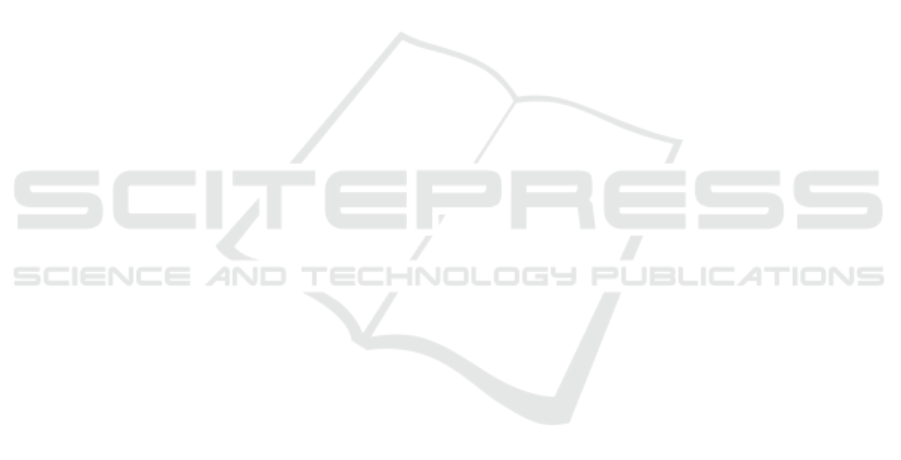
that our algorithm excels.
This issue is not a problem to Serial (Algorithm 1)
neither to the proposed Parallel (Algorithm 2), which
do not need to receive guessed parameters and its de-
tection performance does not change abruptly. For
instance, specifying an small radius range of 10 pix-
els has a better performance than specifying the range
from zero to half the image’s width. Also, the parame-
ters associated with the OpenCV implementation may
vary from image to image, depending on the scale and
the illumination presented in the image.
When there is an obstructed circle, the OpenCV
implementation has a higher chance of considering it
a circle, depending on the tolerance of its parameters,
which mostly increase wrong identification cases. But
both the Serial and the proposed Parallel implementa-
tions (Algorithm 1 and Algorithm 2, respectively) are
robust enough to identify the obstructed circles with-
out affecting the result quality.
4 CONCLUSIONS
In a context of circle identification, where the envi-
ronment variables, such as light, changes quickly, it
is very important to have an algorithm implementa-
tion that allows result stability and quality without a
dynamic change on the received parameters. This im-
plementation is the objective of this paper, which was
motivated by the need to balance circle identification
performance with the achievement of appropriated re-
sults independent of contexts.
In this context, we proposed a parallel algorithm
for circle identification. We applied Hough Trans-
form as the foundation for our algorithm and added
parallelism using the MapReduce paradigm. For exe-
cution purposes, we used Spark framework, which is
a framework to support distributed computing.
We compared the proposed parallel algorithm
with analogous implementations, also using Hough
Transform. We investigated the serial version of
our proposal. We also explored the OpenCV Hough
Transform Algorithm in two ways: in a simplified
way (with predefined parameters) and in a general
way (without predefined parameters).
We found that the main advantage of our proposal
as well as its serialized version is the algorithm gen-
eralization. Such implementations can than be used
to detect different objects as a circle in distinct con-
texts without the need to set specialized configura-
tions. Regarding the detection results, the OpenCV
achieved good results when properly configured. The
OpenCV in a general way was unable to identify cir-
cles correctly, since it ended up with many false pos-
itives. To avoid it, some parameters, such as circle
radius limits, should be defined, even when the ade-
quate values for them are mutable, like when the cam-
era gets closer to the object, the circle radius limits
should change, or when the environment gets lighter,
a different filter parameter should be applied. As ex-
pected, our parallel algorithm gave the same detection
results that its serialized version. However, some re-
sults were not small formations not consistent with
the expected circles, so we need to investigate how to
improve the algorithm in this way.
Regarding performance, OpenCV implementation
has a very good performance, mainly when the pa-
rameters are specified correctly. But, since this im-
plementation relies deeply on the correct choice of
these parameters, when the context changes a little
the performance may decay significantly. The serial
algorithm has the largest processing time of the eval-
uated algorithms, while having a generalized perfor-
mance as good as our parallel implementation. Nev-
ertheless, this serial algorithm can take up to a day of
processing time for high resolution images. Although
the performance of the proposed parallel algorithm
was not impressive, such parallel approach requires
further evaluations, since it relies on cluster architec-
ture and processing power. The interesting result is
that the performance of parallel algorithm is not neg-
atively impacted by context changes.
More experiments and comparisons should be
studied. As future work, we intend to investigate other
parallelism framework different from Spark, other
programming languages, as well as other execution
platform in order to improve performance of our par-
allel algorithm. We will also test different blurring fil-
ters and edge detectors, aiming to reduce the errors in
the circles identification. We argue that, with an opti-
mized implementation, our proposal can adequately
support circle detection in real distributed systems’
problems.
REFERENCES
Bay, H., Ess, A., Tuytelaars, T., and Van Gool, L. (2008).
Speeded-up robust features (surf). Comput. Vis. Image
Underst., 110(3):346–359.
Bradski, G. (2000). The OpenCV Library. Dr. Dobb’s Jour-
nal of Software Tools.
Canny, J. (1986). A computational approach to edge de-
tection. IEEE Trans. Pattern Anal. Mach. Intell.,
8(6):679–698.
Dean, J. and Ghemawat, S. (2004). Mapreduce: Simplified
data processing on large clusters. In Proceedings of
the 6th Conference on Symposium on Operating Sys-
tems Design & Implementation - Volume 6, OSDI’04,
ICSOFT 2019 - 14th International Conference on Software Technologies
458