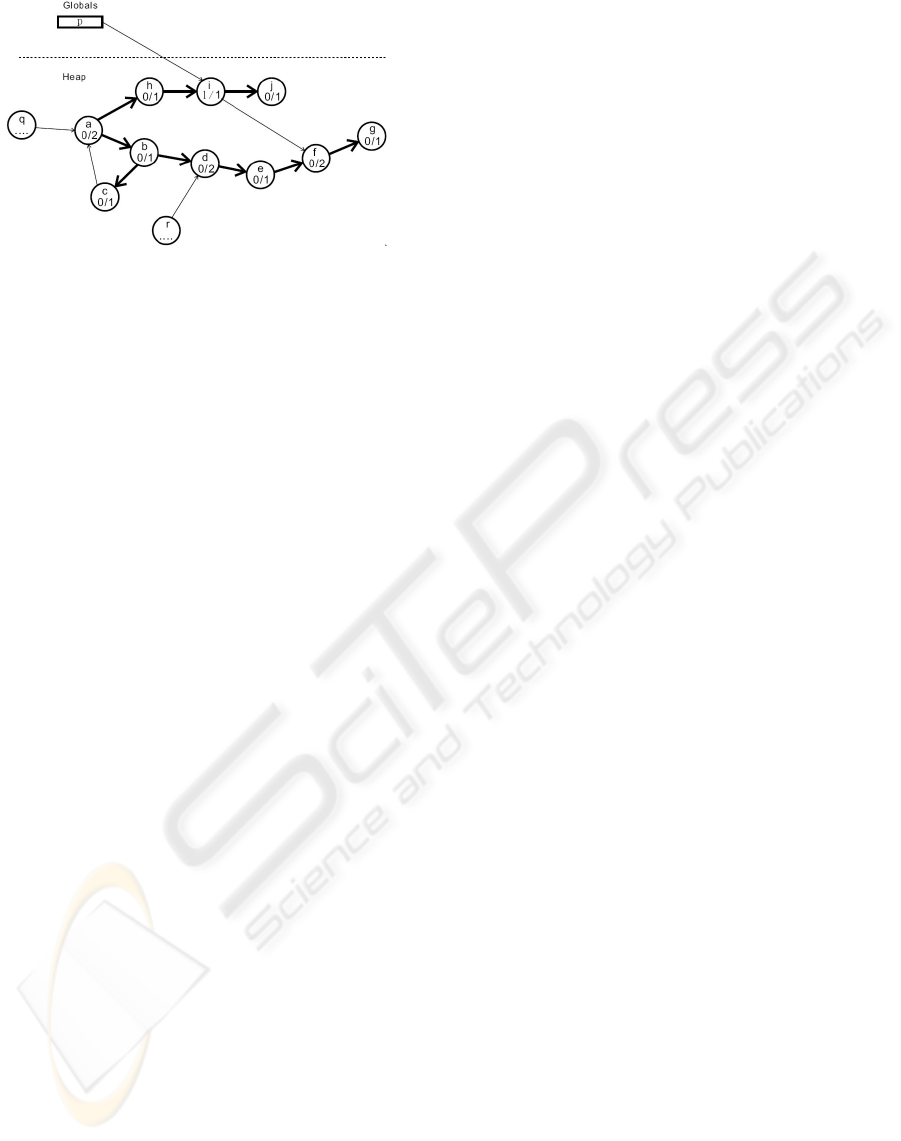
Figure 4: An example. The wide arrows form a depth-first-
search tree.
a, b, c, d, e, f , g, h (we assume that these nodes are vis-
ited in this order) as dead. When node i is visited,
d f slive(i) will be invoked since gcount(i) > 0. d f s(i)
will mark nodes i, j, f ,g as live. Note that nodes f
and g are visited in both d f sdead(a) and d f slive(i).
d f sdead and d f slive together will perform a com-
plete depth-first traversal plus some overlapped por-
tion in the span, which, in this example, contains
nodes f and g. The overlapped portion also depends
on the order nodes are visited during the d f sdead(a)
call.
4 CONCLUSIONS
We may save the root of a collection run in a buffer
and do not activate the garbage collector until a suf-
ficient number of roots have been accumulated. The
above algorithm may be adapted easily (Yang et al.,
2009).
Our new garbage-collection algorithm makes use
of two reference counters to better decide when a
node should be garbage-collected. It is better than
more aggressive algorithms by reducing the possibil-
ity of tracing live nodes and it is also better than less
aggressive algorithms because cyclic garbage is col-
lected sooner.
ACKNOWLEDGEMENTS
The work reported in this paper is partially supported
by National Science Council, Taiwan, Republic of
China, under grants NSC 96-2628-E-009-014-MY3,
NSC 98-2220-E-009-050, and NSC 98-2220-E-009-
051 and a grant from Sun Microsystems OpenSparc
Project.
REFERENCES
Bacon, D. F., Attanasio, C. R., Lee, H. B., Rajan, V. T.,
and Smith, S. (2001). Java without the coffee breaks:
A nonintrusive multiprocessor garbage collector. In
Proc. ACM SIGPLAN’01 Conf. Programming Lan-
guages Design and Implementation (PLDI).
Bacon, D. F. and Rajan, V. T. (2001). Concurrent cy-
cle collection in reference counted systems. In Proc.
15th European Conf. Object-Oriented Programming.
Springer-Verlag, LNCS 2072.
Christopher, T. W. (1984). Reference count garbage collec-
tion. Software Practice and Experience, 14(6):503–
507.
Collins, G. E. (1960). A method for overlapping and erasure
of lists. Communications of the ACM, 3(12):655–657.
Fischer, C. N. and LeBlanc, R. J. J. (1991). Crafting a Com-
piler with C. Benjamin/Cummings, MA.
Jones, R. E. and Lins, R. D. (1996). Garbage Collection
Algorithms for Dynamic Memory Management. John
Wiley and Sons, New York.
Lin, C. Y. (2009). Efficient Cyclic Garbage Reclamation
Appraoch for Reference Coounted Memory Manage-
ment Systems, Ph.D. Dissertation. National Cheng-
Kung University, Tainan, Taiwan, R.O.C.
Lin, C. Y. and Hou, T. W. (2006). A lightweight cyclic
reference counting algorithm. In Proc. Interna-
tional Conf. Grid and Pervasive Computing. Springer-
Verlag, LNCS 3947.
Lin, C. Y. and Hou, T. W. (2007). A simple and efficient
algorithm for cycle collection. ACM Sigplan Notices,
42(3):7–13.
Lins, R. D. (1992). Cyclic reference counting with
lazy mark-scan. Information Processing Letters,
44(4):215–220.
Lins, R. D., de Carvalho Junior, F. H., and Lins, Z. D.
(2007). Cyclic reference counting with permanent
objects. Journal of Universal Computer Science,
13(6):830–838.
Martinez, A. D., Wachenhauzer, R., and Lins, R. D. (1990).
Cyclic reference counting with local mark-scan. In-
formation Processing Letters, 34(1):31–35.
Yang, W., Tseng, H. R., and Jan, R. H. (2009). Identifying
closed clusters in the heap. Submitted for publication.
ICSOFT 2010 - 5th International Conference on Software and Data Technologies
270