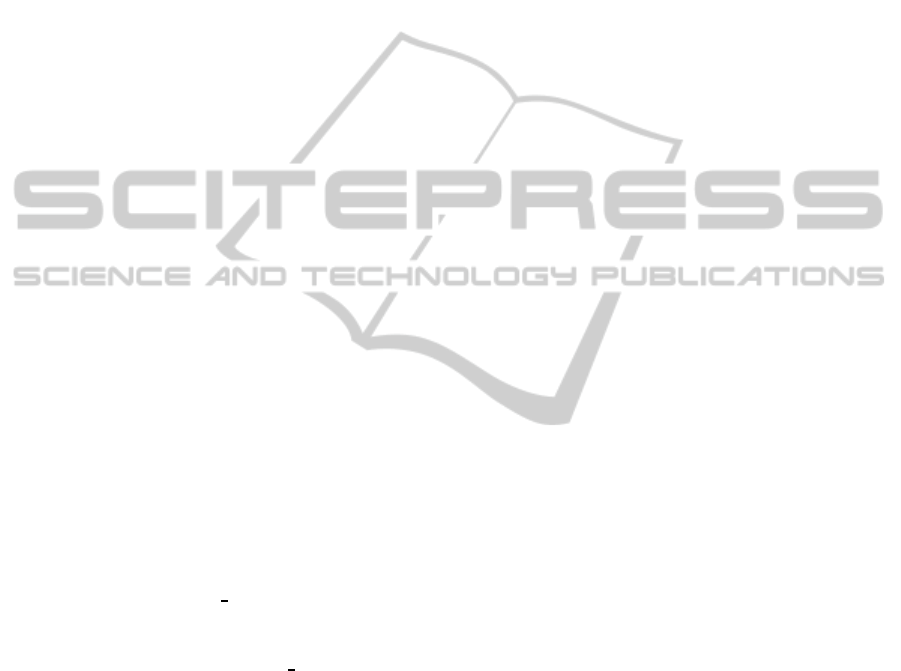
check if
x
is (only) in the domain of the simplicial
fan. If it is we go through all simplices in the fan to
find a simplex containing
x
. If
x
is in the domain of
the simplicial complex, but not in the fan, we use the
efficient strategy of computing a simplex containing
it as described above. The code has, obviously, to
be adapted to the fact that Armadillo indexes vectors
from zero. The implementation is as follows:
int T_std_NK::InSimpNr(vec x){
if(!(min(Np-x)>0 && min(x-Nm)>0)){
return -1;
}
if(min(Kp-x)>0 && min(x-Km)>0){
for(int i=0;i<Fan.size();i++){
if(InSimp(x,Fan[i])){
return Fan[i];
}
}
}
// WE CAN COMPUTE THE POSITION OF THE SIMPLEX
int J=0;
ivec z(n),sig; // sig=sigma
for(int i=0;i<n;i++){
if(x(i)<0){
x(i)=-x(i);
J |= 1<<i;
}
z(i)=static_cast<int>(x(i));
}
sig=conv_to<ivec>::from(sort_index(x-z,1));
return equal_range(NrInSim.begin(),
NrInSim.end(),zJs(z,J,sig)).first->Pos;
}
We havea fewcomments on this implementation. The
command
J |= 1<<i;
sets the (i+ 1)-th bit of the bi-
nary representation of J equal to 1. Because the enti-
ties of
x
are all nonnegativewhen we want to compute
their floor, we can simply cast from
double
to
int
.
The Armadillo function
sortindex
returns a vector
of unsigned integers that describes the sorted order
of the given vector’s elements. The optional second
parameter can be set to 1 to let
sortindex
use de-
scending sort, otherwise it uses the default, which is
ascending sort.
7 SUMMARY
We described the implementation of a simplicial com-
plex with a simplicial fan at the origin. Such com-
plexes allow for the parameterizations of continuous,
piecewise affine (CPA) functions, with an arbitrary
rich structure at a singularity. Such CPA functions
have been shown to be irreplaceable in the computa-
tion of true CPA Lyapunov functions for general non-
linear systems. We used C++11 and the Armadillo
linear algebra library for the implementation and we
discussed some of the advantages of doing so in the
paper. Thus, the paper might be of interest to sci-
entists and engineers interested in modern numerical
programming in C++11 under Windows, even if they
are not necessarily interested in our particular prob-
lem of implementing simplicial complexes for CPA
functions.
REFERENCES
Eghbal, N., Pariz, N., and Karimpour, A. (2012). Discon-
tinuous piecewise quadratic Lyapunov functions for
planar piecewise affine systems. J. Math. Anal. Appl.,
399, pp. 586–593.
Giesl, P. (2007). Construction of Global Lyapunov Func-
tions Using Radial Basis Functions. Lecture Notes in
Mathematics, 1904, Springer.
Giesl, P. and Hafstein, S. (2012). Existence of piecewise lin-
ear Lyapunov functions in arbitary dimensions, Dis-
crete Contin. Dyn. Syst., 32, pp. 3539–3565.
Giesl, P. and Hafstein, S. (2013). Revised CPA method to
compute Lyapunov functions for nonlinear systems.
(submitted)
Hafstein, S. (2004). A constructive converse Lyapunov the-
orem on exponential stability. Discrete Contin. Dyn.
Syst., 10, pp. 657–678.
Hafstein, S. (2005). A constructive converse Lyapunov
theorem on asymptotic stability for nonlinear au-
tonomous ordinary differential equations. Dynamical
Systems, 20, pp. 281–299
Johansen, T. (2000). Computation of Lyapunov Functions
for Smooth Nonlinear Systems using Convex Opti-
mization. Automatica, 36, pp. 1617–1626.
Johansson, M. and Rantzer, A. (1997). On the computa-
tion of piecewise quadratic Lyapunov functions. In:
Proceedings of the 36th IEEE Conference on Decision
and Control.
Julian, P., Guivant, J., and Desages, A. (1999). A
parametrization of piecewise linear Lyapunov func-
tion via linear programming Int. Journal of Control,
72, pp. 702–715.
Marinosson, S. (2002a). Lyapunov function construction
for ordinary differential equations with linear pro-
gramming. Dynamical Systems, 17, pp. 137–150.
Marinosson, S. (2002b). Stability analysis of nonlinear sys-
tems with linear programming: A Lyapunov functions
based approach. Ph.D. Thesis: Gerhard-Mercator-
University, Duisburg, Germany.
Peet, M. and Papachristodoulou, A. (2010). A converse
sum-of-squares Lyapunov result: An existence proof
based on the Picard iteration. In: 49th IEEE Confer-
ence on Decision and Control, pp. 5949–5954.
Rezaiee-Pajand, M. and Moghaddasie, B. (2012). Estimat-
ing the Region of Attraction via collocation for au-
tonomous nonlinear systems. Struct. Eng. Mech., 41-
2, pp. 263–284.
Sanderson, C. (2010). Armadillo: An Open Source C++
Linear Algebra Library for Fast Prototyping and
Computationally Intensive Experiments. Technical
Report, NICTA.
ImplementationofSimplicialComplexesforCPAFunctionsinC++11usingtheArmadilloLinearAlgebraLibrary
57