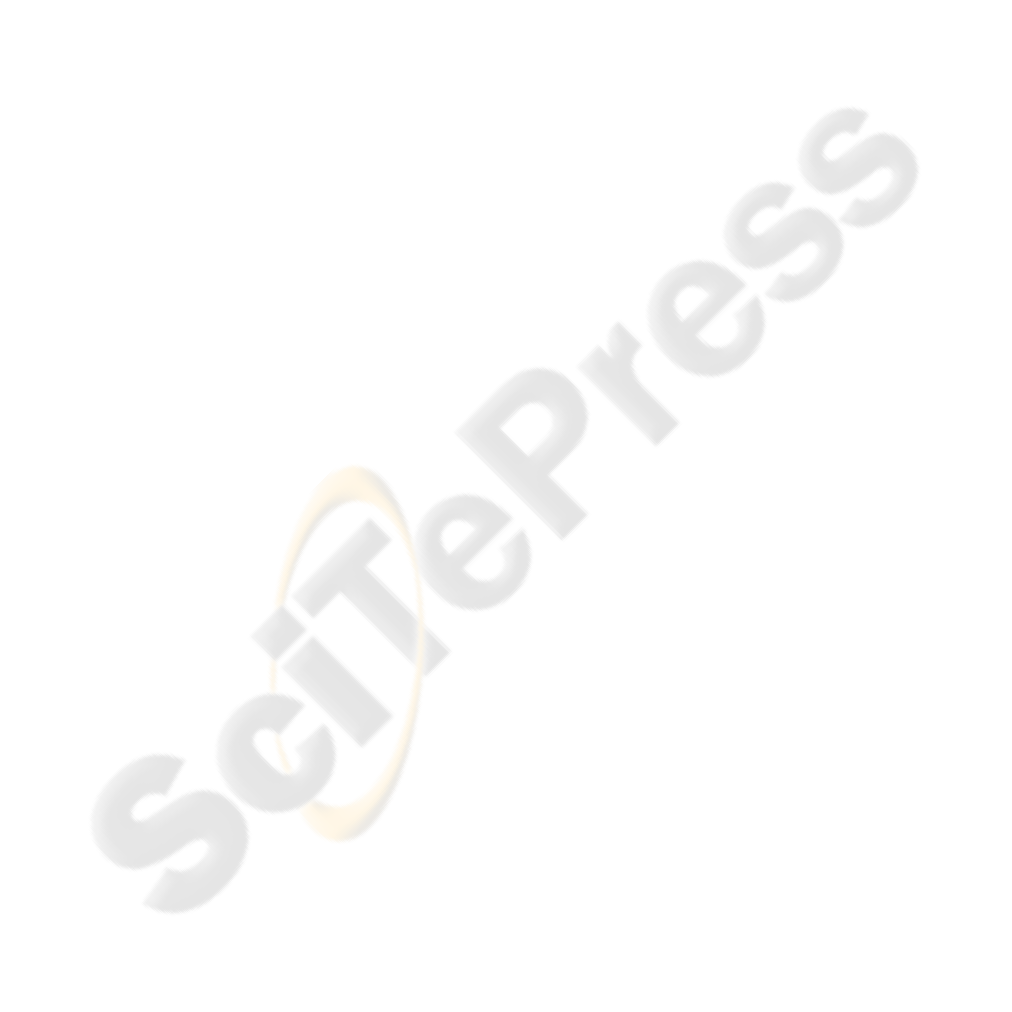
4 TOOL DESIGN AND
IMPLEMENTATION
C# .NET and WPF (Windows Presentation
Foundation) were selected early on in the design
process, partially due to some previous experience
with .NET development, but also because .NET and
WPF in particular offer several features that
significantly enhanced the software. WPF allows for
faster and more streamlined development of visual
elements of an application through the use of
XAML, and has much richer features for graphical
applications. The data binding capabilities of WPF
are extensively used in this application, which
greatly simplifies the logistics of moving collections
of visual objects around the screen by allowing them
to be bound together and automatically handled as a
single unit. Additionally, the flexibility and power of
anonymous methods and lambda expressions in
.NET allow for one of the most unique features of
this software – a variable-speed, interactive
execution of data structure functions which can be
run automatically or stepped through line by line.
This application uses a custom visual framework
designed specifically to represent and manipulate
data structures as visual objects. In all but a few
cases, the structures with which users interact are
composed of the visual objects themselves.
Individual data structures are built from a base data
structure class which handles basic manipulation of
these objects, can arrange them into independent
groups, display value-type variables in a structure,
use illustration objects to highlight pointers, loop
iterators or other peripheral elements, and saves and
restores structure states to allow undo and redo
operations. By using a smart canvas as a drawing
surface for these visual objects, they can be
automatically scaled and centered, allowing a
structure to grow arbitrarily large.
Each data structure function occurs in two
phases: first, a look-ahead phase pre-determines
everything that will occur in a particular execution
of a function based on user input, and in doing so
creates a list of anonymous delegates which act as
small, dynamically generated functions each
representing a single line of code. A controller then
steps through these functions one at a time, allowing
the user to demonstrate them slowly, speed through
them quickly or advance them by a mouse click.
Particular attention was paid to the smoothness and
uniformity of animations, to both capture student
attention and enhance the learning experience.
As originally intended, each line of sample code
displayed corresponds to some action on the screen.
Even small details, such as the lifetime of variables
within their scope, have been considered so as to
provide a completely accurate portrayal of how code
is behaving. The sample code is highlighted both by
„active line‟ and by „pass or fail‟ on conditionals,
illustrating movement inside and between loops and
clauses. Providing a mapping between individual
programming language statements and an animated
display enables the algorithm to come to life, and
greatly aids a student in transitioning from an
abstract concept to a concrete implementation.
5 CONCLUSIONS
The visualization software described in this paper
presents significant benefits for both instructors and
students. In addition to the clear animation features
of this tool, the ability to step through individual
lines of code and observe the effects on data
structures being considered is invaluable. The
expandable design of the tool provides enormous
future potential for additions of more advanced data
structures and algorithms.
This application is now entering testing in Data
Structures courses at Webster University. As part of
this phase, we intend to gather quantitative and
qualitative information from students regarding the
effectiveness of the tool, what features they find
most useful, which ones they find less useful or
could use improvement, and gather suggestions for
useful additions in future versions. The experiences
and opinions of this first group of students will be
extremely important for future development.
Based on some initial feedback, there are already
new features planned for the next release. First,
additional structures will be added (namely AVL
trees and doubly linked lists), as well as new sort
algorithms and variations on existing functions (for
example, different methods of chaining and handling
collisions in a hash table). Additionally, support for
variable levels of detail on structures is planned. For
example, a quick sort could be shown in full detail,
or in a simplified version which omits the actual
line-by-line partition code and displays only the
results of recursive partition, which could be useful
as an introduction to the algorithm.
Finally, some upgrades to the interface which are
planned include the addition of an overlay on top of
the drawing surface which will contain a “key”
illustrating the components of each visual element,
as well as support for variations in the appearance of
elements themselves. WPF's XAML-based styling
greatly simplifies altering the appearance of ele-
CSEDU 2010 - 2nd International Conference on Computer Supported Education
286