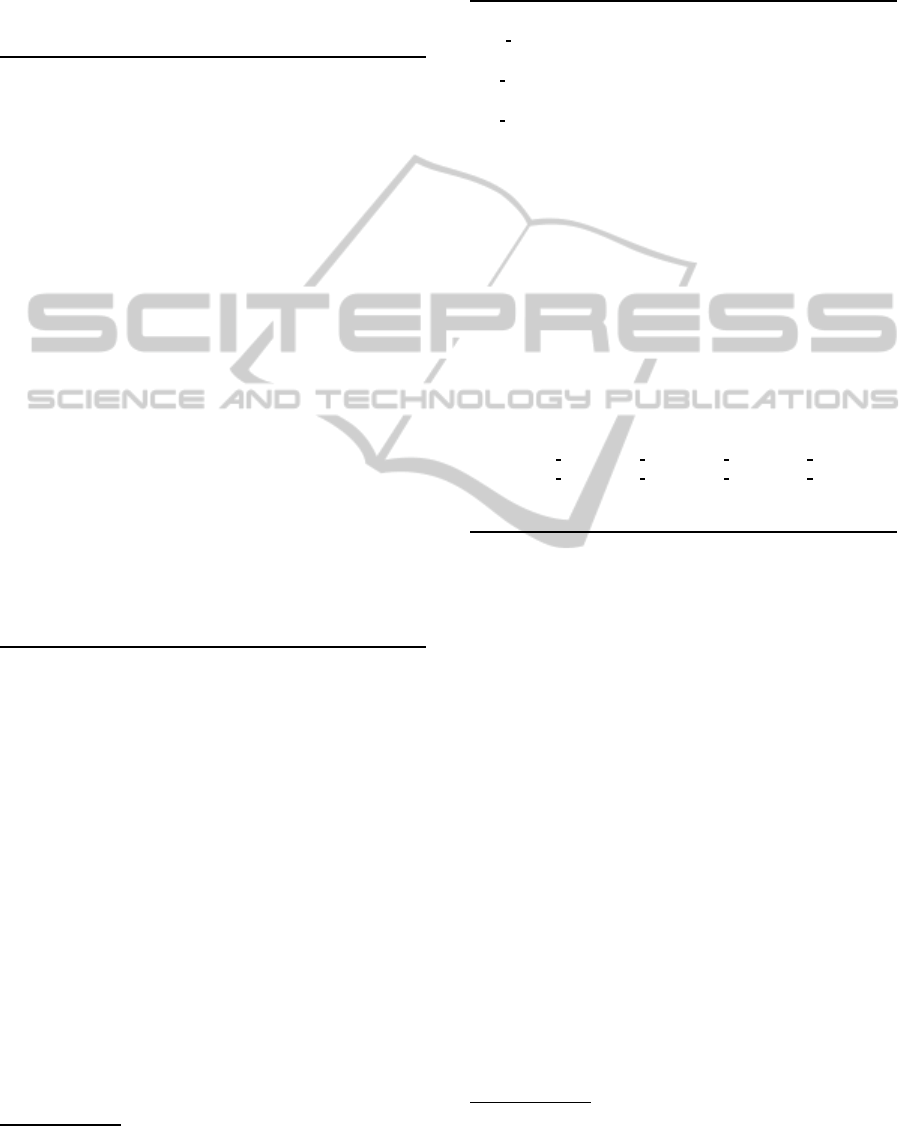
operations performed in the individual functions dur-
ing a round are either table lookups, shifts or xors.
The main loops are given in Listing 2. The code was
taken from the reference implementation by Soeren S.
Thomsen and Krystian Matusiewicz
2
.
Listing 2: Grøstl Kernel.
