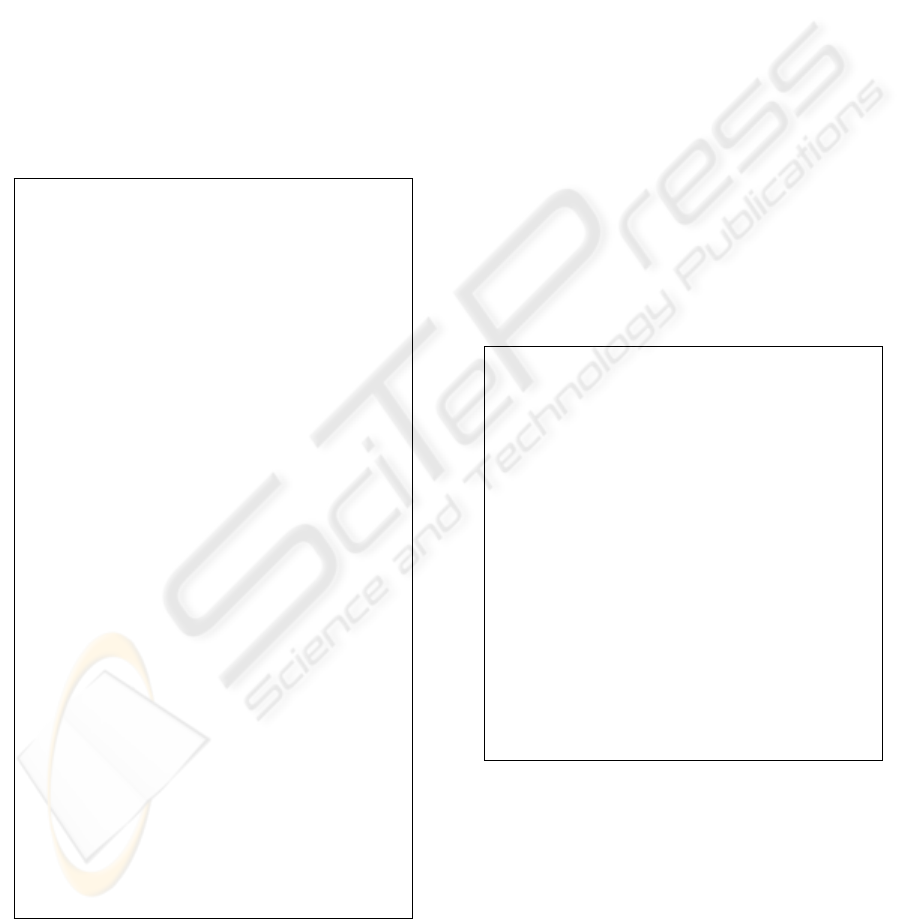
same two structures described in the previous algo-
rithm (whiteList and grayList), and also a list that hold
gray nodes with available bandwidth to support other
children (almostBlackList).
The algorithm starts inserting the source node in
the grayList, initializing all other nodes with it dis-
tance from the source dsrc as infinity and adding
those in the whiteList. It proceeds by getting (and
removing) the closest node X to the source from the
grayList. This node is inserted in the almostBlack-
List. Then, for each node in the grayList and whiteList
(let’s merge these two lists in one whiteAndGrayList)
it is calculated the new distance from the source ele-
ment through each element in almostBlackList. This
is the “relax” process of the Dijkstras algorithm. All
visited nodes are inserted (or updated) in the grayList.
Listing 2: BPA Pseudo Code.
