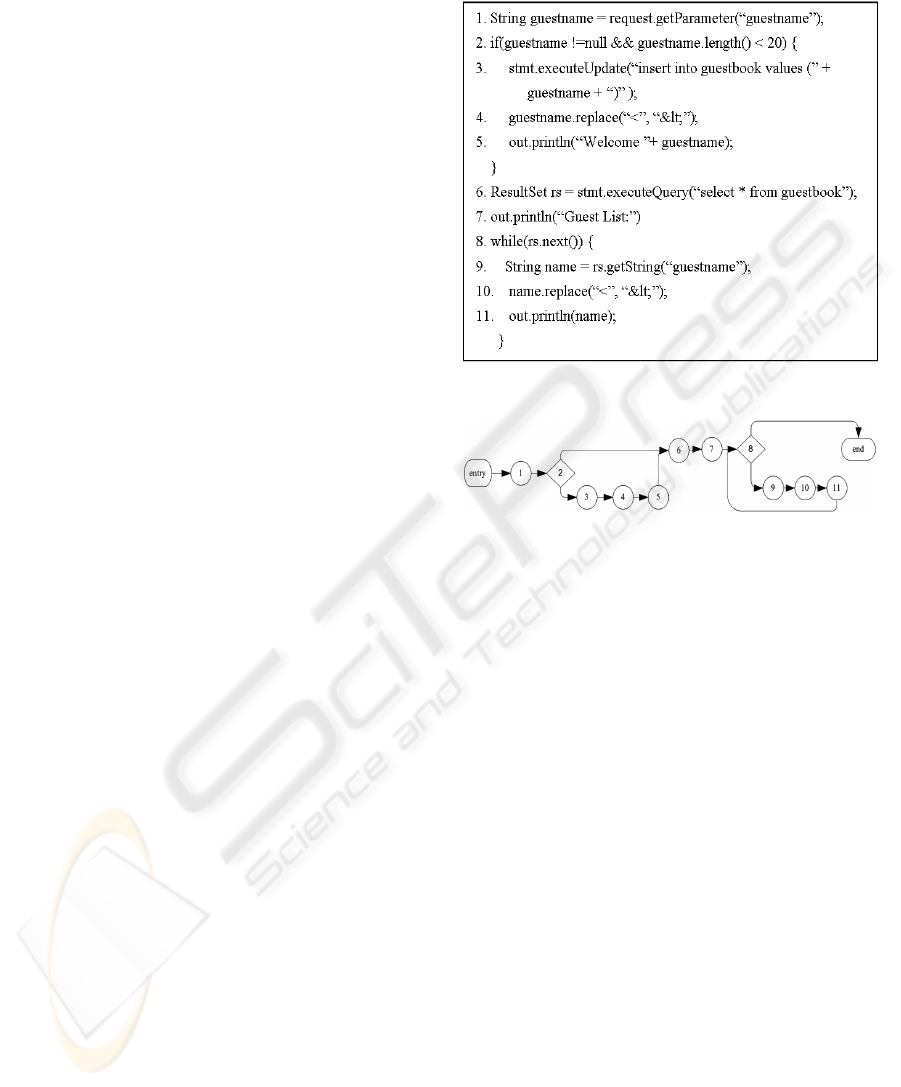
Property 1 – Unprotected Html-O-Node. If there
is no input validation node for k such that both
transitively reference to a common input variable v
of an input node u, then k is unprotected from XSS
attack through illegal manipulation of v.
A path through a CFG is called a 1-path if it
does not repeat any loop. Let Ω be the set of 1-paths
through the CFG. The partition of 1-paths in Ω, such
that paths which contain the same set of iv-nodes for
k and follow the same branch at each of these nodes
are put in the same class, is called the input
validation partition (iv-partition) for k. In the
CFG shown in Figure 2, {(entry, 1, 2, 3, 4, 5, 6, 7, 8,
9, 10, 11, 8, end), (entry, 1, 2, 3, 4, 5, 6, 7, 8, end),
(entry, 1, 2, 6, 7, 8, 9, 10, 11, 8, end), (entry, 1, 2, 6,
7, 8, end)} is the set of 1-paths. Hence, in this CFG,
{{(entry, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 8, end),
(entry, 1, 2, 3, 4, 5, 6, 7, 8, end)}, {(entry, 1, 2, 6, 7,
8, 9, 10, 11, 8, end), (entry, 1, 2, 6, 7, 8, end)}} is
the iv-partition for the html-o-node, node 5; and
{{(entry, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 8, end),
(entry, 1, 2, 6, 7, 8, 9, 10, 11, 8, end)}, {(entry, 1, 2,
3, 4, 5, 6, 7, 8, end), (entry, 1, 2, 6, 7, 8, end)}} is
the iv-partition for the html-o-node, node 11.
Next, we shall formalize the computation of
valid and invalid conditions for a html-o-node in
Property 2 and 3, which can be proved directly from
the definitions of input validation node and input
validation partition.
Property 2 – Invalid Input Condition. IVC
k
= {C
⏐ X is a class in the iv-partition of k such that there
is a path in X which passes through some iv-nodes
for k but does not pass through k; and C = the
conjunction of all the branch conditions of branches
at iv-nodes for k that any path in X follows} is the
set of invalid input conditions for k.
Property 3 – Valid Input Condition. VC
k
= {C ⏐
X is a class in the iv-partition of k such that there is
a path in X which passes through some iv-nodes for
k and also passes through k; and C = the conjunction
of all the branch conditions of branches at iv-nodes
for k that any path in X follows} is the set of valid
input conditions for k.
Note that for each class X stated in Property 2
and 3, the conjunction of all the branch conditions of
branches at iv-nodes for k that any path in X follows
is identical.
From the iv-partition computed for node 5, we
compute according to Property 2 and 3 that IVC = {(
!(guestname!=null && guestname.length()<20))}
and VC={(guestname!= null && guestname.length()
<20)} are the sets of invalid and valid input
conditions for this html-o-node. IVC and VC for the
html-o-node, node 11 can also be computed in a
similar way.
Figure 1: JSP code snippet for Guestbook SignIn.
Figure 2: The CFG of the JSP code snippet.
2.2 Extraction of Defense through
Input Filtering
For defending against XSS attack through a html-o-
statement, another alternative is to remove, replace,
or escape any dangerous characters from inputs that
may define the values of pv-variables referenced at
this html-o-statement. This filtering approach is
generally carried out by a sequence of statements
that may influence the values of all pv-variables of a
html-o-statement. We shall formalize a terminology
to characterize such coding pattern.
Potentially Vulnerable Input Filter (PV-Input
Filter) for a html-o-statement k in a program P is a
sequence F of following statements according to
their order in P:
1) All the statements at which a pv-variable of k is
defined/submitted.
2) Statements on which statements in F are data
dependent.
3) Statements on which statements in F are control
dependent such that k is not transitively control
dependent on these statements.
For example, the sequence of following statements
forms the pv-input filter for the html-o-statement at
line 5 shown in Figure 1:
AUDITING THE DEFENSE AGAINST CROSS SITE SCRIPTING IN WEB APPLICATIONS
507