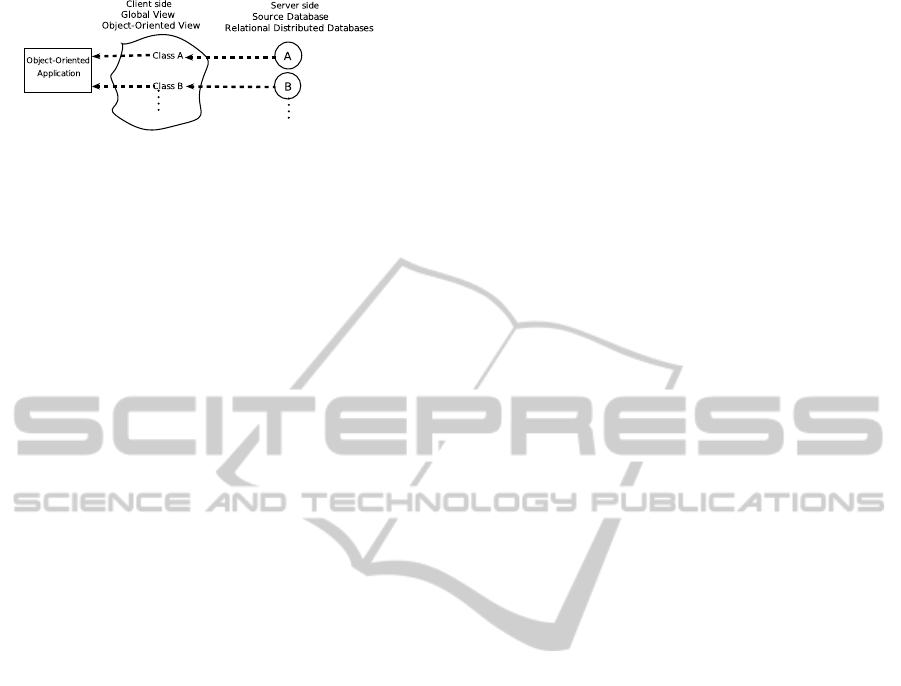
Figure 1: Model of the distributed system
tion is based on changing the object relational map-
ping. By default, object relational mapping shifts the
data processing to the client side to be consistent with
the virtual object database. This can be changed so
that data processing shifts to the source database side.
As a result, less data will transfer from the source
database side to the client side. The other solution is
to reduce the transmisison of a large number of data
from the source database side to the global view side.
This can be done by changing the configuration of
the application with more non-procedural code. This
means that, only the objects needed to satisfy the fil-
tering conditions of the application will be transferred
from the source database side to the global database.
This paper presents a number of transformation
rules which can eliminate iteration over a large
number of objects by changing the configuration of
the application. To do this, we used more OQL in the
body of the application. As a result faster and more
efficient performance of the application is achieved.
In the remainder of this paper, first the motivation
experiment is presented. Section 2, examine the cur-
rent research. Input patterns of the rules for different
styles of programs is presented in section 3. Section
4 presents the transformation rules. Verification and
logical proof of the rules is in section 5. Section 6
contains the conclusion and suggested future work.
1.1 Motivation Experiments
In this section, motivation experiments are pre-
sented. These experiments were conducted on the
TPC Benchmark website which has 300 MB rela-
tional data. All the experiments were run on a Dell
system with 3.33GHz intel(R), Core(TM)2, Duo CPU
by Ubuntu 10.04 LTS, the Lucid Lynx. The system
had 3.25GB RAM. The examples were run in Java
Persistence API (JPA) programming language format
and in the NetBeans 7 environment. The netbeans 7
clock were used to monitor execution time by sec-
onds.
Application 1, is the loop part of an original ap-
plication based on JPA, written by an object-oriented
programmer. Application 1 is an example of a
program that iterates over two relational databases,
called Lineitem and Supplier. This algorithm retrieves
all values from l.partsupp in the Lineitem class which
has equal value to the s.suppkey in the Supplier class.
We managed various experiments for different sized
databases, and measured the response time before
and after transformation. In all examples, Supplier
includes 3000 objects but size of the Lineitem class
varied between 100,000 objects to 2,000,000 objects.
Application 1:
{
Query query1 = em.createQuery
("SELECT s FROM Supplier s
ORDER BY s.sSuppkey", Supplier.class);
List list1 = query1.getResultList();
Iterator iterator1= list1.iterator();
while(iterator1.hasNext()){
Supplier s= (Supplier)iterator1.next();
Long ps_suppkey = s.getSSuppkey();
Query query2 = em.createQuery
("SELECT l FROM Lineitem l
WHERE l.partsupp.supplier.sSuppkey= "
+ ps_suppkey,Lineitem.class);
List list2 = query2.getResultList();
Iterator iterator2= list2.iterator();
}
By changing the configuration of application 1,
we were able to write application 2 which has exactly
same output as application 1. In application 2, we
obtained two SELECT statements together and used
one SELECT statement. By using application 2 and
changing the balance of the data processing, the un-
necessary iteration is eliminated. Also, configuration
of application 2 is such, that not all of the objects
will transfer from the server side to the client side.
As a result, the time needed for execution of the
application was reduced.
Application 2:
{
Query query = (Query) em.createQuery
("SELECT l.partsupp.partsuppPK.psSuppkey,
COUNT(l.partsupp.partsuppPK)
FROM Lineitem l JOIN l.partsupp ps
GROUP BY l.partsupp.partsuppPK.psSuppkey
ORDER BY l.partsupp.partsuppPK.psSuppkey",
Lineitem.class);
List<Object[]> list = query.getResultList();
Iterator iterator1= list.iterator();
}
Figure 2, illustrates the execution time which was
needed to run application 1 with different sizes of
the Lineitem class. We started running application 1
with 100,000 objects in relational database Lineitem
and increased the objects up to 2,000,000. The re-
sults made an exponentially chart shown as Figure
ICEIS2014-16thInternationalConferenceonEnterpriseInformationSystems
202