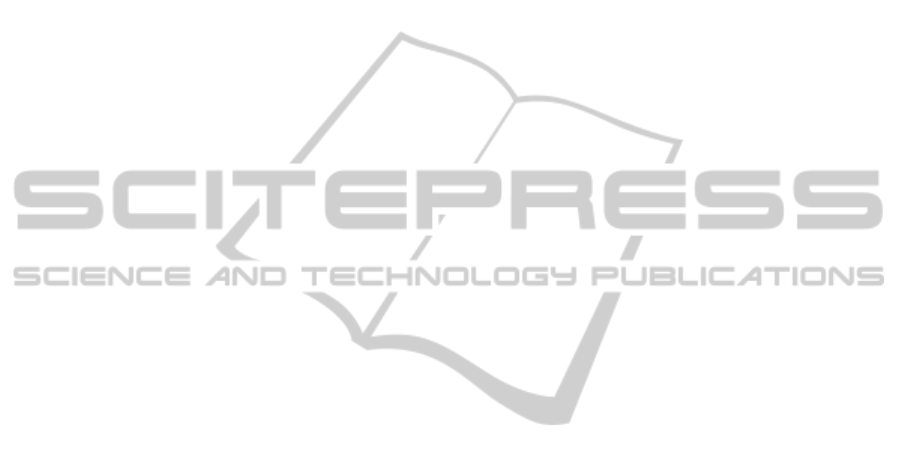
7 CONCLUSIONS
A new library of modular arithmetic and crypto-
graphic functions was developed and then used for a
specific implementation: a user-friendly file encryp-
tion tool aimed at quick deployment of secure com-
munication capability even in absence of a PKI.
In its current configuration the system is mainly
targeted to relatively small groups of users who have
already established communication links able to pro-
vide some form of identity validation. This could take
the form of a standard email system or possibly even
social networks, which could play the role of certifi-
cation authorities in the near future.
There are some features that distinguish the tool
from other personal encryption systems, notably PGP.
In our case the intention was to deploy a very light
system, i.e. with virtually no learning curve for the
user, but without sacrificing security.
Simplicity here means limiting the choice of cryp-
tographic algorithms to only those which are rele-
vant, thus avoiding the inclusion of a wide selection
of older ciphers or public key protocols with limited
added value. AES ad ECC represent the current state
of the art, therefore the idea was to stick to those
and simply offer different key lengths associated with
proven, standard elliptic curves. Another feature is
the ability to choose a random curve, thus creating a
unique set of parameters for extra security guarantee.
As opposed to PGP, the tool here presented is a
stand-alone system which is not designed to interface
with email through the use of plug-ins. These features
add sophistication but at the cost of complexity and
compatibility issues.
We aimed at privileging the most critical use
cases, typically associated with the occasional need
to protect specific files rather than the systematic en-
cryption of the whole email traffic. The adopted ap-
proach possibly sacrifices some elegance and integra-
tion in favor of enhanced simplicity.
In its current implementation the tool is not de-
signed to encrypt emails, rather it can selectively en-
crypt files for a specific recipient. Then the files can
be attached to an email addressed to the recipient who
can download and decrypt.
Additionally, the tool can be used to encrypt files
for secure storage on shared folders or in the Cloud.
Standard email systems are provided with some
level of security, however the content of messages can
in principle be altered because encryption takes place
between individual SMTP (Simple Mail Transfer Pro-
tocol) relays and not between sender and recipient.
Furthermore users normally have no control over this
feature. Trust in external bodies is therefore implicitly
required.
The proposed tool does not rely on centralized
infrastructures for the set-up of the security level,
key distribution and management of the encryp-
tion/decryption and signature functions. Full owner-
ship of the functionality reduces chances of external
interference.
In order to establish the system’s initial set-up,
one of the users shall first generate the curve param-
eters associated with a security level of choice and
then communicate the chosen parameters to the other
users. The security level can be changed by following
the above procedure if required.
The tool represents a readily deployable solution,
useful in absence of sophisticated PKIs.
Possible future developments may involve the
addition of Supersingular Isogeny Diffie-Hellman
Key Exchange (SIDH) which would provide post-
quantum resilience, as suggested in (L. De Feo,
2011). The already available elliptic arithmetic li-
brary would make this extension not too complex.
REFERENCES
D. Hankerson, A. J. Menezes, S. V. (2004). Guide to Elliptic
Curve Cryptography. Springer-Verlag.
Koblitz, N. (1994). A Course in Number Theory and Cryp-
tography. Springer-Verlag.
L. De Feo, D. Jao, J. P. (2011). Towards quantum-resistant
cryptosystems from supersingular elliptic curve isoge-
nies. In PQCrypto Proceedings. Springer.
NIST (2001a). FIPS PUB 197, Announcing the Advanced
Encryption Standard (AES). Federal Information Pro-
cessing Standard.
NIST (2001b). Special Publication 800-38A, Recommen-
dation for Block Cipher Modes of Operation, Methods
and Techniques. NIST Publication.
NIST (2002). FIPS 180-2, Announcing the Secure Hash
Standard. Federal Information Processing Standard.
NIST (2005). NIST-Recommended Random Number Gen-
erator Based on ANSI X9.31, Appendix A.2.4: Using
the 3-Key Triple DES and AES Algorithms. NIST Pub-
lication.
NIST (2007). Special Publication 800-90, Recommenda-
tion for Random Number Generation Using Determin-
istic Random Bit Generators (Revised). NIST Publi-
cation.
NIST (2009). FIPS PUB 186-3, Digital Signature Standard
(DSS). Federal Information Processing Standard.
R. Crandall, C. P. (2005). Prime Numbers, A Computational
Perspective. Springer.
V. Gruhn, M. Hulder, V. W.-M. (2007). Utilizing social
networking platforms to support public key infrastruc-
tures. In SECRYPT 2007 Proceedings. SCITEPRESS.
APublic-KeyCryptographyToolforPersonalUse-AReal-worldImplementationofECCforSecureFileExchange
201