c a ll Gro e s t l Process . P( ctx , temp1 ) ; /∗ P( h+m) ∗/
c a ll Gro e s t l Process .Q( ctx , temp2 ) ; /∗ Q(m) ∗/
/∗ apply P−per mutation to x ∗/
void P( h a s h S tate ∗ctx , u8 x [ROWS][ COLS1024] ) {
u8 i ;
Vari a n t v = P512 ;
for ( i = 0; i < ctx−>rounds ; i ++) {
AddRoundConstant( x , ctx−>columns , i , v ) ; / / xor s
and s h i f t s
SubBytes (x , ctx−>columns ) ; / / t a b l e look ups
Shi f t B ytes (x , ctx−>columns , v ) ; / / s h i f t s
MixBytes ( x , ctx−>columns ) ; / / x ors and s h i f t s
}
}
/∗ apply Q−p ermut at ion to x ∗/
void Q( h a s h S tate ∗ctx , u8 x [ROWS][ COLS1024] ) {
u8 i ;
Vari a n t v = Q512 ;
for ( i = 0; i < ctx−>rounds ; i ++) {
AddRoundConstant( x , ctx−>columns , i , v ) ; / / xor s
and s h i f t s
SubBytes (x , ctx−>columns ) ; / / t a b l e look ups
Shi f t B ytes (x , ctx−>columns , v ) ; / / s h i f t s
MixBytes ( x , ctx−>columns ) ; / / x ors and s h i f t s
}
}
2.3 Tiger
The Tiger hash function (Anderson and Biham, 1996)
is, while already quite old, an interesting case for
our study, since it heavily relies on arithmetic on
64-bit words. Several weaknesses in the algorithm
have already been discovered (Mendel and Rijmen,
2007; Wang and Sasaki, 2010), yet currently there
is no feasible attack against a full round version of
Tiger known. Tiger also operates on 64-byte message
blocks, using three passes with eight rounds each, and
producing a 192-bit digest. At its core it makes heavy
use of lookups into four tables, each consisting of
256 64-bit words, thereby requiring (4∗256∗64)/8=
8,192 bytes only for storing the tables. In this work
we were particularly interested if it was possible to
port an algorithm like this (using 64-bit arithmetic
and quite a lot of memory) to our constrained environ-
ments, how much effort would be required to perform
2
http://www.groestl.info/implementations.html
the port, and how fast the result would be. Listing 3
gives the main kernel of the hash function, taken from
the reference code
3
.
Listing 3: Tiger Kernel.
sa ve abc () ; / / only assign ments
pa ss ( a , b , c , 5 ) ; / / tab l e loo kups and xor s
ke y
sc hedule ( ) ; / / x ors and s u b t r a ct i o n s
pa ss ( c , a , b , 7 ) ; / / tab l e loo kups and xor s
ke y
sc hedule ( ) ; / / x ors and s u b t r a ct i o n s
pa ss (b , c , a , 9 ) ; / / ta b l e l ookups and xor s
feedforward ( ) ; / / x ors and s u b t r a c t i o n s
void pass ( a , b , c , mul ){
round ( a , b , c , x0 , mul ) ;
round ( b , c , a , x1 , mul ) ;
round ( c , a , b , x2 , mul ) ;
round ( a , b , c , x3 , mul ) ;
round ( b , c , a , x4 , mul ) ;
round ( c , a , b , x5 , mul ) ;
round ( a , b , c , x6 , mul ) ;
round ( b , c , a , x7 , mul ) ;
}
void round ( a , b , c , x , mul ) {
c ˆ= x ;
a −= t1 [ c
0 ] ˆ t2 [ c 2 ] ˆ t3 [ c 4 ] ˆ t4 [ c 6 ] ;
b += t4 [ c
1 ] ˆ t3 [ c 3 ] ˆ t2 [ c 5 ] ˆ t1 [ c 7 ] ;
b ∗= mul ;
}
3 IMPLEMENTATION AND
PRELIMINARY RESULTS
We implemented the three algorithms detailed in Sec-
tion 2 on Memsic Iris and MicaZ motes
4
. Both are
equipped with an 8-bit Atmel AVR ATmega128 mi-
croprocessor (the 1281 version in the case of Iris, the
128L variant for the MicaZ), clocked at 8 MHz, with
the Iris motes able to utilize up to 8KB of RAM,
whereas the MicaZ motes are limited to only 4KB.
Both motes are provided with 128KB of Flash ROM,
in addition to 512KB of measurement ROM to hold
sampled values (which was not used for our evalua-
tion).
In practice, there was next to no runtime differ-
ence between the two nodes (which was to be ex-
pected, since they share a common processor archi-
tecture), the only implementation difference emerged
in terms of available RAM; in the Tiger implementa-
tion for the MicaZ node we had to move six of the
lookup tables from RAM to ROM in order to get be-
3
http://www.cs.technion.ac.il/∼biham/Reports/Tiger/
4
http://www.memsic.com/wireless-sensor-networks/
DCNET2013-InternationalConferenceonDataCommunicationNetworking
66