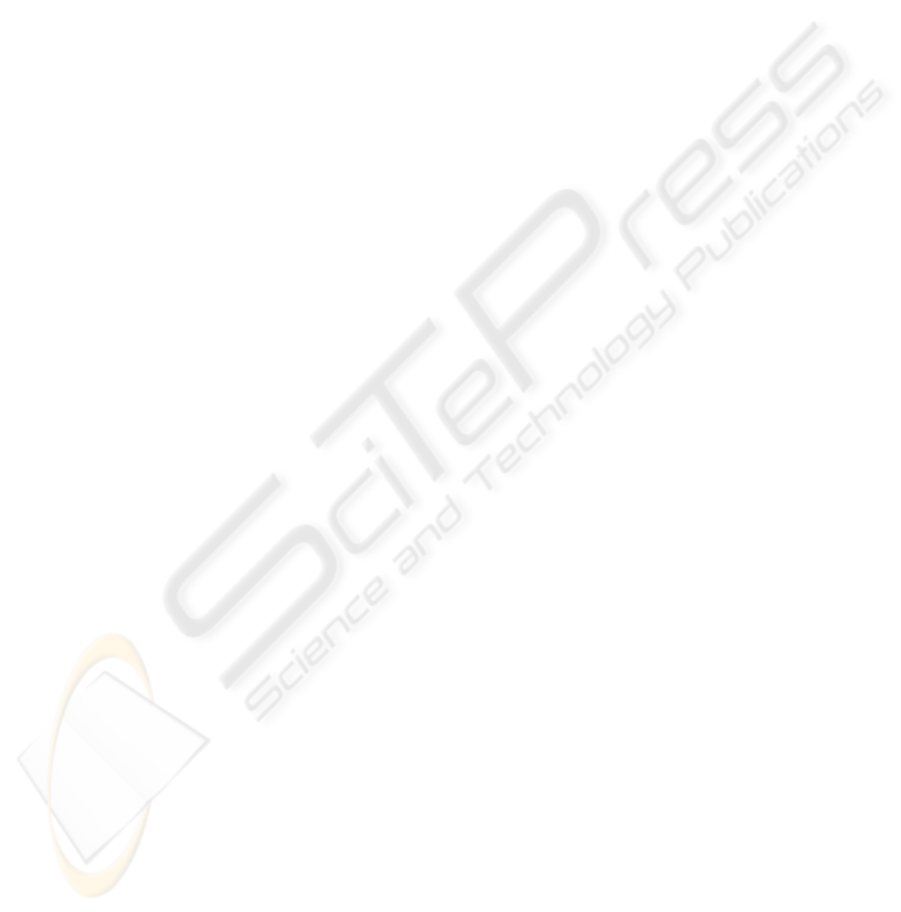
previous sections, the computation is less energy
demanding than communication and thus
computation and communication activity must be
organized together.
Many authors have proposed languages and
middleware for WSN programming, most of them
use nesC (Gay et al. 2003) as C dialect for node
level programming with execution of native code in
the motes, while other systems like Maté (Levis &
Culler 2002) use a virtual machine approach.
For the systems cited above high-level, global
behaviour must be expressed in terms of complex,
local actions taken at each node. To address this
issue different network-oriented programming
models have been proposed. One of the most
assessed strategies is to consider the whole WSN as
a distributed database to be queried with SQL
expressions (Madden et al. 2005) or XML
expressions. This approaches are not well-suited for
developers who wish to implement specific
behaviour at a lower level than the query interface.
Another promising approach is the so-called macro-
programming. In Regiment (Newton et al. 2007) a
global defined logic is automatically de-globalized
into pieces of code at node level. The Regiment
syntax is inspired by ML(Milner 1997) while
PySense is based on Python. Another macro-
programming language based on Python is Kairos
(Gummadi et al. 2005) in which the programmer is
presented with three constructs: reading and writing
variables at nodes, iterating through the one-hop
neighbours of a node, and naming and addressing
arbitrary nodes. Kairos language is an extension of
the Python language while PySense is a hosted
language on top of Python decorators and relies on
the runtime deployment of code on mobile Python
interpreters. Kairos authors have apparently
abandoned the project and have recently proposed
Pleiades(Kothari et al. 2007) , an extension of C
language with dynamic code partitioning and
migration.
5 CONCLUSIONS AND FUTURE
WORK
We consider useful developing a new tool in this
area given the huge potential of WSNs as
technology and the easiness to learn Python as
programming language even for application domain
experts.
At the time of writing, PySense is still
incomplete to be used in real world applications and
its development is ongoing. The BRE is almost
completely implemented while the set of RRE is at
the moment emulated by a network server that
emulates the reception of requests, the transmissions
of responses, and the computation of migrated code.
The real RRE is under development and based
on the Pymite VM developed in the Python-on-a-
chip project (python-on-a-chip). Thus, the current
commitment for the PySense project is now to fulfil
the design depicted in the paper with a complete
implementation to be tested with real world
applications.
REFERENCES
python-on-a-chip - Project Hosting on Google Code.
http://code.google.com/p/python-on-a-chip/
Elrad, T., Filman, R.E. & Bader, A., 2001. Aspect-
oriented programming: Introduction. Communications
of the ACM, 44(10), 29–32.
Gay, D. et al., 2003. The nesC language: A holistic
approach to networked embedded systems. In
Proceedings of the ACM SIGPLAN 2003 conference
on Programming language design and
implementation. pag. 11.
Gummadi, R., Gnawali, O. & Govindan, R., 2005. Macro-
programming wireless sensor networks using kairos.
Lecture Notes in Computer Science, 3560, 126–140.
Heinzelman, W.B. et al., 2002. An application-specific
protocol architecture for wireless microsensor
networks. IEEE Transactions on wireless
communications, 1(4), 660–670.
Kothari, N. et al., 2007. Reliable and efficient
programming abstractions for wireless sensor
networks. In Proceedings of the 2007 ACM SIGPLAN
conference on Programming language design and
implementation. pag. 210.
Levis, P. & Culler, D., 2002. Mate: A tiny virtual machine
for sensor networks. ACM SIGARCH Computer
Architecture News, 30(5), 95.
Madden, S.R. et al., 2005. TinyDB: an acquisitional query
processing system for sensor networks. ACM
Transactions on Database Systems (TODS), 30(1),
173.
Milner, R., 1997. The definition of standard ML, MIT
Press.
Newton, R., Morrisett, G. & Welsh, M., 2007. The
regiment macroprogramming system. In Proceedings
of the 6th international conference on Information
processing in sensor networks. pag. 498.
Pottie, G.J. & Kaiser, W.J., 2000. Wireless integrated
network sensors. Communications of the ACM, 43(5),
51–58.
Younis, O., Krunz, M. & Ramasubramanian, S., 2006.
Node clustering in wireless sensor networks: Recent
developments and deployment challenges. IEEE
Network, 20(3), 20–25.
PYSENSE: PYTHON DECORATORS FOR WIRELESS SENSOR MACROPROGRAMMING
169