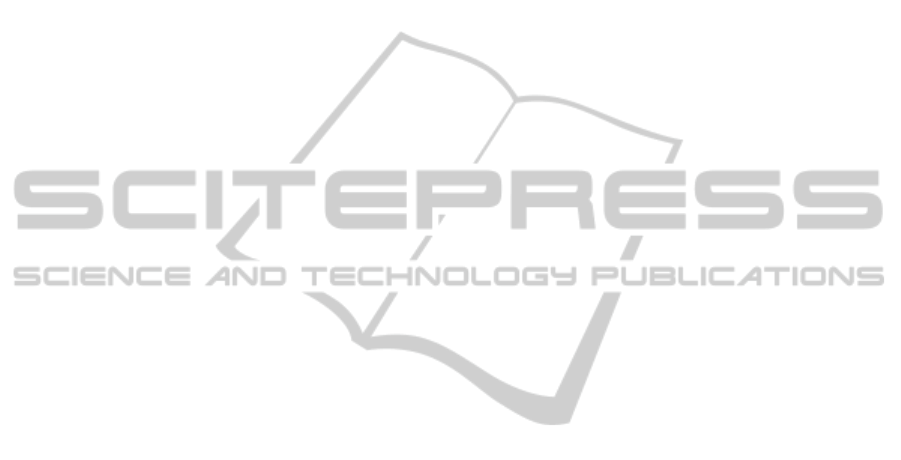
Additionally, greater code optimization (e.g. use of
SIMD instructions) could also improve performance.
Finally, one of FlexRender’s strengths is that it
decouples the ray tracing computations from shading
with a feed-forward design that requires no replies.
Because messages are both asynchronous and never
return, this opens up the possibility for batching com-
putation to specialized hardware coprocessors, such
as GPUs or the upcoming Intel MIC cards.
REFERENCES
´
Afra, A. (2012). Interactive ray tracing of large models
using voxel hierarchies. Computer Graphics Forum,
31(1):75–88.
Badouel, D., Bouatouch, K., and Priol, T. (1994). Distribut-
ing data and control for ray tracing in parallel. Com-
puter Graphics and Applications, IEEE, 14(4):69 –77.
Cignoni, P., Montani, C., Scopigno, R., and Rocchini, C.
(1998). A general method for preserving attribute
values on simplified meshes. In Proceedings of the
conference on Visualization ’98, VIS ’98, pages 59–
66, Los Alamitos, CA, USA. IEEE Computer Society
Press.
Clark, J. H. (1976). Hierarchical geometric models for
visible-surface algorithms. In Proceedings of the 3rd
annual conference on Computer graphics and inter-
active techniques, SIGGRAPH ’76, pages 267–267,
New York, NY, USA. ACM.
Cohen, J., Olano, M., and Manocha, D. (1998).
Appearance-preserving simplification. In Proceedings
of the 25th annual conference on Computer graph-
ics and interactive techniques, SIGGRAPH ’98, pages
115–122, New York, NY, USA. ACM.
Dean, J. and Ghemawat, S. (2004). Mapreduce: simplified
data processing on large clusters. In Proceedings of
the 6th conference on Symposium on Opearting Sys-
tems Design & Implementation - Volume 6, OSDI’04,
pages 10–10, Berkeley, CA, USA. USENIX Associa-
tion.
DeMarle, D. E., Gribble, C. P., and Parker, S. G. (2004).
Memory-savvy distributed interactive ray tracing. In
Proceedings of the 5th Eurographics conference on
Parallel Graphics and Visualization, EG PGV’04,
pages 93–100, Aire-la-Ville, Switzerland, Switzer-
land. Eurographics Association.
Djeu, P., Hunt, W., Wang, R., Elhassan, I., Stoll, G., and
Mark, W. R. (2011). Razor: An architecture for
dynamic multiresolution ray tracing. ACM Trans.
Graph., 30(5):115:1–115:26.
Garanzha, K., Bely, A., Premoze, S., and Galaktionov,
V. (2011). Out-of-core gpu ray tracing of complex
scenes. In ACM SIGGRAPH 2011 Talks, SIGGRAPH
’11, pages 21:1–21:1, New York, NY, USA. ACM.
Hapala, M., Davidovic, T., Wald, I., Havran, V., and
Slusallek, P. (2011). Efficient stack-less bvh traversal
for ray tracing. In 27th Spring Conference on Com-
puter Graphics, SCCG ’11.
Kato, T. and Saito, J. (2002). ”kilauea”: parallel global
illumination renderer. In Proceedings of the Fourth
Eurographics Workshop on Parallel Graphics and Vi-
sualization, EGPGV ’02, pages 7–16, Aire-la-Ville,
Switzerland, Switzerland. Eurographics Association.
Kontkanen, J., Tabellion, E., and Overbeck, R. S. (2011).
Coherent out-of-core point-based global illumination.
In Eurographics Symposium on Rendering.
Krishnamurthy, V. and Levoy, M. (1996). Fitting smooth
surfaces to dense polygon meshes. In Proceedings
of the 23rd annual conference on Computer graph-
ics and interactive techniques, SIGGRAPH ’96, pages
313–324, New York, NY, USA. ACM.
Moon, B., Byun, Y., Kim, T.-J., Claudio, P., Kim, H.-
S., Ban, Y.-J., Nam, S. W., and Yoon, S.-E. (2010).
Cache-oblivious ray reordering. ACM Trans. Graph.,
29(3):28:1–28:10.
Navr
´
atil, P. A., Fussell, D. S., Lin, C., and Childs,
H. (2012). Dynamic scheduling for large-scale
distributed-memory ray tracing. In Proceedings of Eu-
rographics Symposium on Parallel Graphics and Visu-
alization, pages 61–70.
Northam, L. and Smits, R. (2011). Hort: Hadoop online
ray tracing with mapreduce. In ACM SIGGRAPH
2011 Posters, SIGGRAPH ’11, pages 22:1–22:1, New
York, NY, USA. ACM.
Pantaleoni, J., Fascione, L., Hill, M., and Aila, T. (2010).
Pantaray: fast ray-traced occlusion caching of mas-
sive scenes. In ACM SIGGRAPH 2010 papers, SIG-
GRAPH ’10, pages 37:1–37:10, New York, NY, USA.
ACM.
Reinhard, E., Chalmers, A., and Jansen, F. W. (1999). Hy-
brid scheduling for parallel rendering using coherent
ray tasks. In Proceedings Parallel Visualization and
Graphics Symposium, pages 21–28.
GRAPP2013-InternationalConferenceonComputerGraphicsTheoryandApplications
164