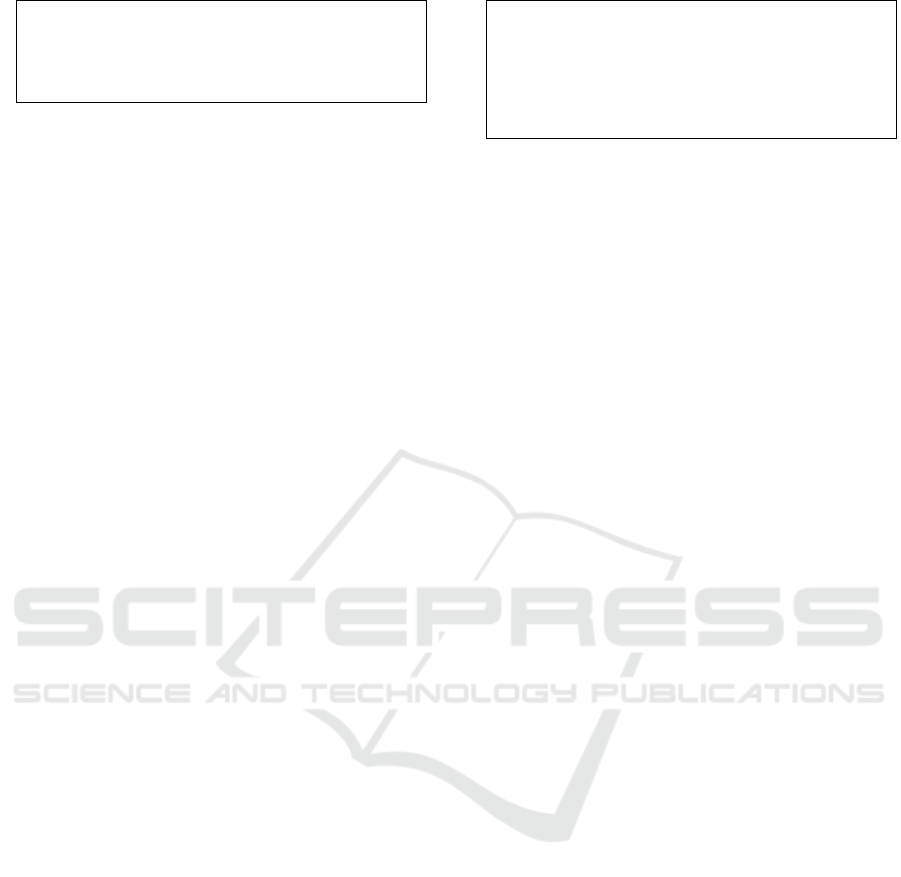
public class MyCustomModule extends EmfParsleyGuiceModule {
2 public Class<? extends ILabelProvider> bindILabelProvider() {
return MyLabelProvider.class;
4 } ...
}
Listing 3: Binding the custom label provider.
Reusable UI Components. EMF Parsley pro-
vides an infrastructure of Java classes and the injec-
tion mechanisms to configure any Eclipse UI compo-
nent, e.g., views and editors. It also provides some of
these components that can be used out-of-the-box and
that can be easily customized. We provide Eclipse ed-
itors for editing EMF models. We also provide views
that can also edit EMF models.
Such views can represent EMF models with a tree,
a table, a form, or a combination of them. Similarly,
we provide views that react on selection, that is, they
show an EMF object that is selected in another view
or editor.
Our implementations also take care of notifica-
tions occurring in the underlying EMF model, so that
we can handle “dirty” state in the editors and views
and we also ensure that all the UI elements are auto-
matically updated if they share the same EMF model.
Undo and Redo mechanisms are also automati-
cally handled by EMF Parsley.
3 THE DSL
Although EMF Parsley can be used directly from
Java, the DSL makes the use of the framework and the
development of EMF applications with EMF Parsley
even easier.
With our DSL we can easily specify and cus-
tomize the aspects of UI components of our frame-
work without writing Java code and without writing
the corresponding Guice module bindings. The DSL
compiler will then generate the corresponding Java
classes.
Xbase. The DSL uses Xbase (Efftinge et al.,
2012) for the expression language. Xbase is a
reusable Java-like expression language, completely
interoperable with the Java type system (including
generics). This means that all the existing Java li-
braries can be used in our DSL. Java programmers
will be able to easily learn the Xbase language. Al-
though Xbase expressions are Java-like, Xbase re-
moves most of the “syntactic noise” from Java, with
type inference, syntactic sugar for getters/setters and
extension methods, which simulate adding new meth-
ods to existing types without modifying them. Xbase
provides lambda expressions, which have the shape
1 module org.eclipse.emf.parsley.examples.librarytreeform {
parts {
3 viewpart views.librarytreeform {
viewname "My Library Tree Form"
5 viewclass SaveableTreeFormView
}
7 } ...
Listing 4: An example of module definition in EMF
Parsley DSL.
[ param1, param2, ... | body ] The types of
parameters can be omitted if they can be inferred from
the context. For these reasons, Xbase expressions are
quite compact, very readable, and look like they are
written in an untyped language.
Specification Structure. A specification in EMF
Parsley DSL, i.e., an input file, consists of a mod-
ule that will correspond to a Guice module in the
generated Java code. Inside this module we specify
customizations (corresponding to the Java customiza-
tions we sketched in Section 2). Each customization
has its own specific section, as we will see in the rest
of the section.
The DSL will then generate the corresponding
Java classes, and the corresponding custom bindings
in the generated Guice module. This way, the cus-
tomizations are specified in a much more compact
form and they are all grouped together in a single file,
instead of being spread into several Java classes.
EMF Parsley provides some Eclipse project wiz-
ards to create projects with an initial setup for the
DSL. These wizards also provide initial templates for
specific views (e.g., tree, tree with form, table, etc.).
Besides customizations, in the module one can
specify also sections corresponding to Eclipse parts
(i.e., views and editors). From such section, the
DSL compiler will generate the plugin.xml with the
Eclipse extension points.
In Listing 4 we show an example of module speci-
fication (together with a view part specification) writ-
ten in the EMF Parsley DSL. These initial contents
are created by our project wizard, mentioned above,
selecting the “tree form” template.
The part specification resembles the extension
point for Eclipse view parts. The “viewclass” refers to
the Java type SaveableTreeFormView which is one
of the views that come with the EMF Parsley distribu-
tion (see Section 2). The specification in Listing 4 is
enough to have a working tree form view that edits an
EMF resource, as shown in Figure 1. The form allows
the user to edit the element selected in the tree. In this
case, we delegate to EMF.Edit standard reflective be-
havior, as described in Section 2.
Actually, we still need to tell such view which
EMF resource to represent. In order to do that, we
do not need to subclass SaveableTreeFormView and
The EMF Parsley DSL for Developing EMF Applications
303