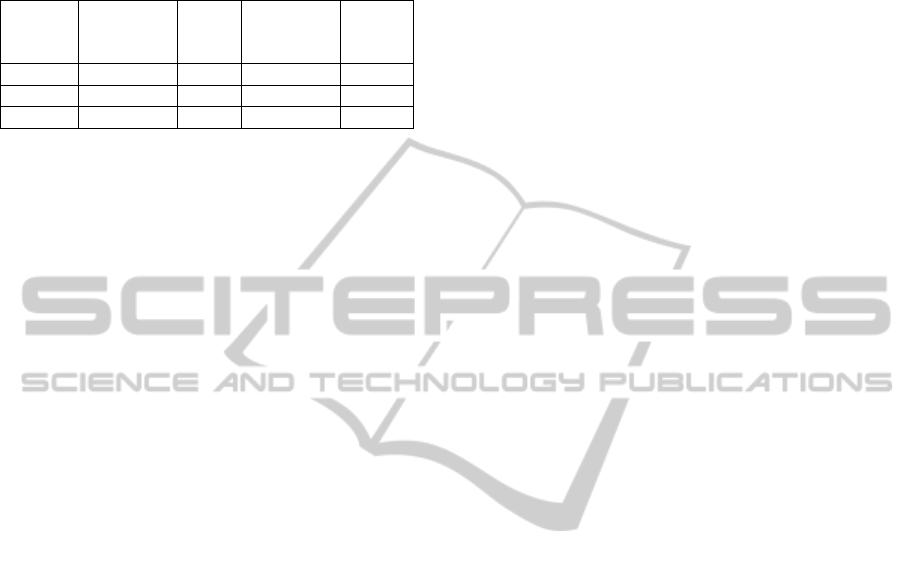
al., 2005). It is also reported that cyclomatic com-
plexity tends to be comparable to lines of code when
measuring complexity (Gill and Kemerer, 1991).
Table 1: Code generation comparison.
Rhapsody Niaz Umple
generated
Code
Umple
LOC
675 250 125 8
Bytes
24,270 6,420 5,010 197
Classes
7 11 1 1
As shown, the number of lines of code is significant-
ly lower in the case of Umple (a reduction of about
50% as compared with Niaz`s approach). The num-
ber of bytes is less in the case of Umple (a reduction
of about 22%).
This comparison cannot be a final word on gen-
erated code complexity. Other complexity measures
may give different results. In addition, It is possible
that Rhapsody or Niaz’s tool provide additional gen-
erated code to support functionality not available in
Umple. The generated code that was used in Niaz’s
study was not publicly available for inspection. On
the other hand, all of our examples can be compiled
and the code generated online. Making our algo-
rithm and its implementation available for future
research.
6 CONCLUSIONS
This paper presented an approach for code genera-
tion from composite state machines. We generate a
set of state machines equivalent to the original state
machine, and use ‘null’ states to indicate when any
given state machine is not active.
We presented cases covering each possible con-
figuration of composite states and their transitions.
Each case exposes an aspect of the code generation.
Our approach significantly reduces complexity and
code volume.
We have also introduced a unique concept in the
Umple model-oriented language where multiple
state machines can reside in the same class and
where multiple transitions can be fired by a single
event.
The code generation method and the associated
algorithms are implemented and are fully available
for inspection online.
REFERENCES
Badreddin O., Lethbridge T. C. and Elassar M., 2013.
"Modeling Practices in Open Source Software." Open
Source Software: Quality Verification. Springer. 127-
139.
Badreddin O., 2010. "Umple: A Model-Oriented Pro-
gramming Language," in Proceedings of the 32nd
ACM/IEEE International Conference on Software En-
gineering,Vol. 2, pp. 337-338.
Badreddin O., Forward A. and Lethbridge T.C., 2012.
"Model oriented programming: an empirical study of
comprehension." 2012 Conference of the Center for
Advanced Studies on Collaborative Research. IBM
Corp.
Badreddin O., Forward A., and Lethbridge T. C., 2014.
"Exploring a Model-Oriented and Executable Syntax
for UML Attributes." Software Engineering Re-search,
Management and Applications. Springer, 33-53.
Badreddin O., Forward A., and Lethbridge T. C., 2014.
"Improving Code Generation for Associations: En-
forcing Multiplicity Constraints and Ensuring Refer-
ential Integrity." Software Engineering Research,
Management and Applications. Springer, 129-149.
Badreddin O., Lethbridge T. C., 2013. “Model Oriented
Programming: Bridging the Code-Model Divide”.
ICSE Workshop on Modeling in Software Engineering
(MiSE), 2013 5th International Workshop,
10.1109/MiSE.2013.6595299. Pages: 69 - 75.
Badreddin O., Lethbridge T. C., Lethbridge T. C., 2012.
"Combining experiments and grounded theory to eval-
uate a research prototype: Lessons from the umple
model-oriented programming technology", User Eval-
uation for Software Engineering Researchers (USER),
2012. 10.1109/USER.2012.6226575. Page(s): 1- 4.
Badreddin O.,2013. "Empirical evaluation of research
proto-types at variable stages of maturity", User Eval-
uations for Software Engineering Researchers (USER),
2013 2nd International Workshop, 10.1109/
USER.2013.6603076. Pages: 1- 4.
Forward A., Badreddin O., Lethbridge T.C.,. "Perceptions
of Software Modeling: A Survey of Software Practi-
tioners," in 5th Workshop from Code Centric to Model
Centric: Evaluating the Effectiveness of MDD
(C2M:EEMDD), 2010. Available: www.esi.es/
modelplex/c2m/papers.php.
Gill G.K., Kemerer C.F., 1991. “Cyclomatic complexity
density and software maintenance productivity”, IEEE
Transactions on Software Engineering, 17(12).
Gold N. E., Mohan A. M., Layzell P. J., 2005. “Spatial
complexity metrics: an investigation of utility”, IEEE
Trans. on Software Engineering,.31(3), pp. 203-212.
Lano K., Clark D., 2007. "Direct Semantics of Extended
State Machines". TOOLS’07, pp. 35-51.
Lethbridge T.C., Badreddin O., 2011. "Umple - Associa-
tions and Generalizations". Accessed 2012,
http://www.youtube.com/watch?v=HIBo0ErCVtU.
Lethbridge T. C., Badreddin O., 2011. "Umple - State
Machines Details". http://www. Youtube. com/
watch?v=mFczzVkTZ9g.
MODELSWARD2014-InternationalConferenceonModel-DrivenEngineeringandSoftwareDevelopment
244