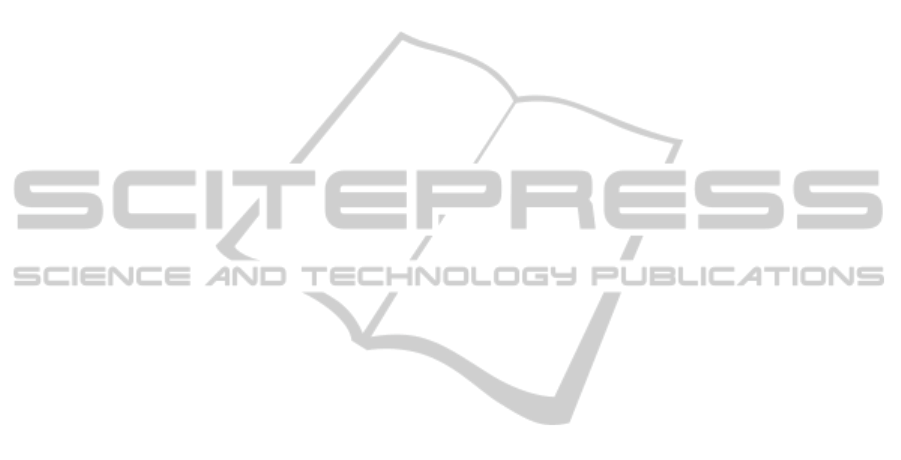
5 CONCLUSIONS
In this paper we have presented a comprehensiveanal-
ysis of several ray-triangle intersection algorithms
and their implementation in GPU using CUDA. When
programminghigh-performanceGPU algorithms, it is
important to keep in mind that the GPU architecture
forces the developer to adopt different principles than
the CPUs. Following these principles, this paper has
proposed some common design guidelines for suc-
cessfully porting most of the ray-triangle algorithms
to the GPU. Our exposition describes relevant aspects
such as the kernel implementation, memory access is-
sues and synchronization between threads.
We have also compared these GPU-based versions
with the original CPU implementations. A thorough
study of the influence of several optional features and
performance optimizations has also been reported. In-
terestingly, our experiments show that the use of pre-
computed values (a typical optimization used by most
CPU ray-triangle algorithms) is no longer required
and even counterproductive. Also, those optimiza-
tions that require additional branch control instruc-
tions or global memory accesses should be minimized
or avoided if possible, even if they apparently save
some computations.
ACKNOWLEDGEMENTS
This work has been supported by the Ministerio de
Econom
´
ıa y Competitividad of Spain and the Euro-
pean Union (via ERDF funds) through the research
project TIN2011-25259.
REFERENCES
Aila, T. and Laine, S. (2009). Understanding the efficiency
of ray traversal on gpus. In Proc. High-Performance
Graphics 2009, pages 145–149.
Amanatides, J. and Choi, K. (1995). Ray tracing triangular
meshes. In Proc. Western Computer Graphics Sympo-
sium, pages 43–52.
Badouel, D. (1990). An efficient ray-polygon intersection.
In Graphics Gems I, pages 390–394. Academic Press
Inc.
Benthin, C. (2006). Realtime Ray Tracing on current CPU
Architectures. PhD thesis, Saarland University.
Foley, T. and Sugerman, J. (2005). Kd-tree accelera-
tion structures for a gpu ray tracer. In Proc. of the
ACM Siggraph/Eurographics Conference on Graph-
ics Hardware, pages 15–22.
Glassner, A. (1989). An Introduction to Ray Tracing. Aca-
demic Press, New York.
Havel, J. and Herout, A. (2010). Yet faster ray-triangle in-
tersection (using sse4). IEEE Trans. Visualization and
Computer Graphics, 16(3):434–438.
Jim´enez, J. J., Segura, R. J., and Feito, F. R. (2010). A
robust segment/triangle intersection algorithm for in-
terference tests. efficiency study. Computational Ge-
ometry: Theory and Applications, 43(5):474–492.
Jones, R. (2000). Intersecting a ray and a triangle with
pl¨ucker coordinates. Ray Tracing News, 13(1).
Kensler, A. and Shirley, P. (2006). Optimizing ray-triangle
intersection via automated search. In Proc. IEEE Sym-
posium on Interactive Ray Tracing, pages 33–38.
Kim, S., Nam, S., Kim, D., and Lee, I. (2007). Hardware-
accelerated ray-triangle intersection testing for high-
performance collision detection. In Proc. Journal of
WSCG, volume 15.
Kirk, D. and Hwu, W. (2010). Programming Massively Par-
allel Processors. Morgan Kaufmann.
Lin, M. and Gottschalk, S. (1998). Collision detection be-
tween geometric models: A survey. In Proc. IMA
Conf. on Mathematics of Surfaces, pages 37–56.
L¨ofstedt, M. and Akenine-M¨oller, T. (2005). An eval-
uation framework for ray-triangle intersection algo-
rithms. Journal of Graphics Tools, 10(2):13–26.
M¨oller, T. and Trumbore, B. (1997). Fast, minimum storage
ray-triangle intersection. Journal of Graphics Tools,
2(1):21–28.
Noguera, J., Urena, C., and Garc´ıa, R. (2009). A vector-
ized traversal algorithm for ray tracing. In Proc. of the
Fourth International Conference on Computer Graph-
ics Theory and Applications (GRAPP 2009), pages
58–63. INSTICC Press.
Plucker, J. (1865). On a new geometry of space. Phil. Trans.
Royal Soc. London, 155:725–791.
Purcell, T. J., Buck, I., Mark, W. R., and Hanrahan, P.
(2002). Ray tracing on programmable graphics hard-
ware. ACM Transactions on Graphics, 21(3):703–
712.
Rueda, A. J. and Ortega, L. (2008). Geometric algo-
rithms on cuda. In Proc. 3rd International Confer-
ence on Computer Graphics Theory and Applications
(Grapp), pages 107–112.
Segura, R. J. and Feito, F. R. (1998). An algorithm for deter-
mining intersection segment-polygon in 3d. Computer
and Graphics, 22(5):587– 592.
Segura, R. J. and Feito, F. R. (2001). Algorithms to test
ray-triangle intersection. comparative study. Journal
of WSCG, 9(3):76–81.
Shevtsov, M., Soupikov, A., and Kapustin, A. (2007). Ray-
triangle intersection algorithm for modern cpu archi-
tectures. In Proc. International Conference on Com-
puter Graphics and Vision (GraphiCon 2007).
Woop, S., Benthin, C., and Wald, I. (2013). Watertight
ray/triangle intersection. Journal of Computer Graph-
ics Techniques (JCGT), 2(1):65–82.
GRAPP2014-InternationalConferenceonComputerGraphicsTheoryandApplications
246