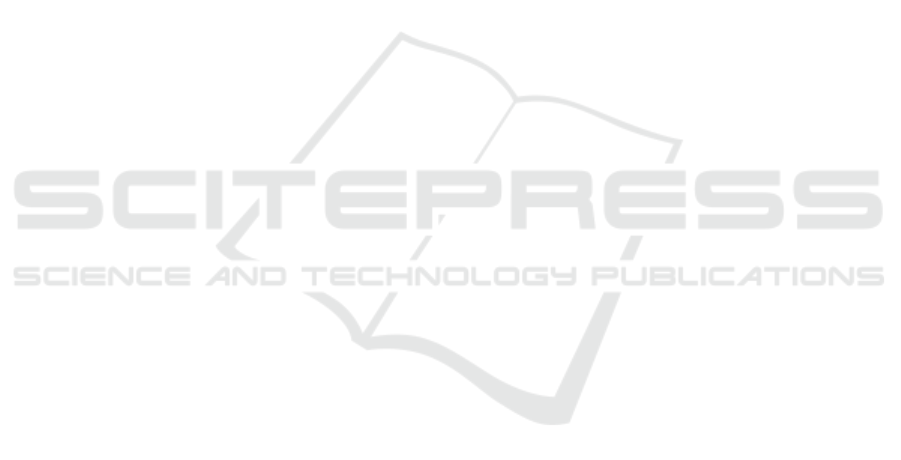
restrictions and wide range of applications. For
example, we can record the address of devices that
passed while the agent was moving, and use it to
return to the transmitter of the agent.
We have developed a simulator of a multi-robot
system to demonstrate the feasibility of our agent
system. When the user executes the agent system, the
agents move to robots that mimic PSO in the multi-
robot system. We could demonstrate the feasibility of
our agent system on a set of search robots.
In the current system, each agent is passed as a
program file, and when it arrives at the destination,
the agent does not have any local data. Local data are
also sent with the agent so that the agent acquires the
necessary data from the file. This is a disadvantage.
We will re-design the agent system so that the agent
can move with its own environment and maintain its
own state even during execution.
The current system does not allow interruption
during the execution and the migration of the agent.
At the next stage, we will extend the agent system so
that execution can be interrupted and moved to a
destination. And then, we will build an actual multi-
robot system for search as a future work.
ACKNOWLEDGEMENTS
This work is partially supported by Japan Society for
Promotion of Science (JSPS), with the basic research
program (C) (No. 17K01304), Grant-in-Aid for
Scientific Research (KAKENHI) and Suzuki
Foundation.
REFERENCES
Braun, P. and Rossak, W.R., 2005. Mobile Agents: Basic
Concepts, Mobility Models, and the Tracy Toolkit,
Morgan Kaufmann.
Ishiwatari, H., Sumikawa, Y., Takimoto, M. and
Kambayashi, Y., 2017. Cooperative Control of Multi-
Robot System Using Mobile Agent for Multiple Source
Localization, In Eighth International Conference on
Swarm Intelligence, pages 210-221.
Kambayashi, Y. and Takimoto, M., 2005. Higher-Order
Mobile Agent for Controlling Intelligent Robots,
International Journal of Intelligent Information
Technologies, 1(2), 28-42.
Kambayashi, Y., Nishiyama, T., Matsuzawa, T., and
Takimoto, M., 2016. An Implementation of an Ad hoc
Mobile Multi-Agent System for a Safety Information,
In Thirty-sixth International Conference on
Information Systems Architecture and Technology,
AICS vol. 430, pages 201-213.
Kennedy, J. and Eberhart, R., 1995. Particle swarm
optimization, In IEEE International Conference on
Neural Networks 4, pages 1942-1948.
Nagata, T., Takimoto, M. and Kambayashi, Y., 2013.
Cooperatively Searching Objects Based on Mobile
Agents, Transaction on Computational Collective
Intelligence XI, pages 119-136.
Nishiyama, H., Ito, M., Kato, N., 2014. Relay-by-
Smartphone: Realizing Multi-Hop Device-to-Device
Communications. IEEE Communications Magazine,
vol. 52, no. 4, Apr, pp. 56-65.
Oda, K., Takimoto, M. and Kambayashi, Y., 2018. Mobile
Agents for Robot Control Based on PSO, In Tenth
International Conference on Agents and Artificial
Intelligence, pages 220-227.
Stone, P. and Veloso, M., 2000. Multiagent systems: A
survey from a machine learning perspective,
Autonomous Robots, 8(3), 345-383.
Taga, S., Matsuzawa, T., Takimoto, M. and Kambayashi,
Y., 2017. Multi-Agent Approach for Evacuation
Support System, In Ninth International Conference on
Agents and Artificial Intelligence, pages 220-227.
Taga, S., Matsuzawa, T., Takimoto, M. and Kambayashi,
Y., 2016. Multi-Agent Approach for Return Route
Support System Simulation, In Eighth International
Conference on Agents and Artificial Intelligence, pages
269-274.
Taga, S., Matsuzawa, T., Takimoto, M., Kambayashi, Y.,
2018 Multi-agent Base Evacuation Support System
Using MANET, In Tenth International Conference on
Computational Collective Intelligence, LNAI 11055,
pages 445-454.
HAMT 2019 - Special Session on Human-centric Applications of Multi-agent Technologies
320