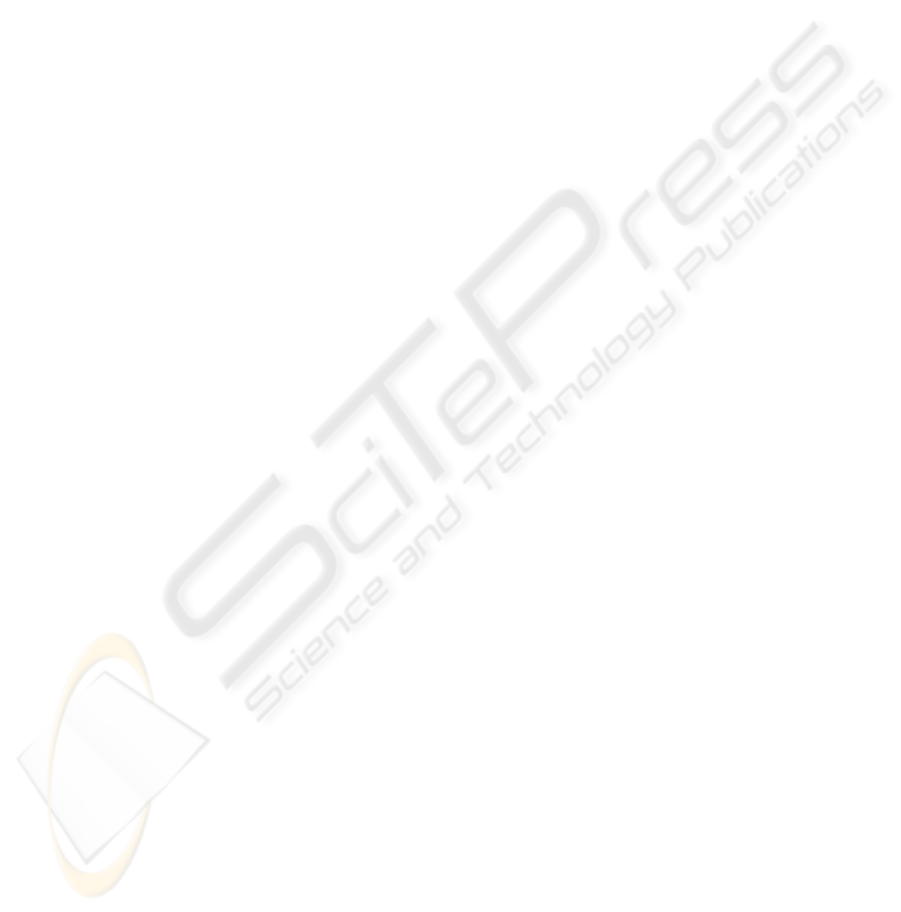
1. The assignment starts from the highest price bid.
In the first stage, the set of asks with the highest unit
contribution are determined. Then we obtain
maximum quantity that can be assigned. Then
assignment is carried out. We combine asks with
the same unit contribution and always determine
maximum possible quantity that can be assigned. If
ask quantity is less than the bid quantity, then the
ask is marked as temporarily assigned, while bid is
marked as partly assigned and assignment continues
from the next ask onwards till complete demand is
fulfilled. The bid and/or all asks are marked as
assigned. If ask has more quantity, then bid is
marked as assigned and ask is marked as partly
assigned. Then assignment is continued in
decreasing order of price for bids.
2. Initially a table indicating demand for different
width is constructed. If the ask size is multiple of
bid size (e.g. bid width 800 cm and ask width 1600
cm), then this table is used to see whether there is
demand for the remaining width (i.e. 800 cm). If
there is demand, then wastage parameter is set to 0.
This is helpful in situations to decide, whether bid is
to be matched with ask of 1600 cm or 1000 cm.
Here matching of current bid with ask width of 1000
cm will show lesser wastage than that of 1600 cm.
However, if there is demand for 800 cm, then it can
be matched with the ask of 1600 cm width so that
wastage is minimized.
