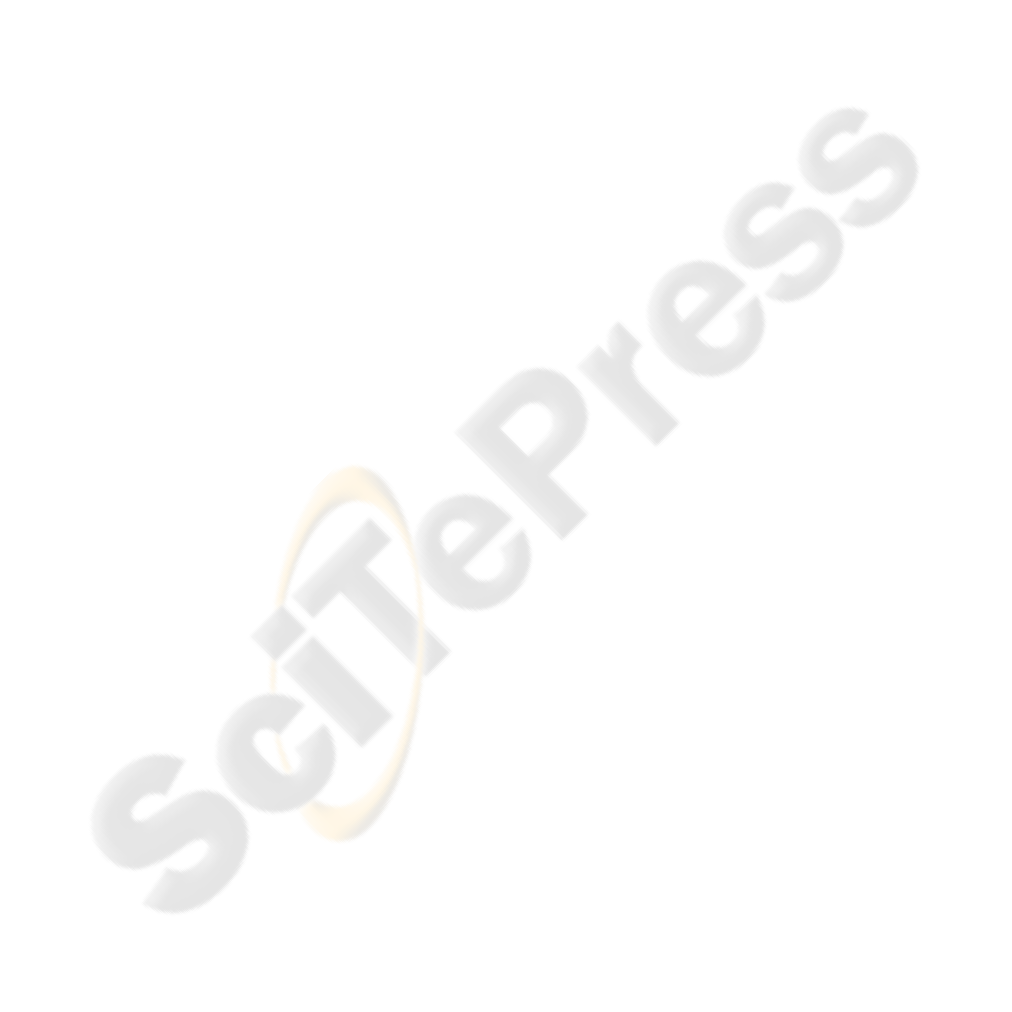
rejectedCandidates method where the
task is to “add some Candidate objects from
one ArrayList to another”, most of the
students use a Candidate parameter (Table 5).
3.2.4 Accessing a Private Field Outside its
Class
Manipulating ArrayLists involves retrieving the
objects stored in them and accessing their fields,
which should be declared private. Although this
is a common task half the students fail to access
private fields correctly outside their class. The
most common errors are:
Direct access of a private field and without an
instance (Table 3: 41%, Table 4: 47%). This
behavior might be a generalization of the fact
that students spend most of the time defining
methods that access directly the fields that are
defined in the same class. Also, this might be a
result of students’ inability to understand that
more than one object of a given class might exist
the same time and it is not enough to mention
just the name of the field we want to access.
Accessing an instance instead of its field (Table
3: 16%).
Direct access of a private field outside its class
(Table 3: 5%, Table 4: 3%).
3.2.5 Retrieving Objects from an ArrayList
Iterating and retrieving the objects stored in an
ArrayList is a typical task in applications based
on such lists. Most textbooks provide templates, but
students do not apply them correctly due to a flawed
understanding of the ArrayList concept. Students
use an Iterator object and a while loop for
iterating an ArrayList, but the following errors
are made:
A while loop is used but objects are not retrieved
(Table 4: 13%, Table 5: 11%).
The name of the ArrayList field is used as
type of the variable and casting (Table 4: 13%).
The ArrayList is not iterated (Table 4: 8%).
The retrieved object is not assigned to a
variable (Table 4 & 5: 5%).
3.2.6 Adding Objects to an ArrayList
The ArrayList class provides an add method for
adding objects to it. However, students do not
always make use of this method or do not use it
correctly (Table 3: 23%, Table 5: 19%). For
example, 1 out of 10 of the students used a statement
like:
FCE += Candidate; or FCE++;
for adding a Candidate object to an ArrayList
called FCE. Also, several students (Table 5:19%)
use a wrong argument in the add method.
4 CONCLUSIONS
Manipulating ArrayLists is a skill that all students
leaning OOP must acquire. However, this does not
seem to be so easy. The difficulties that were
recorded in the first teaching of an OOP course
(Xinogalos, Satratzemi & Dagdilelis, 2006) resulted
in a re-designed course (Xinogalos, Satratzemi &
Dagdilelis, 2007) where two lessons were devoted to
ArrayLists and lab exercises specially designed to
face these difficulties were used. The re-designed
course gave definitely better results, according to
observation during lab sessions, informal interviews
and homework assignments. However, the results of
the middle term exams showed that students still
face many difficulties. The main difficulties
(Research question 1) are:
D1. Students use a wrong return type in methods
where an ArrayList object is returned (Tables 2
& 5). Usually, the type of one of the fields of the
objects stored in the ArrayList is used as return
type. In most cases this is a primitive type or a
type considered by students as primitive (such as
String).
D2. Students use wrong parameter types in methods
manipulating ArrayLists (Tables 3 & 5). In most
cases, the type of the parameter is related to the
type of the entity (i.e. type of the field, object)
being processed in the method.
D3. When iterating an ArrayList students access
private fields of the objects retrieved directly,
without referring to an instance, or without using
accessor methods (Tables 3, 4 & 5).
D4. The size of the ArrayList object or the objects
stored in it are returned one-by-one instead of the
ArrayList object (Table 5).
D5. Students face difficulties in applying correctly
the code pattern for iterating an ArrayList and
retrieving objects (Tables 4 & 5).
D6. The add method of the ArrayList is not used, or
is used incorrectly (Tables 3 & 5).
D7. Generally, students find it difficult to
manipulate a class with fields/attributes of
ArrayList type.
The results of the study make clear that many of
the recorded difficulties are not related to ArrayLists
CSEDU 2010 - 2nd International Conference on Computer Supported Education
124