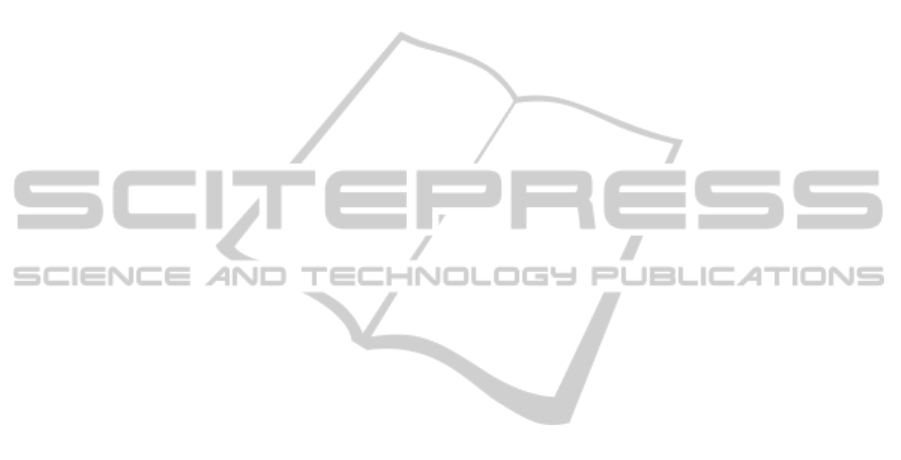
gram deviates from the formal semantics of the
language, an error is raised immediately.
This paper presents this debugger and exposes the
salient aspects of its implementation.
1.1 Debugging Web Applications
Most studies of the debugging of Web applications
consider the client-side of the applications. Beside
an early debugger for CGI applications by Vckovski
(Vckovski, 1998), most efforts concentrate on creat-
ing JavaScript debuggers for the Web browsers. Feld-
man and Sharma have both developed a remote de-
bugger for WebKit (Feldman, 2011; Sharma, 2012).
Mickens has developed a browser-agnostic JavaScript
debugger (Mickens, 2012). These tools offer facili-
ties for inspecting the JavaScript stack frame and for
breakpointing. They do not address the problem of
debugging Web applications globally. These studies
are complementary with our effort as Hop can use
them to step inside the client side execution, provided
they support the source map specification (Lenz and
Fitzgerald, 2011).
A previous study by E. Schrock (Schrock, 2009)
has identified many difficulties posed by JavaScript
when debugging Web applications. “In the early
days, the [JavaScript]’s ability to handle failure
silently was seen as a benefit. If an image rollover
failed, it was better to preserve a seamless Web ex-
perience than to present the user with unsightly error
dialogs.
This tolerance of failure has become a central de-
sign principle of modern browsers, where errors are
silently logged to a hidden error console... Now, at
best, script execution failure results in an awkward
experience. At worst, the application ceases to work
or corrupts server-side state. Tacitly accepting script
errors is no longer appropriate, nor is a one-line num-
ber and message sufficient to identify a failure in a
complex AJAX application. Accordingly, the lack of
robust error messages and native stack traces has be-
come one of the major difficulties with AJAX devel-
opment today... Ideally, we would like to be able to
obtain a complete dump of the JavaScript execution
context[.]”. This is exactly what Hop brings. In ad-
dition to offering multitier execution stacks that re-
flect the states of the server and the client, the Hop-
to-JavaScript compiler (Loitsch and Serrano, 2007)
generates codes which enable early error detection. It
also generates ECMA-262-5 strict mode (ECMA, 2009)
code which helps detecting undeclared variables and
functions that can be located inside JavaScript code
linked against Hop applications.
1.2 The Hop Programming Language
Hop has been presented in several publications (Ser-
rano et al., 2006; Boudol et al., 2012; Serrano and
Berry, 2012). We only remind its essential aspects
and show some examples that should be sufficient to
understand the rest of the paper.
Hop is a Scheme-based multitier functional lan-
guage. The application server-side and client-side
are both implemented within a single Hop program.
Client code is distinguished from server code by pre-
fixing it with the syntactic annotation ‘˜’. Server-side
values can be injected inside a client-side expression
using a second syntactic annotation: the ‘$’ mark. On
the server, the client-side code is extracted, compiled
on-the-fly into standard JavaScript, and shipped to the
client. This enables Hop clients to be executed by un-
modified Web browsers.
Except for multitier programming, the standard
Web programming model is used by Hop. A server-
side Hop program builds an HTML tree that creates
the GUI and embeds client-side code into scripts, then
ships it to the client. AJAX-like service-based pro-
gramming is made available by service definitions, a
service being a server-side function associated with a
URL. The with-hop form triggers execution of a ser-
vice. Communication between clients and servers is
automatically performed by the Hop runtime system,
with no additional user code needed.
The Hop Web application fib-html below con-
sists of a server-built Web page displaying a three-
rows table whose cells enumerate positive integers.
When a cell is clicked, the corresponding Fibonacci
value is computed on the client and displayed in a
popup window. Note the ‘˜’ signs used in lines 3,
8, 9, and 10 which mark client-side expressions.
1: (define-service (fib-html)
2: (<HTML>
3: ˜(define (fib x) ;; client code
4: (if (< x 2)
5: 1
6: (+ (fib (- x 1)) (fib (- x 2)))))
7: (<TABLE>
8: (<TR> (<TD> "fib(1)" :onclick ˜(alert (fib 1))))
9: (<TR> (<TD> "fib(2)" :onclick ˜(alert (fib 2))))
10: (<TR> (<TD> "fib(3)" :onclick ˜(alert (fib 3)))))))
11:
Let us modify the example to illustrate some Hop
niceties. Instead of building the rows by hand, we let
Hop compute them. The new Hop program uses the
(iota 3) expression (line 9) that evaluates to the list
(1, 2, 3) and the map functional operator that applies a
function to all the elements of a list. The $i expres-
sion (line 8) denotes the value of i on the server at
HTML document elaboration time.
WEBIST2014-InternationalConferenceonWebInformationSystemsandTechnologies
6