',
International Conference on Technology of Object-
Oriented Languages and Systems, Sydney, Australia,
53-60.
UML (2009) Unified Modeling Language 2.2,
http://www.omg.org/technology/documents/formal/um
l.htm.
ICSOFT 2009 - 4th International Conference on Software and Data Technologies
308