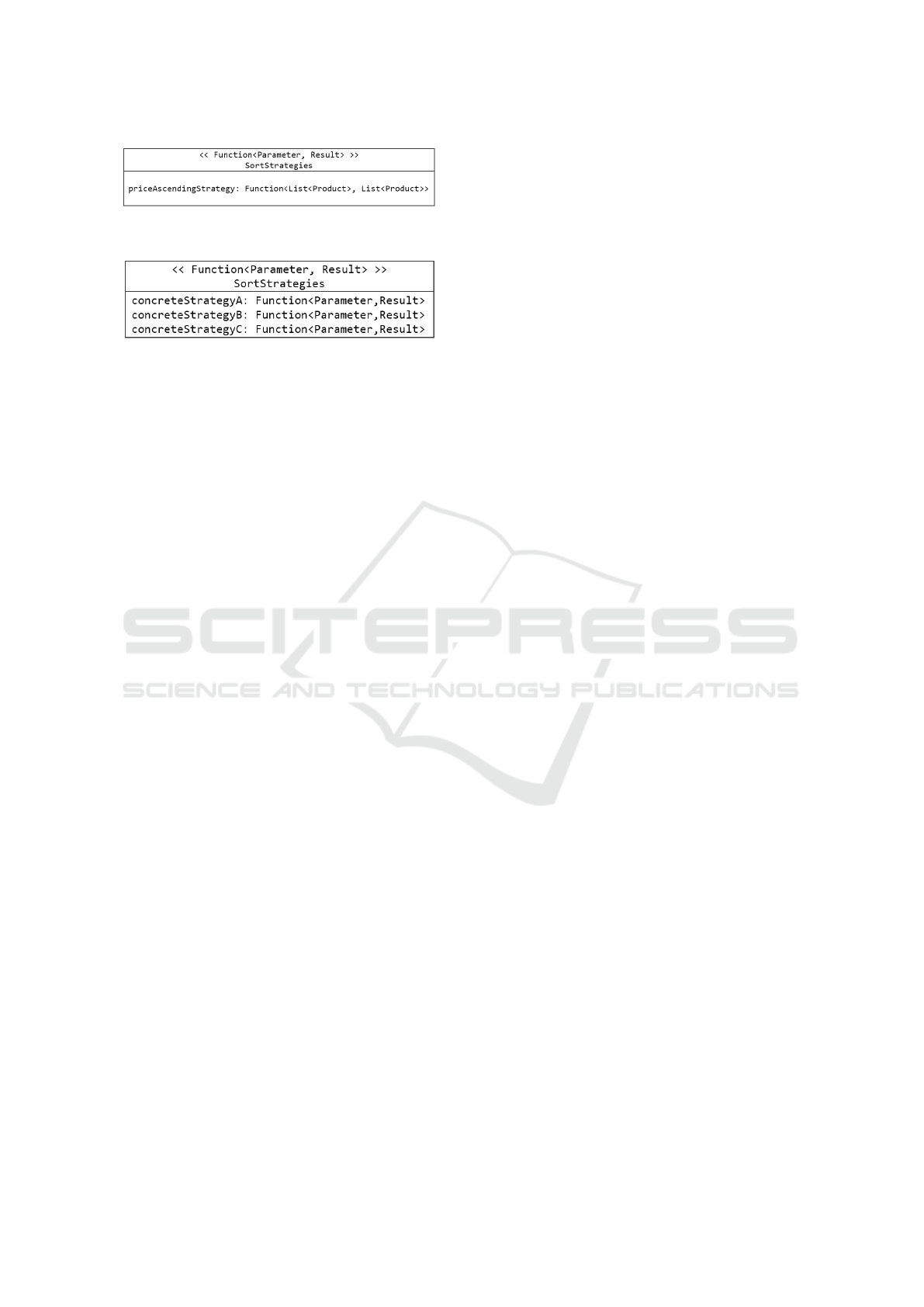
Figure 4: Function stereotype example with one function
reference.
Figure 5: Function stereotype with several function refer-
ences.
type Parameter and return type Result given in the
stereotype. Figure 4 shows an example function from
our online shop which contains a function reference
taking a list of products and returning a sorted prod-
ucts list.
We can also group function references as shown
in Figure 5. The stereotype specifies that all func-
tion references work with the parameter and result
type specified by the stereotype. That means the
stereotype forces all Function objects to be of type
Function<Parameter,Result>.
After all this effort, we can now take a look again
at the strategy pattern in Figure 6, this time expressed
with a function. The context has an aggregation to
SortStrategies. This aggregation means—depending
on the multiplicity—that the Context can have be-
tween 0 and n function references with the type de-
fined in the stereotype. Usually this attribute is filled
with the Functions attributes listed inside the function
by holding a direct reference (i.e. concreteStrategyA).
3 SPECIFICATION OF THE
FUNCTION ELEMENT
In UML, we apply stereotypes to ”transform” classes
to either interfaces, abstract classes, enums, or others.
Our approach utilizes this mechanism to introduce the
function element. Until now, it has not been possible
to directly associate function references with other el-
ements in class diagrams. With the function, it is pos-
sible to treat function references as first class citizens
in class diagrams.
3.1 Function Element
In the following, we specify the function and its se-
mantics.
In Figure 7, a general representation of the func-
tion is shown. The function has a name (Function-
Name), a stereotype with parameter and result type,
and several attributes with a type specified by the
stereotype. The following sections discuss the com-
ponents of function in detail:
Name: The name serves—as usual—as a good
description for the element in order to provide a clear
understanding of the reality captured in the model.
Stereotype: Our stereotype is named Function
and enables the modeler to distinguish between func-
tion and normal classes. Furthermore, the Function
stereotype can be parameterized to specify the object
types that all its function references take as parameter
and return types. In case the stereotype is not param-
eterized, the attributes (i.e. function references) must
indicate the parameter and the return type themselves.
Attributes: The attributes of a function are static
in nature because they are known at coding time. As a
consequence, the attributes are all non-modifiable and
therefore read-only. The attributes are by default pub-
lic and the readOnly property can be omitted. Also,
the type of the attribute can be omitted if it is already
specified by the stereotype. Such a specification is
depicted in Figure 8. Non function references are
not allowed. In case a state is needed across several
function references, a normal class should be used for
modeling.
The parameters have a polymorphic character.
That means that either the type of the parameter itself
or of a subclass can be used.
If several arguments are to be passed to the func-
tion reference, they should be encapsulated in a single
object. Alternatively, it would also be possible to use
a parameter list and interpret the last parameter as the
return type. As a remark: Common programming lan-
guages such as C# and Java have decided against this
approach and work instead with a single parameter
type and a single return type.
3.2 Multiplicity and Relations
An element can be related to a number of functions
in the same ways it can be related to other objects. In
Figure 9, an instance of Context holds exactly n func-
tion references specified in our function. The main
difference to normal classes is that not n objects of
FunctionName are held by the Context instance but
n attributes (i.e. function references) inside of Func-
tionName. All known multiplicities from UML can be
utilized with the multiplicity of n representing several
function references.
Function References as First Class Citizens in UML Class Modeling
337