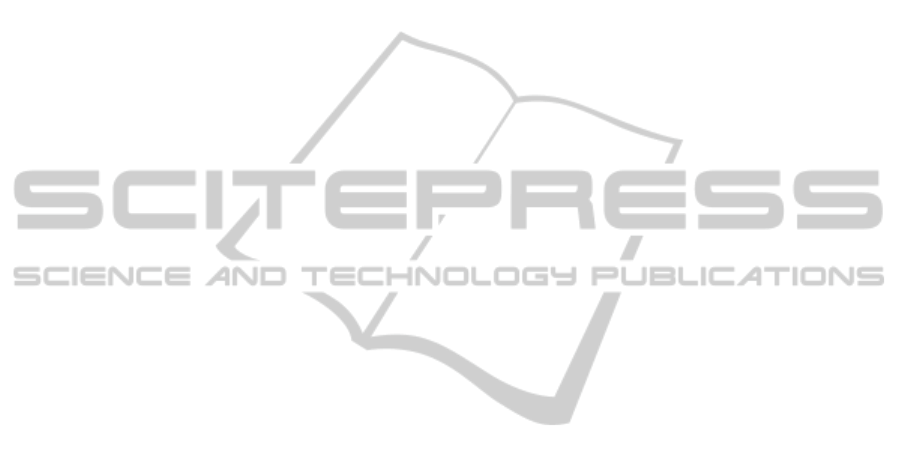
Bracha, G. and Cook, W. (1990): Mixin-Based
Inheritance. In Proceedings of the OOPSLA/ECOOP,
pages 303–311, Ottawa, Canada. ACM Press.
Ceccato, M., Marin, M., Mens, K., Moonen, L, Tonella, P.
and Tourwe, T (2005). A qualitative comparison of
three aspect mining techniques, Proc. of the Inter.
Workshop on Program Comprehension, Washington.
Cutsem, T., Bergel, A., Ducasse, S. and Meuter, W.
(2009): Adding State and Visibility Control to Traits
Using Lexical Nesting, Proc. of ECOOP 2009, Italy.
Ducasse, S., Schaerli, N., Nierstrasz, O., Wuyts, R. and
Black, A. (2004): Traits: A mechanism for fine-
grained reuse. In Transactions on Programming
Languages and Systems.
Ducasse, S., Wuyts, R., Bergel, A., and Nierstrasz, O.
(2007): User-changeable visibility: Resolving
unanticipated name clashes in traits. In Proceedings
OOPSLA, New York, NY.
Fanta, R., Rajlich, V. (1999): Removing Clones from the
Code. Journal of Software Maintenance: Research and
Practice, Volume 11(4):223-243.
Flatt, M. and Felleisen, M. (1998) Units: Cool modules for
HOT languages. In Proc. of PLDI, May 1998.
Fowler, M., (1999), Refactoring: Improving the design of
existing code, Addison-Wesley, Boston, MA, USA.
Gamma, E., Helm, R., Johnson, R. and Vlissides, J.,
(1995): Design Patterns: Elements of Reusable Object-
Oriented Software, Addison-Wesley.
Griswold, W.G., Sullivan, K., Song, Y., Shonle, M.,
Tewari, N., Cai, Y., Rajan, H., 2006: Modular
Software Design with Crosscutting Interfaces. IEEE
Software 23(1), 51–60 (2006).
Herrmann, S., (2005): Programming with Roles in
ObjectTeams/Java. AAAI Fall Symposium: "Roles, An
Interdisciplinary Perspective".
Higo, Y., Kamiya, T., Kusumoto, S., Inoue. K. (2004):
Refactoring Support Based on Code Clone Analysis.
In Proceedings of the 5th International Conference on
Product Focused Software Process Improvement
(PROFES'04), Kansai Science City, Japan.
Kamiya, T., Kusumoto, S. and Inoue, K. (2002), Ccfinder:
a multilinguistic tokenbased code clone detection
system for large scale source code, IEEE Trans. Softw.
Eng. 28, no. 7.
Kästner, C., Apel, S., Batory, D., 2007: A Case Study
Implementing Features using AspectJ. In: 11th Inter.
Conference of Software Product Line, Kyoto, Japan.
Kiczales, G., Hilsdale, E., Hugunin, J., Kersten, M., Palm,
J., Griswold. W. G., (2001): An overview of AspectJ.
In proceedings of ECOOP 2001, Budapest, Hungary.
Komondoor, R. and Horwitz SS. (2000): Semantics-
Preserving Procedure Extraction. In Proceedings of
the 27th ACM SIGPLAN-SIGACT Symposium on
Principles of Programming Languages (POPL'00), pp.
155-169, Boston, MA, USA.
Krogdahl, S., Møller-Pedersen, B., Sørensen, F. (2005):
Exploring the use of Package Templates for flexible
reuse of Collections of related Classes, in Journal of
Object Technology, vol. 8, no. 7.
Mayrand, J., Leblanc, C. and Merlo, E. Experiment on the
Automatic Detection of Function Clones in a Software
System Using Metrics. In Proc. of the International
Conference on Software Maintenance, 1996.
McDirmid, S., Flatt, M. and Hsieh, W.C. Jiazzi: new-Age
Components for Old-Fashioned Java”, OOPSLA 2001.
Mezini, M. and Ostermann, K. 2003. Conquering Aspects
with Caesar. In Proc. of AOSD 2003, pages 90 – 99.
Przybyłek, A. /2011). Systems Evolution and Software
Reuse in Object-Oriented Programming and Aspect-
Oriented Programming , TOOLS 2011, LNCS 6705.
Quitslund, P. and Black, A. (2004): Java with traits -
improving opportunities for reuse. In Proceedings of
the 3rd International Workshop on Mechanisms for
Specialization, Generalization and inheritance.
Riehle, D. 2000. Framework Design: A Role Modeling
Approach, Ph. D. Thesis, Swiss Federal Institute of
technology, Zurich.
Roy, C. and Cordy, J. (2007) A Survey on Software Clone
Detection Research. Tech. Report 2007-451, School of
Computing, Queen’s University at Kingston.
Scharli, N., Ducasse, S., Nierstrasz, O. and Black, A.
(2003): Traits: Composable units of behavior. In
Proceedings of ECOOP 2003, volume 2743 of Lecture
Notes in Computer Science. Springer.
Smith, C. and Drossopoulou, S. (2005): Chai: Traits for
Java-like languages. In Proceedings of ECOOP 2005.
Steimann, F., (2000): On the representation of roles in
object-oriented and conceptual modeling. Data &
Knowledge Engineering 35(1):83–106.
Tamai, T., Ubayashi, N., and Ichiyama, R., (2007):
Objects as Actors Assuming Roles in the
Environment, in Software Engineering For Multi-
Agent Systems V, Lecture Notes In Computer
Science, vol. 4408. Springer-Verlag, Berlin.
ComposingClasses-RolesVsTraits
73