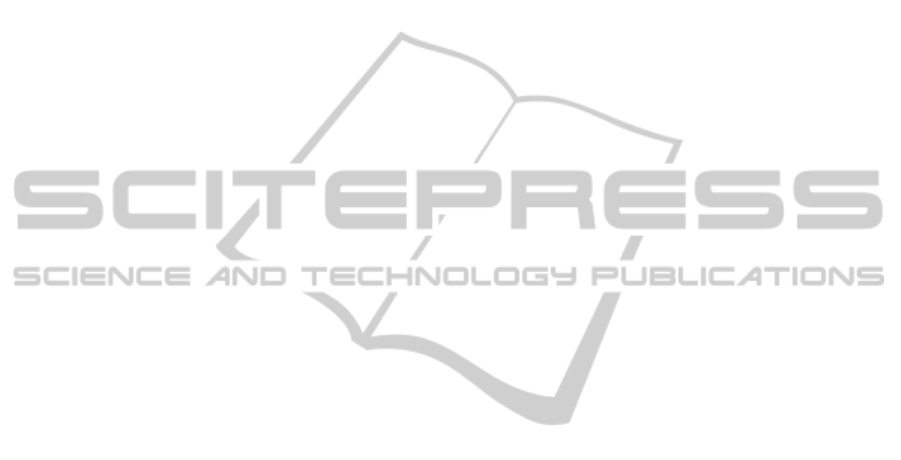
simplify the design of both wireless and wired
network interface device drivers.
Another issue related to Linux and b43.ko
interdependencies is the complexity of the module
architecture. The b43.ko module has a large number
of external interfaces making it difficult to decouple
the driver module from its operating environment.
By modeling a wireless device driver as a self-
contained object it becomes possible to eliminate all
other module interactions and provide a standard
interface directly to the application programmer.
This approach was used to develop device drivers
for a variety of bare machine applications. These
drivers require no OS, kernel, or embedded system
to support their operation.
The b43.ko module also incorporates general
functionality that is rarely used in a typical operating
environment. Such functionality makes the module
itself complex and large in size. This in turn makes it
harder to understand the driver, port it, and test its
operations. Bare machine drivers can solve these
problems; however, more research is required to
understand how to transform existing OS drivers so
that their OS dependencies are removed.
8 CONCLUSIONS
We studied the b43.ko Linux wireless driver module
and discussed its system view and code view, and
also its external interactions with other modules. The
code and functions used in the module were
classified based on their type of operation and
functionality. Design issues identified with the
design of b43.ko provide a starting point for future
development of device drivers that are independent
of any OS. The driver details provided in the paper
will be useful for developing techniques that can
transform OS drivers to bare machine drivers.
REFERENCES
Appiah-Kubi, P., Karne, R. K., Wijesinha, A. L., 2012. A
bare PC TLS webmail server. In ICNC.
Amar, A., Joshi, S., Wallwork, D., Generic driver model
using hardware abstraction and standard APIs.
Available from: <http://www.design-reuse.com/
articles/18584/generic-driver-model.html>. [12 Dec
2014].
Boyd-Wickizer, S., Zeldovich, N., 2010. Tolerating
malicious device drivers in Linux. In USENIX ATC.
Broadcom Wireless-Arch Wiki. Available from:
<http://wiki.archlinux.org/index.php/broadcom_wirele
ss>. [12 Dec 2014].
b43-Linux Wireless. Available from: <http://wireless.
kernel.org/en/users/Drivers/b43>. [12 Dec 2014].
Chipounov, V., Candea, G., 2006. Reverse engineering of
binary device drivers with RevNIC. In Eurosys.
Corbet, J., 2011. Broadcom's wireless drivers, one year
later. Available from: <http://lwn.net/Articles/
456762/>. [12 Dec 2014].
Doxygen. Available from: <http://www.stack.nl/
~dimitri/doxygen/>. [12 Dec 2014].
Ford, G. H., Karne, R. K., Wijesinha, A. L., Appiah-Kubi,
P., 2009. The design and implementation of a bare PC
email server. In COMPSAC.
He, L., Karne, R. K., Wijesinha, A. L., 2008. Design and
performance of a bare PC web server. International
Journal of Computers and Their Applications (IJCA).
Kadav, A., Swift, M. M., 2012. Understanding modern
device drivers. In ASPLOS XVII.
Karne, R. K., Jaganathan, K. V., Ahmed, T., Rosa, N.,
2005. Dispersed Operating System Computing
(DOSC). In Onward Track OOPSLA.
Karne, R. K., Wijesinha, A. L., Okafor, U., Appiah-Kubi,
P., 2013a. Eliminating the operating system via the
bare machine computing paradigm. In Future
Computing.
Karne, R. K., Liang, S., Wijesinha, A. L., Appiah-Kubi,
P., 2013b. A bare PC mass storage USB driver.
International Journal of Computers and Their
Applications (IJCA).
Khaksari, G. H., Wijesinha, A. L., Karne, R. K., He, L.,
Girumala, S., 2007. A peer-to-peer bare PC VoIP
application. In CCNC.
LeVasseur, J., Uhlig, V., Stoess, J., Gotz, S., 2004.
Unmodified device driver reuse and improved system
dependability via virtual machines. In OSDI.
NDISwrapper. Available from: <http://en.wikipedia.org/
wiki/NDISwrapper>. [12 Dec 2014].
Okafor, U., Karne, R. K., Wijesinha, A. L., Appiah-Kubi,
P., 2013. A methodology to transform OS based
applications to a bare machine application. In
TrustCom/ISPA/IUCC.
Rawal, B., Karne, R., Wijesinha, A. L., 2011. Mini web
server clusters based on HTTP request splitting. In
HPCC.
Source Insight Program Editor and Analyzer. Available
from: <http://www.sourceinsight.com>. [12 Dec
2014].
ENASE2015-10thInternationalConferenceonEvaluationofNovelSoftwareApproachestoSoftwareEngineering
300