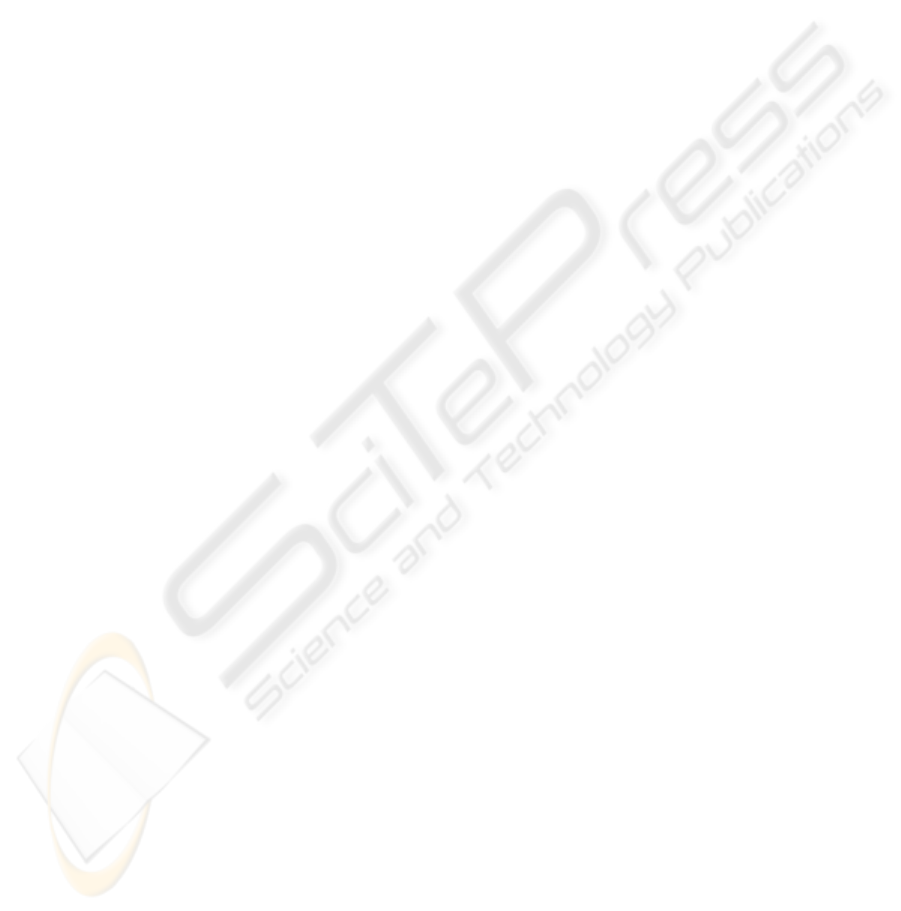
improvement versus (Lagae and Dutr
´
e, 2008), even
using worse hardware.
Estimated algorithm performance is ≈ 4.26 FPS
for the Thai Statue scene at 1024×1024 resolution on
a quad core system assuming a linear speedup, com-
mon in ray tracing. This compares well with the 3.14
FPS KD-tree performance achieved by (Shevtsov
et al., 2007) on such a system.
5 CONCLUSIONS AND FUTURE
WORK
Multi-level hashed grids have good behavior for large
scanned models, having twice the render-time perfor-
mance of one-level hashed grids, with a small penalty
in terms of build time or memory usage. They suc-
cessfully combine the better traits of classic multi-
level array grids and one-level hashed grids, manag-
ing to provide best of class performance for scanned
scenes.
There still seems to be room for improvement
in regards to speeding up grid traversal by skipping
empty cells. Possibilities include proximity clouds
(Cohen and Sheffer, 1994) and macro-regions (Dev-
illers, 1989). This work also does not employ SIMD
instructions or ray coherence. All of these techniques
have a chance of significantly improving performance
and should be worthy of further pursuit.
ACKNOWLEDGEMENTS
It would not have been possible to make the tests in
this work without the scanned models from the Stan-
ford 3D Scanning Repository. Office and Confer-
ence scenes were created by Anat Grynberg and Greg
Ward.
We would also like to thank the anonymous re-
viewers, for their comments helped improve this
work.
Supported by the Portuguese Foundation for
Science and Technology project VIZIR (PTD-
C/EIA/66655/2006).
REFERENCES
Amanatides, J. and Woo, A. (1987). A fast voxel traversal
algorithm for ray tracing. In Eurographics ’87, pages
3–10.
Cleary, J. and Wyvill, G. (1988). Analysis of an algorithm
for fast ray tracing using uniform space subdivision.
The Visual Computer, 4(2):65–83.
Cohen, D. and Sheffer, Z. (1994). Proximity clouds - an ac-
celeration technique for 3D grid traversal. The Visual
Computer, 11(1):27–38.
Devillers, O. (1989). The macro-regions: an efficient space
subdivision structure for ray tracing. In Eurographics
’89, pages 27–38.
Fujimoto, A., Tanaka, T., and Iwata, K. (1986). Arts: Ac-
celerated ray-tracing system. Computer Graphics and
Applications, IEEE, 6(4):16–26.
Havran, V., Sixta, F., and Databases, S. (1999). Comparison
of hierarchical grids. Ray Tracing News, 12(1):1–4.
Ize, T., Shirley, P., and Parker, S. (2007). Grid creation
strategies for efficient ray tracing. In Interactive Ray
Tracing, 2007. RT’07. IEEE Symposium on, pages 27–
32.
Jevans, D. and Wyvill, B. (1989). Adaptive voxel subdivi-
sion for ray tracing. In Graphics Interface ’89, pages
164–172.
Kalojanov, J. and Slusallek, P. (2009). A parallel algorithm
for construction of uniform grids. In Proceedings of
the 1st ACM conference on High Performance Graph-
ics, pages 23–28. ACM.
Kim, T., Moon, B., Kim, D., and Yoon, S. (2009). RACB-
VHs: random-accessible compressed bounding vol-
ume hierarchies. In SIGGRAPH 2009: Talks, page 46.
ACM.
Lagae, A. and Dutr
´
e, P. (2008). Compact, fast and robust
grids for ray tracing. Computer Graphics Forum (Pro-
ceedings of the 19th Eurographics Symposium on Ren-
dering), 27(8).
M
¨
oller, T. and Trumbore, B. (2005). Fast, minimum stor-
age ray/triangle intersection. In International Con-
ference on Computer Graphics and Interactive Tech-
niques. ACM Press New York, NY, USA.
Shevtsov, M., Soupikov, A., and Kapustin, A. (2007).
Highly parallel fast kd-tree construction for interactive
ray tracing of dynamic scenes. In Computer Graphics
Forum, volume 26, pages 395–404. Citeseer.
Wald, I., Ize, T., Kensler, A., Knoll, A., and Parker, S.
(2006). Ray tracing animated scenes using coher-
ent grid traversal. In International Conference on
Computer Graphics and Interactive Techniques, pages
485–493. ACM Press New York, NY, USA.
Woo, A. (1992). Ray tracing polygons using spatial subdi-
vision. In Proceedings of the conference on Graph-
ics interface ’92, pages 184–191, San Francisco, CA,
USA. Morgan Kaufmann Publishers Inc.
GRAPP 2010 - International Conference on Computer Graphics Theory and Applications
224