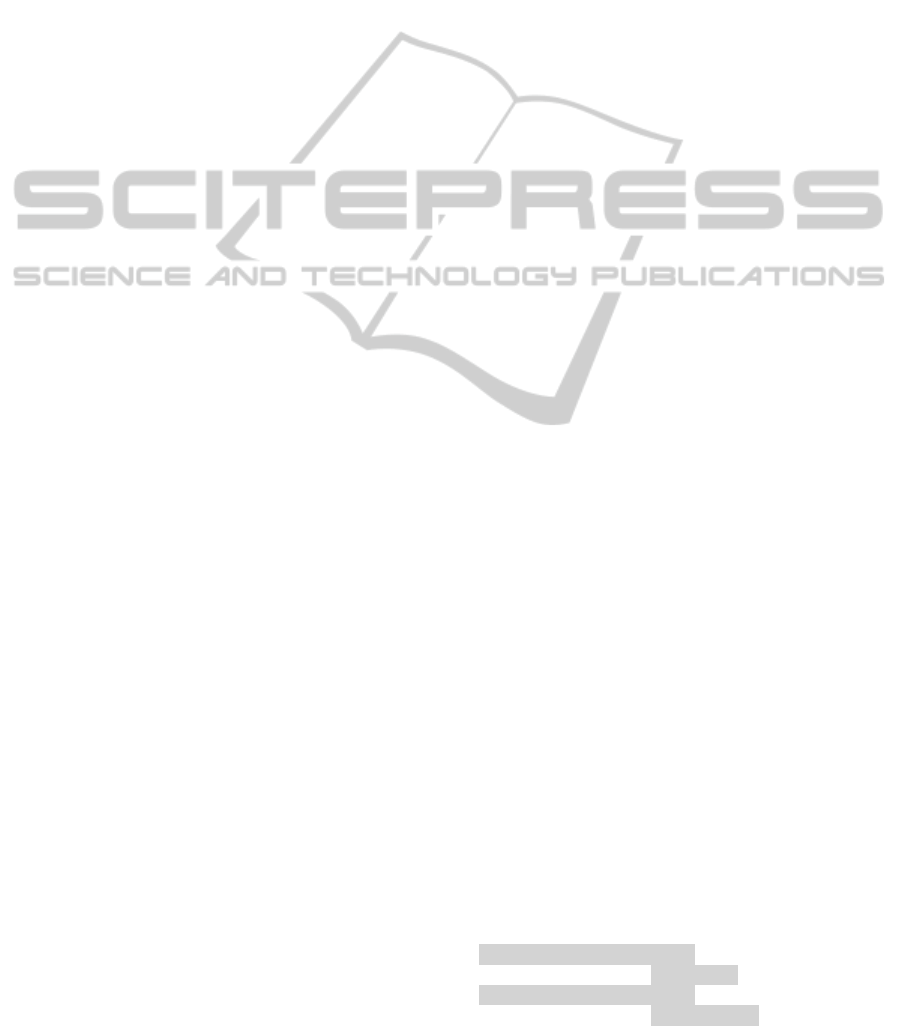
significantly helps properly structure the source
code.
A disadvantage of the Unit tests is that they can
validate the functionality, moreover only the
functionality of accessible methods. When we are
concerned about the proper usage of attributes or
private methods (common elements of well designed
code), Unit tests do not help.
To assess the internal quality of implementation,
a commonly used means are tools for static checking
of the source code. The most known ones are PMD
(PMD, 2014), CheckStyle or FindBugs. In the case
of checking of beginners’ programs we usually use
only very limited set of their possibilities (rules),
because there we do not expect sophisticated bugs to
appear in these simple programmes and the detailed
checks would overwhelm the students. Exaggerating
slightly, we use these tools mainly for checking how
“nicely written” the explored code is, i.e. its
comprehensibility for humans—eg. an inspection of
block parenthesis, an appropriate number of lines of
methods, suitable number of formal parameters, not
too big cyclomatic complexity etc.
A good programmer’s documentation is a
necessary part of a well written source code. Not
many solutions are available in this respect; one
such tool we developed (JavadocCheck, 2014) is
able to check an occurrence (but not
meaningfulness) of all the elements of the source
code which can be documented.
1.2 Tests of Quality of Implementation
and Meeting Assignment
Unfortunately, it is quite uncommon to
automatically test how student met the assignment
and what is the quality of implementation. Both
these needs are very important in the introductory
programming courses.
Simple tasks in these courses are very often
based on learning basic skills which students
sometimes struggle with. A typical negative example
is not using any formal parameters of method or
local variables, when students misuse “global”
attributes instead. “Magic numbers” in the source
code instead of using symbolic constants are another
example. Very confusing are insignificant names of
variables or methods (namely private ones).
Functional (Unit) tests are inherently not capable
to reveal all these flaws, and we cannot use them for
tests of documentation either. The tools for static
checking of the source code are successful in this
case, but only partly.
1.3 Goal of This Paper
The main goal of this article is to explore a solution
which would reconcile these two needs—to test that
functionality with respect to a given assignment is
correct and to check that the implementation is well
written, in an automated way. In the following
sections we first discuss a motivation example and
then describe a technique which has proven to be
useful in our work. It is based on a lesser known
approach of duck testing.
2 MOTIVATION EXAMPLE
Let’s have a typical assignment in beginner’s
courses of programming: Prepare the class
Person
which calculates a person’s Body Mass Index
(BMI). The “non-functional requirements” are:
The class is able to create immutable object only.
Attributes of this class are name, real weight (in
kg) and integer height (in cm).
The class has two constructors. A constructor
without parameters creates the person with name
Person, weight 65 (kg) and height 175 (cm). Use
symbolic constants for setting these values.
The constructor with three formal parameters sets
all the three attributes.
Both constructors calculate an integer value of
BMI and store it into an attribute.
BMI will be calculated by private method
calculateBMI().
All attributes will have getters only, no setter
(because of the immutable objects).
The class has an overriden method
toString(),
which returns eg. string
"
Person [w:65.0, h:175, BMI:21]".
2.1 Teacher’s Solution
The teacher’s idea of source code meeting the
assignment is as follows (the name of the class is
changed to
PersonByTeacher for the sake of
definiteness):
/** Class Person with atributes
* name, weight and height
* BMI is calculated
* immutable object - getters only
*/
public class PersonByTeacher {
private static final String
DEF_NAME = "Person";
private static final double
DEF_WEIGHT = 65.0;
DuckTestingEnhancementsforAutomatedValidationofStudentProgrammes-HowtoAutomaticallyTesttheQualityof
ImplementationofStudents'Programmes
229