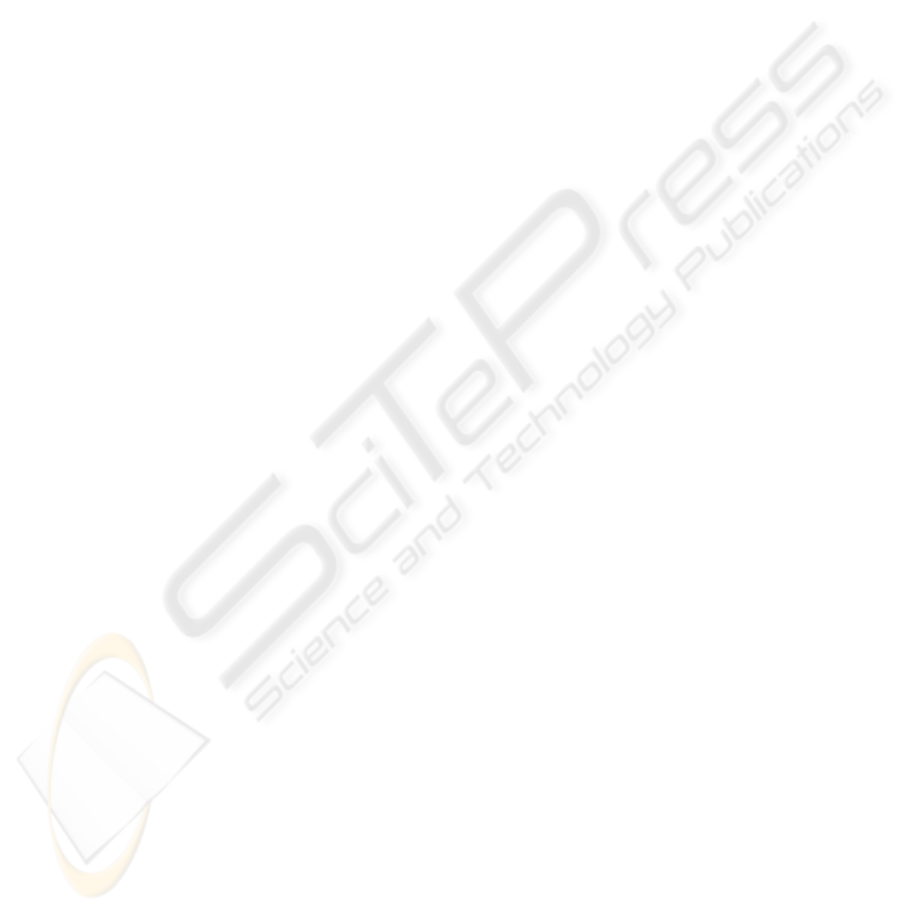
REFERENCES
Booch, G., Maksimchuk, R., Engle, M., Young, B., Con
allen, J., Houston, K. 2007. Object Oriented Design
with Applications, Addison Wesley Professional.
Brittanica, E. 1986. Encyclopaedia Brittanica, Chicago,
IL, USA, Encyclopaedia Brittanica.
Coad, P., Yourdon, E. 1991. Object Oriented Design,
Upper Saddle River, NJ, USA, Yourdon Press.
Eckel, B., Sysop, Z. F. 1998. Thinking in Java, Upper
Saddle River, NJ, USA, Prentice Hall PTR.
Fowler, M. 2004. Inversion of Control Containers and the
Dependency Injection Pattern [Online]. [Accessed Jan
2010].
Goldberg, A., Robson, D. 1989. Smalltalk-80: The Lan-
guage, Boston, MA, USA, Addison Wesley Longman.
Hejlsberg, A., Torgersen, M., Wiltamuth, S., Golde, P.
2008. The C# Programming Language, Addison
Wesley Professional.
Holub, A. 2003. Why Extends Is Evil. Java World.
Hunt, A., Thomas, D. 1998. Tell, Don't Ask [Online].
Available: http://www.pragprog.com/articles/tell-dont-
ask [Accessed].
Irwin, W. 2007. Understanding and Improving Object-
Oriented Software through Static Software Analysis.
Ph.D., University of Canterbury.
Irwin, W., Churcher, N. I. 2003. Object Oriented Metrics:
Precision Tools and Configurable Visualisations. In:
METRICS2003: 9th IEEE Symposium on Software
Metrics, Sep 2003 Sydney, Australia. 112-123.
Irwin, W., Cook, C., Churcher, N. I. 2005. Parsing and
Semantic Modelling for Software Engineering
Applications. In: Strooper, P., ed. Australian Software
Engineering Conference, Mar 2005 Brisbane,
Australia. IEEE Press, 180-189.
Lieberherr, K., Holland, I. 1989. Assuring Good Style for
Object-Oriented Programs. IEEE Software, 6, 38-48.
Martin, R. C. 1997. Stability. C++ Report.
Meyer, B. 1988. Object-Oriented Software Construction,
New York, Prentice-Hall.
Parnas, D. L. 1972. On the Criteria to Be Used in
Decomposing Systems into Modules. Communications
of the ACM, 15, 1053 - 1058.
Qualitas_Research_Group. 2009. Qualitas Corpus Version
20090202 [Online]. Available: http://www.
cs.auckland.ac.nz/~ewan/corpus [Accessed 2009].
Riel, A. J. 1996. Object-Oriented Design Heuristics,
Reading, Mass., Addison-Wesley.
Rogers, P. 2001. Encapsulation Is Not Information Hiding.
Java World. http://www.javaworld/javaworld/jw-05-
2001/jw-0518-encapsulation.html
Snyder, A. Year. Encapsulation and Inheritance in Object-
Oriented Programming Languages. In: Object-
oriented programming systems, languages and
applications, 1986. 38-45.
Stroustrup, B. 1994. The Design and Evolution of C++,
ACM Press/Addison-Wesley Publishing Co.
Stroustrup, B. 1997. The C++ Programming Language,
Boston, MA, USA, Addison Wesley Longman.
Tempero, E. D. Year. How Fields Are Used in Java: An
Empirical Study. In: Australian Software Engineering
Conference, Apr 2009 2009 Gold Coast, Australia.
IEEE Computer Society, 91-100.
Voigt, J., Irwin, W., Churcher, N. I. 2009. Intuitiveness of
Class and Object Encapsulation. 6th International
Conference on Information Technology and
Applications. Hanoi, Vietnam.
Voigt, J., Irwin, W., Churcher, N. I. 2010. Technical
Report Tr-Cosc 01/10: List of Qualitas Code Corpus
Programs Used for Encapsulation Research [Online].
Christchurch, New Zealand: University of Canterbury.
Available: http://www.cosc.canterbury.ac.nz/research/
reports/TechReps/2010/tr_1001.pdf [Accessed].
Yourdon, E., Constantine, L. 1979. Structured Design:
Fundamentals of a Discipline of Computer Program
and Systems Design, Englewood Cliffs, N. J., Prentice
Hall.
ENASE 2010 - International Conference on Evaluation of Novel Approaches to Software Engineering
178