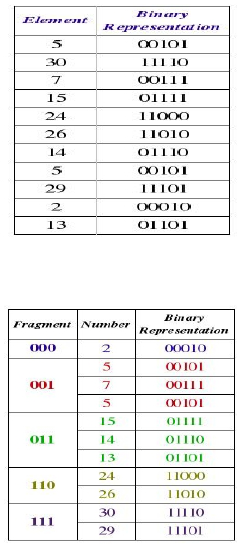
elements of each fragment isn’t yet sorted. The sec-
ond phase uses a simple but efficient technique to sort
these fragments based on the binary representation of
the fragments’ elements.
2.1.1 Phase One, Bucket Sort
The functionality of this phase is to generate a num-
ber of sequences from the original sequence such that
for any two sequences Si and Sj, any element in Si is
less than all element of Sj, when ever i is less than j.
Let’s consider an array of unsigned integer. Standared
integer numbers are 32 bits. In this level we will sort
these elements based on the first k MSBs without any
consideration of the remainding bits. The process of
choosing the value of k will be discussed later when
analyzing the time complexity of the algorithm. This
level of sorting could be easily performed in linear
time by using some sort of counting sort keeping in
mind that the number of MSBs involved in this pro-
cess should be kept withen a reasonable range to allow
the counting sort to operate in a linear time and space
complexities.(Thomas H. Cormen and Stein, 2001)
This stage of sorting could be formulated with the
following terms, assuming ascending sorting is re-
quired:
• Generate an array of size 2
k
and initialize all its el-
ements to zero. This array will act as the counter
array in the counting sort technique. The counter
array used here will differ slightly from the clas-
sical counter array. An element at the index q in
a classical counter array will hold the number of
elements in the sequence to be sorted having the
value q, while an element at index q in the counter
array that is used here will hold the number of ele-
ments in the sequence having the k (MSBs) equal
to the binary representation of the value q. This
step costs constant time.
• To fill the counter array, loop over every element
in the array. Using bit masking obtain the value
of the k MSBs in each element and increment the
corresponding item in the counter array by one.
This step consumes linear time proportional to the
size of the list to be sorted.
• Based on the resulting counter array. Partion the
original sequence into virtual fragments. This step
could predict the number of the resulting frag-
ments, the size of each fragment, and the correct
starting and ending position of these fragments in
the final sorted list. This step could be performed
in exponential time complexity proportional to the
value which is chosen for k. This reflects the crit-
ical operation of choosing the value of k to keep
the complexity of this step linear compared to the
size of the original sequence.
• Based upon the resulting information that is ob-
tained from the previous step, loop on every ele-
ment in the list and insert it into the proper posi-
tion in a new list, so that the resulting list will be
composed of some virtual portions that are sorted
relatively to each other but having their elements
unsorted yet as stated before. Again the time com-
plexity of this step is linear proportional to the size
of the original list.
The cost of this phase is 2n+2
k
considering the time
and n+2
k
considering the memory. Choosing the
proper value for k keeps the time and spatial com-
plexities of this phase linear compared to the size of
the original list as discussed later. Figure one and two
give an example of applying phase one of the algo-
rithm on an array with integer elements. In this ex-
ample n= 11 , m = 5 and k = 3. (Mahmoud and Al-
Ghreimil, 2006; Akl, 1990)
Figure 1: Unsorted list.
Figure 2: Fragments resulting from phase 1.
2.1.2 Phase Two, Binary based Sort
The second phase of the algorithm is to sort the virtual
portions that construct the list resulting from phase
one. Here some sort of radix sort is used. Instead of
considering the size of the list only, this stage of sort-
ing takes into consideration the binary representation