1 BPA( ALLNodes , s rc No de )
2 f o r e a c h n o d e n i n ALLNodes :
3 d s r c [ n ] : = i n f i n i t y
4 c o l o r [ n ] : = WHITE
5 end f o r
6 c o l o r [ s r c N o d e ] : = GRAY
7 d s r c [ src No de ] : = 0
8 i n s e r t ( g r a y L i s t , sr c N o d e )
9 w h i l e ( g r a y L i s t != 0 )
10 u : = e x t r a c t −min−d s r c ( g r a y L i s t )
11 al m o s t B l a c k : = a l m o s t B l a c k U {u }
12 p : = p a r e n t [ u ]
13 i f p i s n o t u :
14 N[ p ] : = N[ p ] − 1
15 i f N[ p ] = 0 :
16 rem o v e [ a l m o s t B l a c k , p ]
17 c o l o r [ p ] : = BLACK
18 fo r e ac h node v i n w h i t e A n d G r a y L i s t :
19 i f p a r e n t [ v ] = p :
20 f o r e a c h n o d e b i n a l m o s t B l a c k :
21 RELAX( b , v )
22 end f o r
23 end f o r
24 e l s e
25 f o r e a ch node v i n wh i t e A n d G r a y L i s t :
26 RELAX( u , v )
27 en d f o r
28 end w h i l e
29
30 RELAX( u , v )
31 i f ( d i s t a n c e O f ( u , v ) + d s r c [ u ] < d s r c [ v ] )
32 d s r c [ v ] : = d i s t a n c e O f ( u , v ) + d s r c [ u ]
33 p a r e n t [ v ] : = u
34 i f ( c o l o r [ v ] = WHITE)
35 c o l o r [ v ] : = GRAY
36 i n s e r t ( g r a y L i s t , v )
37 e l s e
38 u p d a t e ( g r a y l i s t , v )
When one node in the almostBlackList uses all N
connections (do not support more children), then it
is removed from that list and all nodes in the white-
AndGrayList must update its dsrc information (relax
process). We check this every time a parent is as-
signed to a node. The operation is repeated until the
grayList is empty. This algorithm is described in the
following pseudo code.
2.3.3 Fast Bandwidth and Position Aware Tree -
FBPA
This algorithm is based on the first algorithm pre-
sented, but using the “relax” process of the previous
algorithm. Therefore, it uses two data structures, the
whiteList and grayList.
As the previous algorithms, this one starts insert-
ing the source node in the grayList, initializing all
other nodes with it distance from the source dsrc as
infinity and adding those in the whiteList. It pro-
ceeds by extracting the closest node X to the source
node from the grayList. Node X share bandwidth to
provide other N nodes as it children. For all nodes
in the whiteList it is calculated the distance from X.
Then, the N closest nodes from X are inserted in the
grayList. Node X is set as a black node. The operation
is repeated until the grayList is empty. This algorithm
is described in the following pseudo code.
Listing 3: FBPA Pseudo Code.
1 FBPA ( AllN o des , sr cN od e )
2 f o r e a c h n o d e n i n Al lN od es :
3 c o l o r [ n ] : = WHITE
4 d s r c [ n ] : = i n f i n i t y
5 end f o r
6 c o l o r [ s r c N o d e ] : = GRAY
7 d s r c [ src No de ] : = 0
8 p u sh ( g r a y L i s t , sr c N o d e )
9 w h i l e ( g r a y L i s t != 0 )
10 u : = p u l l F i r s t ( g r a y L i s t )
11 fo r e ac h node v i n w h i t e L i s t :
12 d s r c [ v ] : = d i s t a n c e O f ( u , v ) + d s r c [ u ]
13 end f o r
14 fo r i = 1 t o N[ u ] :
15 n : = e x t r a c t −min−d s r c ( w h i t e L i s t )
16 c o l o r [ n ] : = GRAY
17 p a r e n t [ n ] : = u
18 pu s h ( g r a y L i s t , n )
19 end f o r
20 c o l o r [ u ] : = BLACK
21 end w h i l e
3 PRELIMINARY ANALYSIS
In this section we analyze the three building algo-
rithms, comparing the mean distance from the source
node against the elapsed time during the calculation
of the tree. The nodes were inserted in a network
topology and its coordinates were normalized to be
in a Cartesian plan between (0,0) and (1000,1000).
Also, the nodes could share their bandwidth with
A LOCATION AND BANDWITDH AWARE P2P VIDEO ON DEMAND SYSTEM FOR MOBILE DEVICES
251