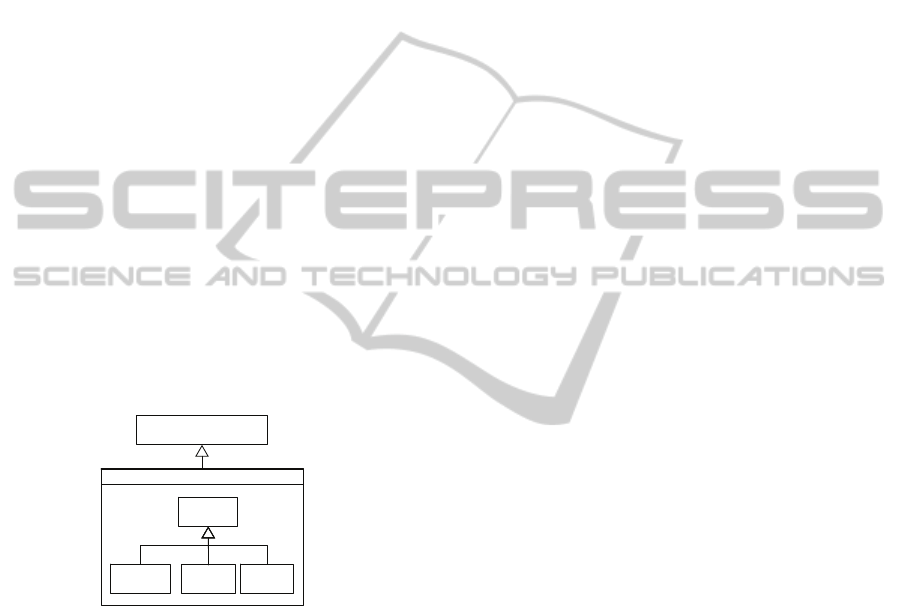
events, the states of a state machine are also
represented by virtual classes (see
On and Off in
Figure 11).
Figure 12 illustrates how the
MediaSwitch is
defined as a subclass of
Switch. The class State is
extended in order to implement the new event
method mode, the states
CD and TV are added as
subclass of
State, and On is extended in order to
become a composite state.
The fact that the state classes of a state machine
are virtual classes implies that the construction of
the state hierarchy may be inherited and does not
have to be made again for specialized state
machines. As an example, the constructor for
Switch in Figure 11 will have a statement that
generates an
On state object and sets the encloser to
be an object of class
SwitchState.
The
MediaSwitch state machine inherits this
constructor, and as
On has been extended, the
inherited generation statement will now generate an
object of the extended
On. In this respect a virtual
class works the same way as a virtual method: like a
call of virtual method implies a call of the
overridden method in case the call is made in the
context of a subclass, generation of an object of a
virtual class will imply generation of the extended
class.
Figure 12: MediaSwith as a specialization of Switch.
As part of extending a virtual state class, it is
possible to override inherited event methods. In
principle an event method may be completely
overridden, i.e. changing also the next state of the
transition, and that is not desirable. A simple
solution is to define the event methods as non-virtual
(final in Java) and then rather define for each event
method a corresponding virtual action method that is
called by the event method.
Java does not support virtual classes. While it is
straightforward to obtain delegation by means of a
design pattern, virtual classes are not that easy to do
by a design pattern. The framework in Java therefore
does not support the solution with virtual state
classes. This is also the reason that this subsection
just has illustrations of the solution, no code
fragments. Except for the part of the code that
expresses virtual classes and their extensions, the
rest of the code, e.g. for the handling of events, will
be the same as already described.
With virtual state classes there is no need for
generics as described above. The
cS would be typed
by
State, and along with extension of State in
subclasses of
StateMachine the type of cS is also
extended.
6 RELATED WORK
As described in the introduction, the original state
design pattern does not cover composite states.
Most modelling languages have full support for state
machines directly as language mechanisms. Existing
state machine APIs in various programming
languages also support full state machines, but
without any attempts to integrate the state machine
mechanisms with the mechanisms of language.
Among the approaches that are integrated with
existing language mechanisms, the Actor model
(Hewitt, Bishop et al. 1973) was the first approach.
Actors can change state explicitly and thereby
accepting a new set of messages. This idea has later
been followed by proposals where an object may
change its class and thereby the methods it will
accept. The Modes approach (Taivalsaari 1993) also
belongs to the well-integrated approaches, and it is
directed towards supporting state-oriented
programming in that an object does not have to
change its class, only its virtual method dispatch
pointer. The solution in (Madsen 1999) takes the
Modes approach a little further in that it supports
composite states by means of state class inheritance.
State-Oriented Programming (Sterkin 2008) is
very similar to our approach. It recognizes that states
have to be defined by objects that are linked to
represent state hierarchies, but does not use
delegation.
A quite different approach is taken by Typestate-
Oriented Programming (Aldrich, Sunshine et al.
2009; Sunshine, Naden et al. 2011) supported by the
Plaid language. It is in line with Modes and with our
approach in that state mechanisms are well
integrated in the language, however, it only supports
simple states. The reason is that the main objective
is to define a corresponding type system that will
make it possible to check that objects behave in
accordance to the constraints specified by state
types.
MediaSwitch
Switch
ext e nde d
On
ext ended
State
vi r t ual
CD
vi r t ual
TV
CombinedModellingandProgrammingSupportforCompositeStatesandExtensibleStateMachines
237