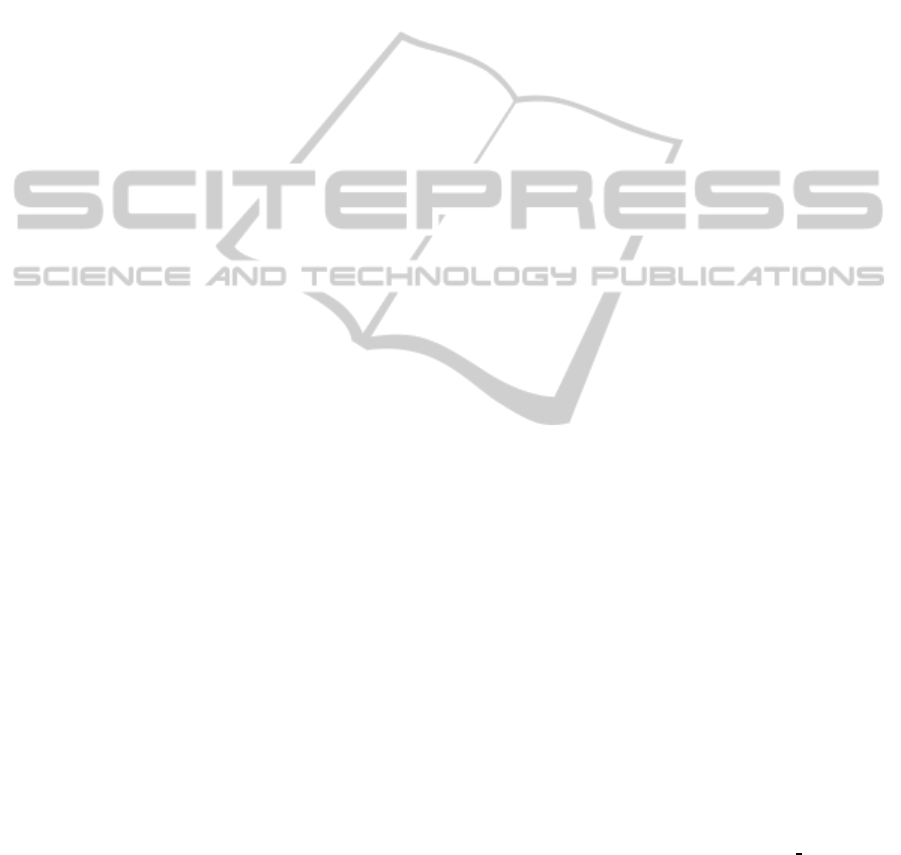
source license is available. It is a very rich frame-
work offering extensions for ExtJs, jQuery, Google
Maps, WebGL and many more. Again it supports
only JavaScript.
F#
Web Tools is an open source
tool whose main objective is not the translation to
JavaScript, instead, it is trying to solve the difficulties
of web programming: “the heterogeneous nature of
execution, the discontinuity between client and server
parts of execution and the lack of type-checked ex-
ecution on the client side”, see (Petˇr´ıˇcek and Syme,
2012). It does so by using meta-programming and
monadic syntax. One of it features is translation to
JavaScript. Finally, a translation between Ocaml byte
code and JavaScript is provided by Ocsigen, and de-
scribed in (Vouillon and Balat, 2011).
On the theoretical side, a framework integrat-
ing statically and dynamically typed (functional) lan-
guages is presented in (Matthews and Findler, 2009).
Support for dynamic languages is provided with ad
hoc constructs in Scala, see (Moors et al., 2012).
A construct similar to stm2exp, is studied in recent
work by one of the authors, see (Ancona et al., 2013),
where it is shown how to use it to realize dynamic
binding and meta-programming, an issue we are plan-
ning to address. The only work to our knowledge that
proves the correctness of a translation between a stat-
ically typed functional language, with imperative fea-
tures to a scripting language (namely JavaScript) is
(Fournet et al., 2013).
7 CONCLUSIONS
AND FUTURE WORK
In this paper we introduced
IL
an intermediate lan-
guage for the translation of a significant fragment
of
F#
to scripting languages such as Python and
JavaScript. The translation is shown to preserve
the dynamic semantics of the original language. A
preliminary version of this paper was presented at
ICTCS 2012, see (Giannini et al., 2012), which has
not published proceedings. We have a prototype im-
plementation of the compiler that can be found at
http://www.bluestormproject.org/. The compiler is
implemented in
F#
and is based on two metaprogram-
ming features offered by the .net platform: quotations
and reflection. Our future work will be on the practi-
cal side to use the intermediate language to integrate
F#
code and JavaScript or Python native code. (Some
of the features of
IL
, such as dynamic type check-
ing, were originally introduced for this purpose.) A
previous implementation of the translation supported
other features such as namespacing, classes, pattern
matching, discriminated unions, etc. We are in the
process of adding them at the current implementation,
since some of this features have poor or no support at
all in JavaScript or Python. On the theoretical side,
we are planning to complete the proofs of correctness
of the translations. We need to formalize our target
languages Python and JavaScript, and then prove the
correctness of the translation from
IL
to them. (We
anticipate that these proofs will be easier than the one
from
F#
to
IL
.) Moreover, we want to formalize the
integration of native code, and more in general meta-
programming on the line of recent work by the au-
thors, see(Ancona et al., 2013) . We are also consid-
ering extending the type system for the intermediate
language with polymorphic types, which is, as shown
in (Ahmed et al., 2011), non trivial.
ACKNOWLEDGEMENTS
We warmly thank Daniele Mantovani for his support
and involvement in the topic of the paper. We also
thank the anonymous referees of a previous version
of the paper for pointing out some problems which
lead to a substantial review of the intermediate lan-
guage. Any misinterpretation of their suggestions is,
of course, our responsibility.
REFERENCES
Ahmed, A., Findler, R. B., Siek, J. G., and Wadler, P.
(2011). Blame for all. In Proceedings of POPL 2011,
Austin, TX, USA, ACM, pages 201–214.
Ancona, D., Giannini, P., and Zucca, E. (2013). Recon-
ciling positional and nominal binding. In ITRS 2012,
EPTCS.
Appel, A. W. (1998). Modern Compiler Implementation in
ML. Cambridge University Press.
Bray, Z. (2013). Funscript. http://tomasp.net/files/funscript/
tutorial.html.
Fahad, M. S. (2012). Pit - F Sharp to JS compiler. http://
pitfw.org/.
Fournet, C., Swamy, N., Chen, J., Dagand, P.-
´
E., Strub, P.-
Y., and Livshits, B. (2013). Fully abstract compilation
to javascript. In POPL, pages 371–384. ACM.
Giannini, P., Mantovani, D., and Shaqiri, A. (2012). Lever-
aging dynamic typing through static typing. ICTCS
2012. http://ictcs.di.unimi.it/papers/paper
4.pdf.
Igarashi, A., Pierce, B., and Wadler, P. (2001). Feather-
weight Java: A minimal core calculus for Java and
GJ. ACM TOPLAS, 23(3):396–450.
Intellifactory (2012). Websharper 2010 platform. http://
websharper.com/.
Matthews, J. and Findler, R. B. (2009). Operational seman-
tics for multi-language programs. ACM Trans. Pro-
gram. Lang. Syst., 31(3).
ICSOFT2013-8thInternationalJointConferenceonSoftwareTechnologies
102