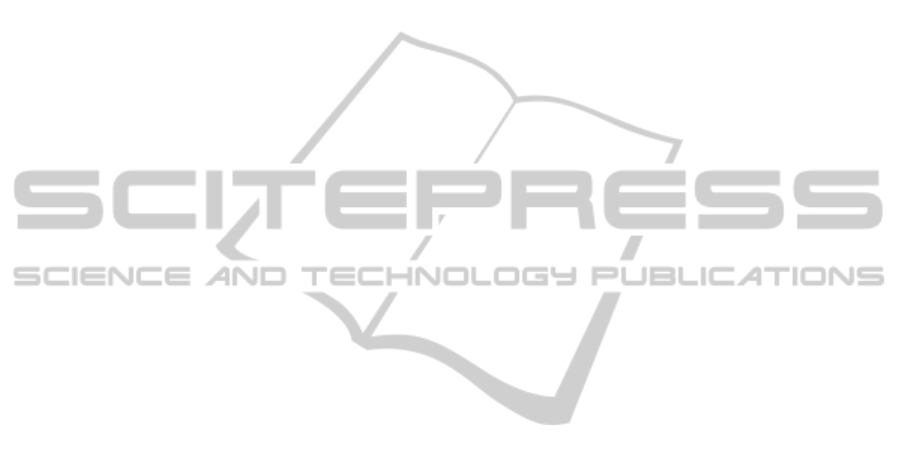
PHP itself has XML parser called SimpleXML
and the source code for processing elements in the
file is following:
$source = simplexml_load_file
('export_1m.xml');
$items = $source->xpath
("/root/item");
foreach ($items as $item) {
$array[$i] = (string)$item;
$i++;
}
We are saving every line into array just to be sure
that this line is processed. Script for parsing data
from
param attribute looks very similar:
$source = simplexml_load_file
('export_1m.xml');
$items = $source->xpath
("/root/item");
foreach ($items as $item) {
$array[$i] = (string)$item['param'];
$i++;
}
Start time and end time of application is tracked
with function
microtime(), which is called before
loading XML file and after loading the last node in
XML file.
3.2 Application in Java
According to TIOBE index, this programming
language was the most popular world-wide until
year 2012. Java uses 256 megabytes of memory in
default, which is sufficient for only around 150
thousands records. Therefore it is also required to
enlarge memory limit up to 1024 megabytes to be
limitless in our testing using Xmx parameter.
Java has its default XML parser called XPath API
which is part of basic Java package since version 5,
but thanks to its popularity, wide variety of libraries
extending and improving work with XML files is
available all over community forums.
Source code of application processing elements is
following:
XPath xpath =
XPathFactory.newInstance().newXPath();
NodeList nodes = (NodeList)
xpath.evaluate("/root/item/text()";,
new InputSource('export_1m.xml'),
XPathConstants.NODESET);
int size = nodes.getLength();
String[] valueArr = new String[size];
for (int i = 0; i < size; i++) {
valueArr[i] =
nodes.item(i).getNodeValue();
}
And with slight modification we get source code
of application which parses attributes of given XML
file:
XPath xpath =
XPathFactory.newInstance().newXPath();
NodeList nodes = (NodeList)
xpath.evaluate("/root/item/@param", new
InputSource('export_1m.xml'),
XPathConstants.NODESET);
int size = nodes.getLength();
String[] valueArr = new String[size];
for (int i = 0; i < size; i++) {
valueArr[i] =
nodes.item(i).getNodeValue();
}
Duration of running application is in this case
monitored using function nanoTime() which is again
called twice, once before file is loaded and nodes
parsed and once after whole process is finished.
3.3 Application in Visual Basic
The youngest of all used languages is Visual Basic
developed by Microsoft Company. According to the
TIOBE index this language is losing its popularity
since year 2010.
Unlike the two already mentioned languages, this
one does not have any memory limitation in default,
so there is no need for initial settings modification.
Application used for parsing elements has following
source code:
xml.LoadXmlFile('export_1m.xml')
Dim item = xml.FirstChild()
While Not (item Is Nothing)
Dim value As String = item.Content
item = item.NextSibling()
End While
While again with slight modifications we get an
application which parses attributes from XML file.
xml.LoadXmlFile('export_1m.xml')
Dim item = xml.FirstChild()
While Not (item Is Nothing)
Dim value As String =
item.GetAttrValue("param")
item = item.NextSibling()
End While
KMIS2014-InternationalConferenceonKnowledgeManagementandInformationSharing
356