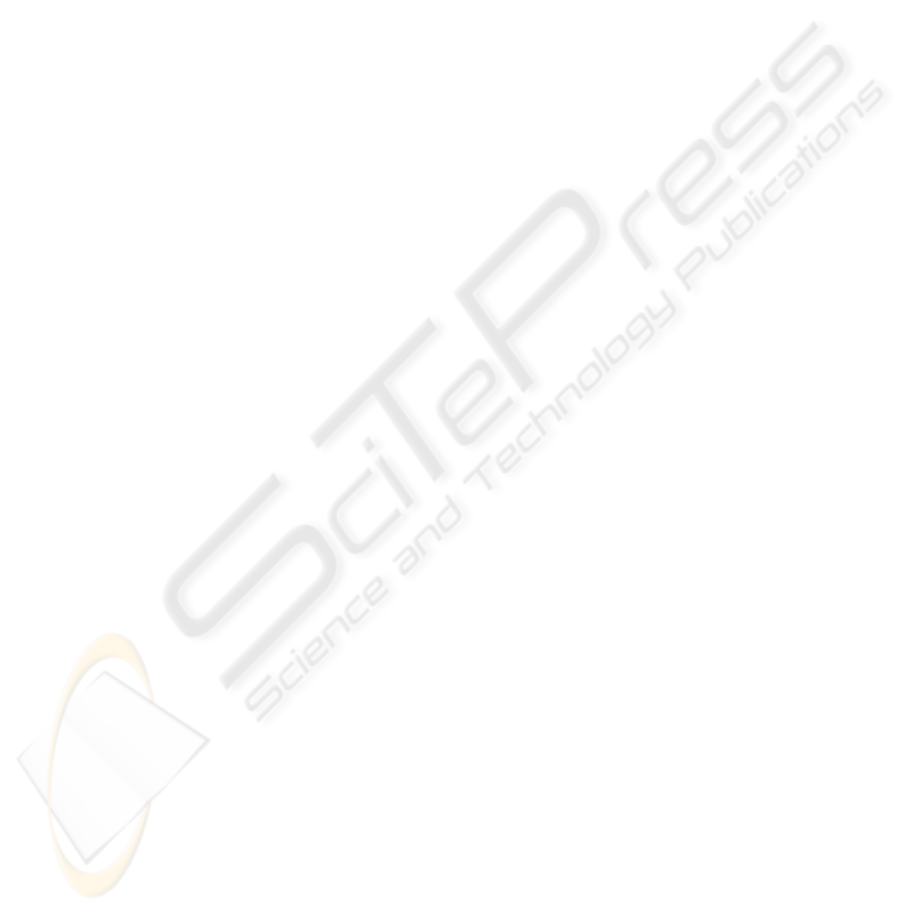
and tend to associate advantages and drawbacks of
tries with those of DSTs. For instance, at the time of
writing of this paper, Wikipedia does not even list the
DSTs as one of the tree data structures, while many
other structures, including AVL trees, AA trees, splay
trees, tries, etc. are listed (Wikipedia, The Free Ency-
clopedia, 2007).
The second reason for low popularity of DSTs is
that they are sensitive to bit distribution. Namely,
these trees will be skewed if the probability of occur-
rence of 0 and 1 digits is not equal (Knuth, 1997).
In this paper we show that although this is true, the
worst case performance is still satisfactory, primarily
because of the effects the cache hierarchy has on the
performance of modern computers.
The third reason is associated with limited use of
DSTs. At first, it was thought that these trees can
only be used when all the keys in the tree have equal
length, or with variable length keys when no key is a
prefix of another. Later, a simple method for storing
arbitrary keys of variable lengths into DSTs was de-
vised (Nebel, 1996). Another limitation is due to the
fact that DSTs require access to individual bits of the
key, which is not easily achievable in some high-level
languages. This is a valid concern, but it should not
be a reason to prevent the use of these trees by the
programmers in languages, such as C, where bit-level
data manipulation is not an uncommon task.
Believing that their advantages may outweigh
their disadvantages, in this paper we experimentally
evaluate the performance of DSTs and compare their
performance with alternate approaches. The rest of
the paper is organized as follows. The next sec-
tion gives a description of DSTs and associated algo-
rithms. Experimental evaluation is presented in sec-
tion 3, and conclusion in section 4.
2 DST ALGORITHMS
A digital search tree (DST) is a binary tree whose or-
dering of nodes is based on the values of bits in the
binary representation of a node’s key (Knuth, 1997).
The ordering principle is very simple: at each level
of the tree a different bit of the key is checked; if the
bit is 0, the search continues down the left subtree, if
it is 1, the search continues down the right subtree.
The search terminates when the corresponding link in
the tree is NIL. Every node in a DST holds a key and
links to the left and right child, just like in an ordinary
binary search tree. In contrast, a trie does not store
keys in internal nodes, only in leaves.
Let us define some terms that are used in this pa-
per. A node x has the height h
x
, which is equal to
the number of edges on the longest downward path
from the node to a leaf. The height of the tree, h, is
equal to the height of its root. A node x has the depth
d
x
, which is equal to the number of edges on the path
from the node to the root. The depth of the tree, d, is
equal to the depth of the leaf with the greatest depth,
and is equal to the tree height, h.
In the following sections we only give intuitive
descriptions of algorithms for DSTs. Exact algo-
rithms can be found in (Knuth, 1997) and (Sedgewick,
1990).
2.1 Creating and Searching Dsts
Let us build a tree with the following elements: 1001,
0110, 0000, 1111, 0100, 0101, 1110. Since we start
with an empty tree, the first element, 1001, becomes
the root of the tree. The next key to be inserted is
0110. First, we have to check whether this key is
equal to the key of the root node. Since it is not, we
continue traversal down the tree. To decide where to
place the node, we look at the first bit from the left.
Since this bit is 0, we take the path down the left sub-
tree and find that the left pointer is NIL. Therefore, we
create a new node, which is the left child of the root
node, as shown in Figure 1a. The next element to be
inserted is 0000. Since the root of the tree is not NIL
(and its key is not equal to the key being inserted),
we look at the first bit (from the left) of the key to be
inserted. Since this bit is 0, we take the path down
the left subtree. Since the next node is not NIL either,
we look at the next (second) bit of the key to be in-
serted. The bit is 0, so we take the path down the left
subtree. At this point we encounter a NIL pointer and
place the new node in its place, as depicted in Figure
1b. We continue the procedure until all nodes have
been added, and the tree in Figure 1f is obtained. Bits
used to make the decisions while progressing down
the tree are shown in bold in the figure. It is worth
noting that scanning the bits from left to right, when
making search decisions, is an arbitrary choice. The
tree can be built in the same manner by scanning the
bits from right to left.
The insertion method for DSTs is nearly identical
to the insertion method for binary search trees. We
first compare the key to be inserted with the key in
the current node, except that for DSTs we test only for
equality. In addition, DST algorithms test the corre-
sponding bit of the new key to choose the next move.
To search for an element, we traverse the tree in
the same way the insert procedure does. If along the
way we find that the current node’s key matches the
key we are looking for, the search completes success-
fully. If during the traversal we reach a NIL node, this
ICSOFT 2007 - International Conference on Software and Data Technologies
62