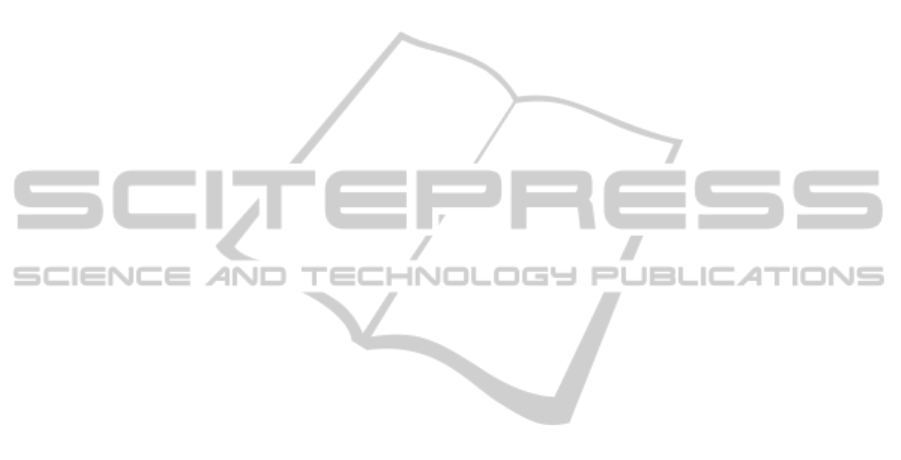
average of the surrounding four cells is taken, with
their weight dependent on their proximity to the
origin location.
In order to model the advection step is in Cell-
DEVS we are required to include any of the cells
where the density can originate, in the neighborhood.
The first and most important part is ensuring that the
possible source cells are included within the neigh-
borhood. The neighborhood of the advection step is
defined 6 by 6 cells therefore; the maximum distance
a particle can travel is 2 cells from the center. Hence,
the velocity vectors cannot exceed the range of (-
2,2). This can be done by scaling the time step to en-
sure that the velocities remain within the acceptable
limits. For example, if the velocity is 4, it can be
scaled down to 2 and the new time step would be half
of the original. The values of the cells are truncated
to discrete values therefore, there are 5 potential val-
ues: -2,-1,0,1 and 2, for u and v and therefore 25 dif-
ferent combinations of the two. For each combina-
tion, the ratio of the four source cells is calculated.
The following is a portion of the code that deter-
mines the value if the truncated values of the u and v
velocities are 1 and1, respectively.
if( trunc((0,0,-6)) = 1,
if( trunc((0,0,-5)) = 1,
(((1-remainder(abs((0,0,-6)),1))
*((1-remainder(abs((0,0,-5)),1))
*(-1,- 1,-2)+ remainder(
abs((0,0,-5)),1)*(-1,0,-2))+
remainder(abs((0,0,-6)),1)* ((1-
remainder(abs((0,0,-5)),1)) *(0,-1,-
2)+remainder(
abs((0,0,5)),1)*(0,0,-2) )
)
) * this is 1 of 24 possibilities
In this code, it is checking to see if the u and v
vectors fall within the range of 1.0 to 1.999. If this is
the case than by the weighted averages are calculated
and summed.
The code contains 25 iterations of the above code
segment to cover the possible outcomes. This func-
tion is used 3 times in each cycle; the advection of
the density, the advection of u and the advection of v.
However, since the offsets of the required planes are
the same for both u and v (the offset is 0), the func-
tion can be recycled to solve for both. The advection
of the density step, however, requires access to a dif-
ferent plane with a different offset (2) and therefore
must be rewritten with its corresponding neighbor
values.
4.3 Projection
The projection step can be broken into three sub
sections: solving for div, p, u, and v. The original al-
gorithm is implemented using the following code:
void project ( int N, float *u,
float *v, float *p, float *div) {
int i, j, k; float h;
h = 1.0/N;
for ( i=1 ; i<=N ; i++ ) {
for ( j=1 ; j<=N ; j++ ) {
div[IX(i,j)] = -0.5*h*
(u[IX(i+1,j)]- u[IX(i-1,j)]+
v[IX(i,j+1)]-v[IX(i,j-1)]);
p[IX(i,j)] = 0; }
}
set_bnd(N,0,div); set_bnd(N,0,p);
for ( k=0 ; k<20 ; k++ ) {
for ( i=1 ; i<=N ; i++ ) {
for ( j=1 ; j<=N ; j++ ) {
p[IX(i,j)] = (div[IX(i,j)]+
p[IX(i-1,j)]+p[IX(i+1,j)]+
p[IX(i,j-1)]+p[IX(i,j+1)])/4;}
}
set_bnd ( N, 0, p );
}
for ( i=1 ; i<=N ; i++ ) {
for ( j=1 ; j<=N ; j++ ) {
u[IX(i,j)] -= 0.5*(p[IX
(i+1,j)]-p[IX(i-1,j)])/h;
v[IX(i,j)] -= 0.5*(p[IX(i,
j+1)]- p[IX(i,j-1)])/h; }
}
set_bnd(N,1,u); set_bnd ( N, 2, v );
} // (Stam 2003)
The implementation of Div is straightforward. It
takes the two u and two v values from their respec-
tive temp layers and is implemented with the follow-
ing code snippet in CD++ Model file:
rule : { if( remainder( time, 20 ) = 0,
-0.05*((1,0,-4)-(-1,0,-4)+(0,1,-3)
- (0,-1,-3) ), (0,0,0) ) } 1 {t}
As can be seen, the “if” function will work as a
loop that is reset after each iteration. The calculations
are essentially the same with the only difference on
where and how the information is accessed. The -4 at
the end of each neighbor cell means those values are
taken from the temporary layer for the u vectors
while the cells with -3 are taken from the temporary v
vectors.
To solve for p, we use the same method as solv-
ing for the diffusion. The code segment is exactly the
same as mentioned before, however the values of a
are adjusted to reflect the viscosity instead of the dif-
fusion coefficient.
The final step for the projection is the separating
of the vector fields into component form. The sepa-
rating of the horizontal and vertical components in
ComputationalFluidDynamicSolverbasedonCellularDiscrete-EventSimulation
221