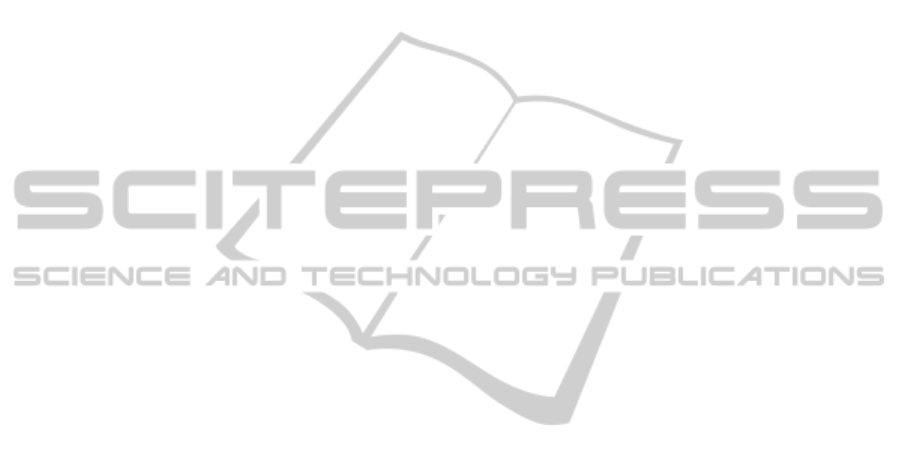
greenlets (Schmitt, 2012)) that can be used to simu-
late a large number of concurrent processes with very
little overhead. A process oriented paradigm can of-
ten greatly simplify the implementation of simulation
models, especially those used for computer systems
modeling.
The main goal in developing SimX was to enable
domain researchers to rapidly develop simulation ap-
plications and deploy it on clusters without having to
get bogged down by the intricacies of parallel pro-
gramming, managing MPI communication or worry
about issues such as load balancing. In recent years,
Python has emerged as a popular and attractive choice
for domain scientists due to its ease of use, flexibility
and expressive power. This made it a natural choice
as a front-end for SimX. A thriving and substantial
ecosystem for scientific computing in Python already
exists and an application developed in SimX can be
easily integrated with other modules available for sci-
entific computing in Python. SimX has been written
as a tool for research where developer cycles, are in
general, more valuable than program execution time.
Indeed, our initial experiences with SimX have shown
that the code base of a Python simulation application
is often smaller than its C++ counterpart by a factor of
four or more. While the flexibility of Python comes
with a performance cost, the implementation of the
core functionality in C++ provides a reasonable trade-
off between ease-of-use and performance. For situ-
ations where performance is an over-riding concern,
computationally intensive parts of the program can be
re-written in pure C++. In fact the entire simulation
application itself can be written in C++ as SimX al-
lows for applications to be pure Python, a Python/C++
hybrid or even pure C++.
In the following sections we discuss related work
and differences between our approach and others. We
then provide a brief architectural overview of SimX,
which includes a simple example illustrating some of
the features of SimX. We also provide some initial ex-
periences with SimX in terms of the program size of
Python simulations compared to equivalent C++ pro-
grams, and some initial scaling results.
2 RELATED WORK
The hybrid programming-language approach to simu-
lation is not new, the most notable example of this be-
ing the NS-2 network simulator (NS-2, ), which uses
a C++ backend with a TCL front end, it’s parallel and
distributed version called PDNS (PDNS, ) and more
recently NS-3 (NS-3, ) which uses C++ and Python
for parallel network simulation. SimX, however, is a
general purpose library, and not tied to any one do-
main; it can be used to develop applications in any
context where discrete-event simulation is an appro-
priate modeling paradigm.
There are a number of general purpose libraries
available for developing discrete-event simulations.
RePast (North et al., 2006) is a collection of open-
sourced modules for parallel agent based simulations
in C++ and Java. SASSY (Hybinette et al., 2006)
is a scalable agent simulation system for PDES that
provides a middleware between an agent-based API
and a PDES simulation kernel. MASON (Luke et al.,
2005) is a discrete-event multi-agent simulation li-
brary core in Java, designed to be the foundation for
large custom-purpose Java simulation. Another Java-
based simulation system is JiST (Barr et al., 2005),
that uses a virtual-machine based simulation approach
by embedding simulation semantics directly into the
Java execution model. PrimeSSF (PRIME, ), origi-
nally known as DaSSF, is a parallel simulation frame-
work, developed originally for network simulation,
that supports both distributed and shared-memory im-
plementations.
OMNET++ (OMNET++, 1996) is a component-
based C++ simulation library and framework, pri-
marily focussed on the domain of network simula-
tion. OMNET++ also supports parallel simulations.
ClusterSim (Ramos and Martins, 2004) is a Java-
based parallel discrete-event simulation tool for clus-
ter computing. ClusterSim supports visual model-
ing and simulation of clusters and their workloads
for performance analysis. µsik (Perumalla, 2005) is
a micro-kernel for PDES that supports both conser-
vative and optimistic parallel simulations. A more re-
cent effort is the ROme OpTimistic Simulator (ROOT-
Sim) (Pellegrini et al., 2011), an open source C/MPI-
based simulation package targeted at POSIX systems,
which implements a general purpose PDES environ-
ment with optimistic synchronization; the simulation
models, however, need to be implemented in C.
On the Python side, SimPy (SimPy, ) is an object-
oriented, process-based discrete-event simulation lan-
guage written in pure Python and provides the mod-
eler with classes for both active and passive compo-
nents in a simulation. Parallel support was later added
to SimPy, but the parallelism remains non-transparent
to the user. In the hybrid-approach category, PC-
Sim (Pecevski et al., 2009) is a C++ based neural net-
work simulator with a python front-end, that supports
both sequential and distributed memory simulations.
Thus while numerous libraries exist for parallel
discrete-event simulations for languages in languages
such as C, C++ and Java, and while packages also
exist for developing sequential discrete-event appli-
DevelopingParallel,DiscreteEventSimulationsinPython-FirstResultsandUserExperienceswiththeSimXLibrary
189