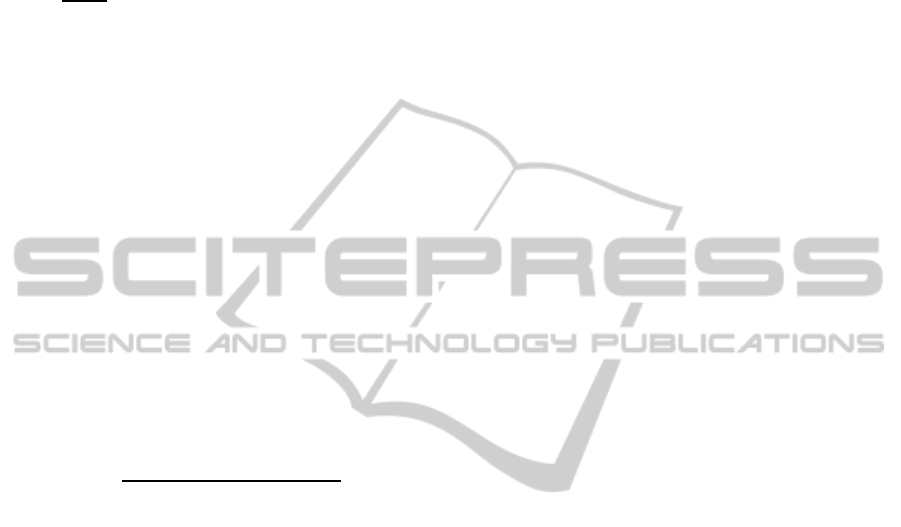
the length metric λ. The second metric is the invari-
ance metric
ι( f) =
1+
m
∑
i=1
f
2
(x
i,1
,. .. ,x
i,m
)
!
−1
.
However, these two metrics are not enough. The ex-
pression
1
2
100
+x
is almost invariant on small x, how-
ever this expression is not acceptable. The solution is
introducing the tautology metric
τ( f) = 1−
1+
k
∑
i=1
f
2
(y
i,1
,. .. ,y
i,m
)
!
−1
,
where y
i, j
are random numbers. Typical weights of
metrics are w
ι
= 1, w
τ
= 1 and w
λ
= 0.1.
In classification problem we are given the set
{(x
i,1
,x
i,2
,. .. ,x
i,m
,c
i
) : i = 1,.. .,n}, where c
i
is a
Boolean value indicating whether the corresponded
tuple belongs to a class. We need to use the rules for
floating point type and associated operators’ domains;
rules to support Boolean type (defined by items T1–
T3 in the section 2); rules to support relation operators
<, >, = (only D1 and D2, because these operators do
not preserve the operands’ types); rules for operators
∨, ∧, ¬ (D1–D4).
The fitness metric is adjusted as follows
σ(g) =
1+
|{i : g(x
i,1
,. .. ,x
i,m
) = c
i
}|
n
−1
.
4 CONCLUSIONS AND FUTURE
WORK
We have proposed a methodology of genetic pro-
gramming algorithm that embeds the features of
symbolic computations. This approach was imple-
mented in .NET library. We have supported alge-
braic, trigonometric and comparison operations with
floating-points numbers, as well as logical operations
with Boolean values. Using the library, we were able
to solve the different data mining problems: function
approximation, invariants finding and classification.
Our future research will be conducted in the fol-
lowing directions:
• Finding the parameters that provide the most ef-
ficient GP performance. By our observation,
changing of rules’ and metrics’ weights leads to
significant changes in performance. Moreover,
changing the parameters during the algorithm’s
work has different effect depending on the current
state of the population. We believe that the thor-
ough examination of such effects can lead to sig-
nificant improvements in genetic programming.
• Using genetic programming in new domains:
fuzzy numbers, fuzzy logic, temporal logic, etc.
• Exploring substitutions for length metric. Length
metric does not seem to catch the intuitive mean-
ing of a “good” expression. We plan to introduce
computation complexityand aesthetics metrics in-
stead, and understand how it improves the work of
the algorithm.
ACKNOWLEDGEMENTS
The work is supported by the Russian Federation
President’s program MK-844.2011.1.
REFERENCES
Drayton, P., Albahari, B., and Neward, T. (2002). C# in a
Nutshell. O’Reilly.
Goldberg, D. (1986). Genetic Algorithms in Search, Opti-
mization, and Machine Learning. Addison-Wesley.
Kinzett, D., Johnston, M., and Zhang, M. (2008). Numer-
ical simplification for bloat control and analysis of
building blocks in genetic programming. Evolution-
ary Intelligence, 4.
Koza, J. R. (1992). Genetic programming: on the program-
ming of computers by means of natural selection. MIT
Press, Cambridge, MA.
Koza, J. R. (1994). Genetic programming for economic
modeling. In Intelligent Systems for Finance and
Business.
Mori, N., McKay, B., Hoai, N. X., Essam, D., and Takeuchi,
S. (2009). A new method for simplifying algebraic
expressions in genetic programming called equiva-
lent decision simplification. Journal of Advanced
Computational Intelligence and Intelligent Informat-
ics, 13(14):237–238.
Poli, R., Langdon, W. B., McPhee, N. F., and Koza, J. R.
(2008). A Field Guide to Genetic Programming.
Robertson, A. P. and Dumont, C. (2002). Design of robot
calibration models using genetic programming. In
Mayorga, R. V. and Rios, A. S.-D. L., editors, Pro-
ceedings of the Third International Symposium on
Rob. and Autom., volume 3, pages 449–454.
Schmidt, M. and Lipson, H. (2009). Distilling free-
form natural laws from experimental data. Science,
324(5923):81–85.
Zhang, M. and Wong, P. (2008). Genetic programming
for medical classification: a program simplification
approach. Genetic Programming and Evolvable Ma-
chines, 9(2):229–255.
Zhang, M., Wong, P., and Qian, D. (2006). Online pro-
gram simplification in genetic programming. Simu-
lated Evolution and Learning - SEAL, pages 592–600.
GENETIC PROGRAMMING WITH EMBEDDED FEATURES OF SYMBOLIC COMPUTATIONS
479