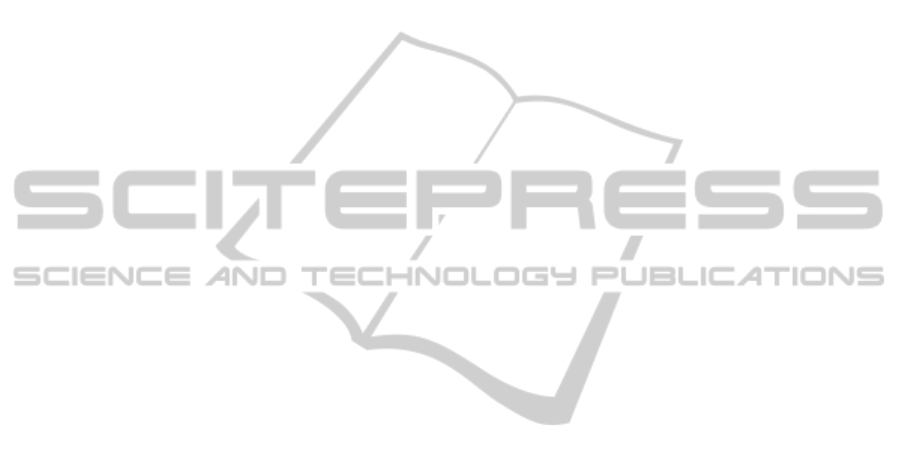
version, COP-II (Savinov, 2008; Savinov, 2009),
changes the interpretation of concepts and adds
several new mechanisms. This paper describes a
new major revision of concept-oriented
programming, denoted as COP-III. Its main goal is
to describe this programming model by using fewer
general notions and more natural interpretations by
simultaneously covering more programming patterns
existing in other approaches.
The first major change in COP-III is that
concepts are defined differently: instead of using
two symmetric constituents – object class and
reference class – we use only one component which
models references. Instead of modeling objects
explicitly via object classes, we propose a new
general treatment of objects: object is a function of
its reference.
Another important change is the use of two
keywords for navigating through the hierarchy,
super and sub (as opposed to using only super in
OOP), and the existence of two opposite overriding
strategies. In addition, COP-III introduces incoming
and outgoing methods instead of using reference
methods and object methods in previous versions.
Incoming methods of concepts intercept requests
from outside and outgoing methods intercept
requests from inside. We also remove the
continuation method and reference resolution
mechanism from the programming model. Instead,
access indirection relies on the ability of elements to
intercept incoming and outgoing methods.
The paper has the following layout. Section 2
defines the notion of concept. Section 3 is devoted to
describing inclusion relation. Section 4 describes
how inheritance, polymorphism and cross-cutting
concerns are implemented in COP-III using concepts
and inclusion. Section 5 makes concluding remarks.
2 CONCEPTS INSTEAD OF
CLASSES
Concepts and values. Concepts in COP-III describe
values. In this sense, concepts are analogous to
classes in C++ except that concepts do not have a
possibility to get an address or reference for their
instances. Like all values, concept instances are
passed by-copy only and do not have any permanent
location, address, pointer, reference or any other
indirect representation. For example, the following
concept describes a bank account:
concept Account {
char[10] accNo;
Person owner;
}
The first field will contain 10 characters while the
second field will contain a value with the structure
defined by the Person concept.
Dual methods. What makes concepts different
from classes is the presence of two kinds of
methods: incoming methods (marked by the
modifier ‘in’) and outgoing methods (marked by the
modifier ‘out’). Such a pair of incoming and
outgoing methods with the same signature is referred
to as dual methods. For example, if we would like to
have a method for getting the current account
balance then formally this functionality can be
specified in the incoming and outgoing methods:
concept Account
char[10] accNo;
in double getBalance() {...};
out double getBalance() {...};
}
It is not necessary to define both versions: if one of
them is absent then it is supposed to have a default
implementation. Dual methods are invoked as usual
using only their name without any indication if it is
an incoming or outgoing version. The main purpose
of dual methods is performing different functions for
different directions of access. If concepts are thought
of as borders then dual methods are responsible for
processing incoming and outgoing requests. In other
words, a request originating from inside is processed
by an outgoing method and a request originating
from outside is processed by an incoming method.
Scopes and directions of access are described in
Section 3.
References and objects. One of the most
important assumptions in COP is that references are
values and hence modeling the structure of
references is equivalent to modeling that of values.
More specifically, references are values interpreted
as locations or addresses of objects. References not
only identify objects but also provide access to other
values which are thought of as being stored in the
object fields. Thus object fields can be defined as
functions of references which return the same output
value for the same input reference (but this
association may change by using setters). An object
is defined as a couple of two tuples: the first tuple,
called reference or identity, is a number of values
, and the second tuple, called object
or entity, is a number of values returned by functions
defined on the reference (first tuple),
. Thus an object is identified by its
ICSOFT 2012 - 7th International Conference on Software Paradigm Trends
382