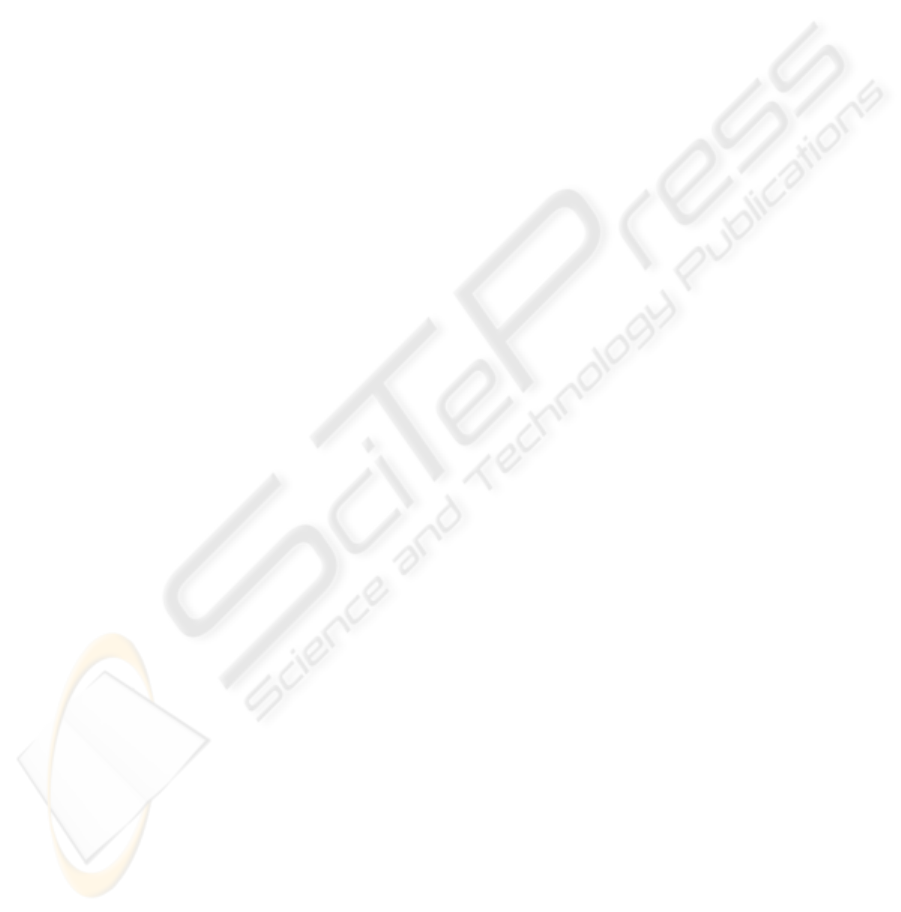
• Knowing the basics of the interoperability of
applications.
• Knowing design techniques in order to develop
business applications (especially concerning Web
applications) through a layered architecture.
3 TEACHING METHODOLOGY
In spite of having only practical credits, during the
development of the subject, we also make use of
lecture sessions. They consist of theoretical basic
concepts, necessary to carry out the practice tasks.
Thus, special emphasis is placed on database access
technologies or software patterns used for the
development of the model layers, view and
controller of business applications.
Each part of the subject involves carrying out a
practice task, to be conducted in groups. Each task
tries to mimic the generic performance of known
Web applications (Betandwin, Amazon, etc.),
although obviously with fewer features due to the
limited time available for its completion.
For each application two iterations or deadlines
are defined. In the first iteration, which does not
entail a grade, the initial part is implemented. The
objective of this first iteration is to try to guarantee
that the students focus on its development. To this
end, the teacher tries to detect significant errors, and
if there is any, the students are guided towards their
solution. In the second iteration, the students correct
the errors identified in the first one and add the other
features.
As discussed above, the second application uses
the functionality provided by the first one. In this
way, the students will be helped to go in depth into
the aspects relating to the interoperability of
applications, one of the objectives of the subject.
Due to the extent of the practice task, the
division of iterations to be handed in makes easier
that a larger number of students reach the agreed
deadlines and giving up occurs less often.
4 PROPORTIONAL
IMPLEMENTATIONS
A very important part in the progress of the subject
consists of the development and giving of a series of
examples to students. These examples allow to
observe the implementation of the theoretically
explained concepts. This makes it possible to reduce
the student's learning curve, as they can check the
concepts required subsequently to perform practice
tasks using functional examples.
The development of examples also facilitates the
practical implementation since the example code is
taken as a starting point for their progress. Thus, the
students finally develop a complex (and perfectly
usable) web application, without having to encode it
entirely.
Two complete web applications are also
provided (MiniPortal and MiniBank), in which the
emphasis is placed on the fundamentals of business
web application development. These applications
involve different aspects (layering, authentication,
transaction management, etc.) that subsequently, the
students should apply for the completion of the
practice tasks. Both applications are completed using
.NET and Java.
As follows, a brief description of each of these
materials is performed.
4.1 Tutorials
First, a series of short tutorials programs are
developed and made available to students. These
tutorials are intended to be seen as a set of examples,
each of which shows the operation or performance
of a particular aspect.
The tutorials are implemented considering they
would be used as a technology learning guide,
leaving aside issues such as efficiency or correctness
of design. This approach comes from the fact that
most of the technologies are new to the student, so
we try to provide them in a way to be understood as
far as possible. The tutorial sessions are organized as
a set of examples, each focusing on the use of a
particular aspect. All examples are thoroughly
documented in order to facilitate their assimilation.
Regarding Java technology, students are given
various examples of access to databases using
JDBC, ORM Hibernate, as well as Tapestry
framework.
Concerning the .NET technology, a similar
approach is followed. Thus, a number of examples
are given, which initially show basics of C#
programming language (structures, exception
handling, etc.). The tutorial session also includes
examples of connection to databases through
ADO.NET (on line and off line environment) and
ORM Entity Framework.
4.2 MiniPortal
As discussed above, the MiniPortal application is a
complete web application. Specifically, it is a portal
CSEDU 2010 - 2nd International Conference on Computer Supported Education
482