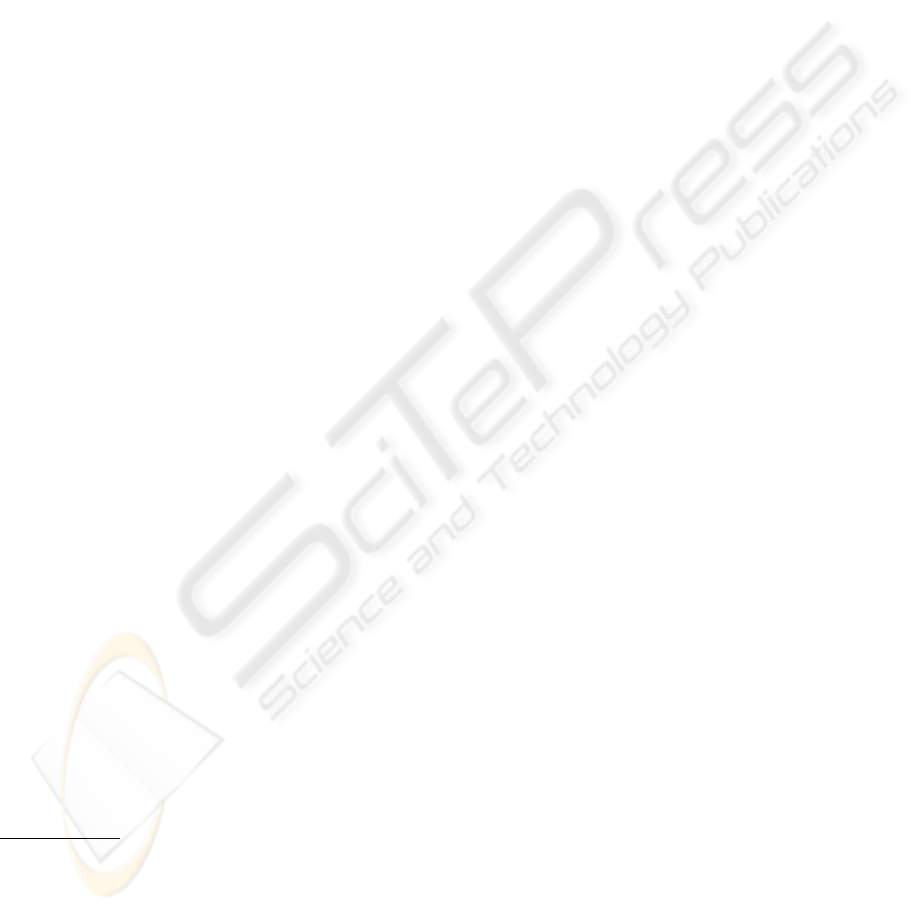
tivities
3
. Therefore, any re-use of the model that is
independent of a platform or performance constraints
can lower the cost of maintenance. Many UML state-
ments can be implemented in more than one way.
The semantics of UML associations only specifies
the relations that the system should maintain. The de-
tails of how to access, traverse, and modify the re-
lation are left for the implementer. All of the works
mentioned above focus on providing a single imple-
mentation to associations, based on standard collec-
tions frameworks — For example, see the Java collec-
tions tutorial (Bloch, 1995). This approach provides
the richer semantics of associations in code, but does
not allow for replacing the implementation without
re-writing the code. For example, most standard col-
lections frameworks provide only sequential traver-
sal over an entire collection. This limitation neither
appears nor is implied by the UML standard
4
. We
would like to allow the developer to choose between
sequential and parallel traversal by changing a prop-
erty of the association, without re-writing the code.
Fast access to elements in a collection with specific
values requires in most frameworks adding a support-
ing data structure explicitly (E.g., a hash-table that
maps from integers to persons in a collection that are
of a given age). We would like to allow the developers
to add such indices as a property of the association, or
even let a smart code-generator decides which indices
should be used.
In this work we present flexible code generation
scheme that allows for replacing the generated imple-
mentation of associations without requiring changes
to the rest of the code. Section 2 describes the in-
terfaces for accessing, updating, and traversing over
associations. Section 3 describes different schemes
for generating implementations for those interfaces.
Section 4 provides an example of implementing and
using this scheme to improve the implementation of
associated classes. Section 5 concludes.
2 INTERFACES
To replace the implementation of associations in-
dependently of the client code
5
, we must provide
3
Activities that adapt the software to new platforms
4
Class diagram specifications do not discuss any oper-
ations over associations. The relevant sequence diagrams
specifications, such as for loops and multiple instances, also
omit these details. See the UML reference manual (Rum-
baugh et al., 2004).
5
“Client code” here refers to the code that uses the asso-
ciations, as opposed to the code that implements the associ-
ations
uniform interfaces for operations over associations.
These operations include:
1. Adding and removing pairs of associated in-
stances from the relation.
2. Traversing over the instances associated with a
given object.
We ignore the case of changing the set of instances
that are associated with a given object, since it can
be implemented by adding and removing pairs from
the relation. Operating on pairs instead of unrelated
collections allows the implementation to enforce bi-
directionality and multiplicity constraints. This ap-
proach is common to many of the works mentioned
above.
The second kind of operations, traversing associ-
ated instances, requires special attention. Most work
on associations propose to use a variation of the Iter-
ator pattern (Gamma et al., 1995). This pattern pro-
vides a way to perform a sequential traversal over a
data structure without exposing its implementation.
This approach, however, has several drawbacks:
1. It forces the traversal to be sequential and does
not enable parallel traversal, even when there are
no data dependencies between the iterations.
2. It does not encapsulate the selection of elements.
Therefore, optimizations like using hash-tables or
caching must be a part of the client code.
3. Iterators, together with loop commands (for,
while, etc.) often serve for implementing oper-
ations over a range of elements. The body of the
loop represents an operation over a single element
and is dependent on its internal structure. The iter-
ator pattern encapsulates the traversal, but exposes
the structure of the traversed elements. Moving
this implicit operation to a method in the class of
the element and explicitly applying this method
on all associated instances would provide better
encapsulation.
To overcome these drawbacks, we propose to use
different set of interfaces, similar to the one outlined
in (Martin and Odell, 1992):
• The Association interface represents the relation
between two types.
• The AssocEnd interface represents the collection
of instances that are associated with a given ob-
ject.
• The Foreach, Map, Filter, and Fold interfaces rep-
resent operations over a collection of instances.
They are called aggregator interfaces.
The aggregator interfaces are generated and in-
clude sub-sets of the messages from the associated
GENERATING FLEXIBLE CODE FOR ASSOCIATIONS
97