The assignment is continued till one of the three
conditions holds (i) no ask is left (ii) no bid is left
(iii) when ask price exceeds that of bid price. In our
solution, assignment is carried out if bid price is
either more than ask price or both are same. This
assumption is reasonable in the sense that in most
exchanges asks are cleared with bids of same or
higher price. It can also be seen in (
Kalagnanam,
2001)
, that equilibrium price is first obtained. This
price is used to determine the asks and bids which
can be cleared (asks below this price and bids above
it). Let A be the list of asks and B be the list of bids.
Algorithm findopasg (A,B) /* Main Algorithm */
Call Create_ Size_Demand_Table(bids) ;
While ( there is unassigned bid in B ){
Call get_next_unassigned_bid(bids) ;
Call get_opt_ask(bid_quantity,bid_size,) ;
Call assignment(bid,ask,bid_quantity) } return ; } /*
End of main algorithm **/
/** Function to create Size Demand Table **/
create_Size_Demand_Table(bids) {
while ( there is next bid) { get bid_size, bid_quantity ;
call search_table(bid_size,table_size) ;
if ( .not.. found )then { increase current_index by 1 ;
store bid_size to search_table(current_index,1) ;
store bid_quantity to search_table(current_index,2) ;
set table_size to current_index ;}
else{ add bid_quantity to search_table(current_index,2);}
read next_bid ; } return ; }
/** Function to search table **/
Search_Table(bid_size,table_size) {
while ( i <= table_size ) {
If (search_table(I,1) = bid_size) then {set
current_index to i ; return .true ; }
else {increase i by 1 ;} return false; }
/** This function gets next highest price bid **/
get_next_unassigned _bid(bids) {
while ( there is unassigned bid) {
if (bid_price > max_price) then { set max_price to
bid_price; set bid to current_bid ; } } return ; }
/** Function to get ask which brings maximum
improvement **/
get_opt_ask(bid_quantity,bid_size) {
while ( there is unassigned ask) { call
get_next_unassigned_ask(asks,bid) ;
if ( ask_quantity >= bid_quantity and ask_size >=
bid_size) then qty_asg = bid_quantity end if ;
if ( bid_quantity < ask _quantity ) then qty_asg =
qty_asg_+ bid_quantity ; mark ask temp_assigned ;
call cal_obv(bid,ask,qty_asg) ;
if ( ov > max_imp ) then { ask_ret = current_ask ;
max_imp = ov ; } return ; }
/** This function gets next lowest price ask **/
get_next_unassigned _ask(asks,bid) {
while ( there is unassigned and unmarked ask } {
if ( (bid_price < min_price) and can be assigned to bid )
then { set min_price to ask_price;
set ask to current_ask; } } return ; }
/ ** This function calculates the contribution **/
cal_obv(bid,ask,qty_asg) { net_surplus = ( bid_price –
ask_prce ) * bid_quantity ;
wastage = ( ask_size – bid_size ) * bid_quantity ; call
search_table(wastage,table_size); if found then set
wastage = 0 ; ov = net_surplus + wastage ; return }
/** This function does the assignment **/
assignment(bid,ask,qty_asg) {
assign ask to bid ; quantity_assigned = qty_asg ;
if ( qty_asg = bid_quantity ) then mark bid as assigned ;
if ( qty_asg = ask_quantity and ( ask_size – bid_size = 0 )
) then mark ask as assigned ;
if ( qty_asg < bid_quantity ) then bid_quantity =
bid_quantity – qty_asg ;
if ( qty_asg < ask_quantity ) then ask_quantity =
ask_quantity – qty_asg ;
if ( ( ask_size – bid_size) > 0 ) then ask_size = ask_size-
bid_size; return ; }
Example: The working of the algorithm is
illustrated in the following example with five asks
and five bids. The assignment constraints are
basically size constraints i.e. a bid can be matched
with an ask of same or higher size. The wastage is 8
here. The output can be seen in Table 3.
DESIGN OF CONTINUOUS CALL MARKET WITH ASSIGNMENT CONSTRAINTS
185